Create Performant Falling Particles With The Sparticles Library
File Size: | 1.53 MB |
---|---|
Views Total: | 3145 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
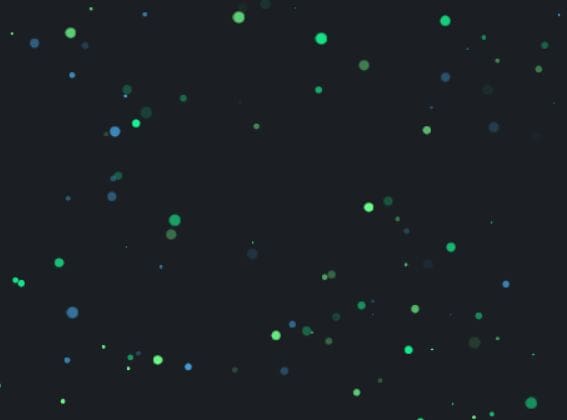
Sparticles.js is a lightweight and performant library for creating falling particles using JavaScript, requestAnimationFrame, and HTML5 canvas.
Compatible with Vanilla JavaScript, jQuery, Svelte.js and ESM. Ideal for creating falling sparkles, snowflakes, stars, and any shapes as the background of your webpage.
Features:
- Supports any type of shaples like circle, square, triangle, diamond, line, image, or random.
- Configurable min/max size, alpha, animation speed, and much more.
- Parallax and Rotate effects.
How to use it:
1. Download and import the Sparticles.js
library into your project.
<!-- Vanilla JS --> <script src="/path/to/dist/sparticles.js"></script> <!-- jQuery --> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/dist/sparticles.js"></script>
// OR As An ES Module import Sparticles from "/path/to/dist/sparticles.esm.js";
2. Initialize the Sparticles.js on the targer container where you want to render the particles.
// Vanilla JS (function() { window.onload = function() { var $main = document.querySelector("main"); window.mySparticles = new Sparticles($main); } }()); // jQuery $(function() { var $main = $("main"); window.mySparticles = new Sparticles($main.get(0)); }); // ESM new Sparticles( document.querySelector("main")); // Svelte let sparticles; function addSparticles(node) { new Sparticles(node); }
3. Customize the particles by overriding the following options and pass the options object as the second parameter to the Sparticles()
.
new Sparticles($main,{ // rate of change in alpha over time alphaSpeed: 10, // random deviation of alpha change alphaVariance: 1, // should the particles bounce off edge of canvas bounce: false, // css color as string, or array of color strings color: "random", // a custom function for setting the random colors when color="random" randomColor: randomHsl, // the number of random colors to generate when color is "random" randomColorCount: 3, // canvas globalCompositeOperation value for particles composition: "source-over", // number of particles on the canvas simultaneously count: 50, // default direction of particles in degrees direction: 180, // the "driftiness" of particles which have a horizontal/vertical direction drift: 1, // the glow effect size of each particle glow: 0, // if shape is "image", define an image url (can be data-uri, must be square (1:1 ratio)) imageUrl: "", // maximum alpha value of every particle maxAlpha: 1, // maximum size of every particle maxSize: 10, // minimum alpha value of every particle minAlpha: 0, // minimum size of every particle minSize: 1, // speed multiplier effect for larger particles (0 = none) parallax: 1, // can particles rotate rotate: true, // default rotational speed for every particle rotation: 1, // shape of particles (any of; circle, square, triangle, diamond, line, image) or "random" shape: "circle", // default velocity of every particle speed: 10, // fill style of particles (one of; "fill", "stroke" or "both") style: "fill", // particles to exhibit an alternative alpha transition as "twinkling" twinkle: false, // random deviation of particles on x-axis from default direction xVariance: 2, // random deviation of particles on y-axis from default direction yVariance: 2 });
4. Set the width & height of the canvas element.
new Sparticles($main, options, 400, 400);
5. API methods.
// destroy the Sparticles instance and remove event listeners instance.destroy(); // set the canvas size instance.setCanvasSize( width, height ); // reset all the particles instance.resetSparticles();
This awesome jQuery plugin is developed by simeydotm. For more Advanced Usages, please check the demo page or visit the official website.