JavaScript Plugin For Customizable Terminal Text Effect - TypeIt
File Size: | 20.3 KB |
---|---|
Views Total: | 17368 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
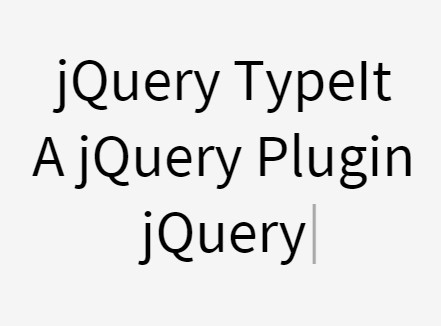
Just another typewriter-style text animation plugin that simulates someone typing and deleting over an array of words.
Note that the plugin is rewriten in pure JavaScript since TypeIt v5 so it now works with no dependencies such as jQuery.
Basic usage:
1. Download & Install the library.
# Yarn $ yarn add typeit # NPM $ npm install typeit --save
2. Import the TypeIt as a module.
import TypeIt from 'typeit';
3. Or directly load the TypeIt library from dist
folder.
<script src="dist/index.umd.js"></script> // OR FROM A CDN <script src="https://unpkg.com/typeit"></script>
4. Create a new TypeIt instance and specify the target element where text will be typed
new TypeIt('#textContainer',{ strings: "Your Content Here", }); // OR new TypeIt('#textContainer',{ strings: ["Your Content Here"], });
5. Start the typing animation.
new TypeIt('#textContainer').go();
6. Plugin's default settings.
new TypeIt('#textContainer',{ // what to type strings: 'You need a string!', // shows cursor cursor: true, // cursor character cursorChar: "|", // bink speed cursorSpeed: 1000, // delete speed deleteSpeed: null, // Choose whether you want multiple strings to be printed on top of each other breakLines: true, // The amount of time between typing multiple strings. breakDelay: 750, // Typing speed speed: 200, // The amount of time before the plugin begins typing after initalizing. startDelay: 250, // Whether to begin instance by deleting strings inside element, and then typing what strings are defined via JSON or companion functions. startDelete: false, // The amount of time (in milliseconds) between typing multiple strings. nextStringDelay: 750, // Have your string or strings continuously loop after completing. loop: false, // The amount of time between looping over a string or set of strings again. loopDelay: 750, // Handle strings as HTML, which will process tags and HTML entities html: true, // Make the typing pace irregular, as if a real person is doing it lifeLike: true, // Determines if the instance will begin typing automatically on .go(), or only when the target element becomes visible in the viewport. waitUntilVisible: false, });
7. Callback functions.
new TypeIt('#textContainer',{ beforeStep: (step, queue, instance) => { // before each step in the queue. }, beforeString: (step, queue, instance) => { // before each string in the queue. }, afterStep: (step, queue, instance) => { // after each step in the queue. }, afterString: (step, queue, instance) => { // after each string in the queue. }, afterComplete: (instance) => { // after the entire instance has completed typing. }, });
8. API methods.
new TypeIt('#textContainer', { /;/ options here }) .type('something to type') .pause(300) .break() .delete(2) .pause(250) .options({speed: 100, deleteSpeed: 75}) .empty() ..move(-5) // move the cursor .exec() // fire any arbitrary function .go() const intance = new TypeIt('#textContainer', { // options here }); instance.destroy(); instance.reset(); instance.freeze(); instance.unfreeze(); instance.is('started'); instance.is('completed'); instance.is('frozen'); instance.is('destroyed');
Changelog:
v8.2.0 (2021-12-30)
- more efficient string parsing
- more efficient printing of characters to a page
- generally less complexity throughout the library
- a 5-7% reduction in gzipped bundle size
v8.0.3 (2021-09-12)
- Updated dependencies
v8.0.2 (2021-09-02)
- Bugfixes
v8.0.0 (2021-08-22)
- BREAKING: Remove support for legacy browsers (primarily Internet Explorer).
- BREAKING: The .move() method no longer accepts 'START' or 'END' as the first paramter. Instead, it must be passed as an option in the second parameter. For example: .move('.selector', {to: 'START'}).
- ENHANCEMENT: Switch from Rollup to Microbundle for bundling.
- FEATURE: Add ability to make .type(), .delete(), and .move() methods execute instantly by passing an { instant: true } option.
- FEATURE: Allow .move() method to accept selectors for typed elements to which the cursor should move.
- FEATURE: Allow .delete() method to accept selectors for typed elements to which text should be deleted.
- FEATURE: Allow all chainable methods to accept a function that returns a value, rather than the value itself.
- FEATURE: Introduce CSS custom properties for cursor styling.
- FIX: Fix bug causing the .move() method not to work when the cursor was disabled.
- FIX: Fix bug causing hard-coded strings to mess with the lineBreak configuration option.
v6.0.2 (2019-02-06)
- Rewrite with no jQuery dependency.
v4.4.1 (2017-09-07)
- Fix visibility bug, update docs.
v4.4.0 (2017-04-04)
- add tiEmpty() companion function.
v4.3.0 (2016-12-02)
- bugfix
v4.2.3 (2016-06-08)
- improvement.
v4.2.0 (2016-06-07)
- added deleteSpeed feather.
v4.0.0 (2016-05-25)
- added more options
v3.0.1 (2016-02-15)
- Fix non-working callback function option.
v3.0.0 (2016-02-09)
- Code consolidation, remove CSS dependency, cursor options additions.
v2.0.2 (2016-01-21)
- Fix bug not allowing use of in-element string.
v2.0.1 (2016-01-08)
- Add check for existence of array to delete.
v2.0.0 (2016-01-07)
- Handle HTML tags, add loop and related options.
2015-11-19
- v1.1.0
This awesome jQuery plugin is developed by alexmacarthur. For more Advanced Usages, please check the demo page or visit the official website.