Simple Adaptive Popup Window Plugin with jQuery - MiniPopup
File Size: | 42.6 KB |
---|---|
Views Total: | 4784 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
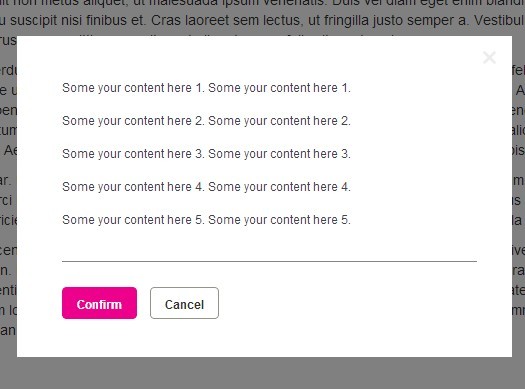
A minimal jQuery plugin used to display any Html elements into a responsive modal-style popup window with various useful options.
How to use it:
1. Include jQuery library and the miniPopup.js script in the Html page.
<script src="//code.jquery.com/jquery-1.11.1.min.js"></script> <script type="text/javascript" src="src/miniPopup.js"></script>
2. Create a button to trigger a popup window.
<input type="button" class="ShowDemo" value="Show Popup">
3. Create the Html for the popup content.
<div class="mini-popup"> <div class="mini-popup-header"> ... Popup header ... <div class="mini-popup-buttons mini-popup-close close" title="Close"></div> </div> <div class="mini-popup-content"> ... Popup body ... </div> <div class="mini-popup-footer"> ... Popup footer ... </div> </div>
4. Style the popup window whatever you like in the CSS.
.mini-popup-mask { position: absolute; z-index: 9998; top: 0px; left: 0px; background-color: #000000; display: none; width: 100%; height: 100%; filter: alpha(opacity=70); opacity: 0.7; } .mini-popup { position: absolute; z-index: 9999; width: 495px; height: auto; background: #fff; overflow: hidden; padding: 15px 0 35px 0; display: none; font-size: 12px; } .mini-popup-buttons { background: url(images/popup-buttons.png) no-repeat; display: inline-block; } *+html .mini-popup-buttons { display: inline; } .mini-popup-header { height: 13px; clear: both; } .mini-popup-close { float: right; width: 13px; height: 13px; margin-right: 16px; cursor: pointer; background-position: 0 -80px; } .mini-popup-content { margin: 0 35px 0 45px; padding-bottom: 32px; border-bottom: 1px solid #858585; color: #3D4550; } .mini-popup-content p { margin: 15px 0 0 0; line-height: 18px; } .mini-popup-footer { margin: 25px 45px 0 45px; height: 35px; } .confirm { float: left; height: 35px; overflow: hidden; } .confirm-btn { background-position: 100% 0; display: inline-block; height: 35px; position: relative; cursor: pointer; margin-left: 7px; } *+html .confirm-btn { display: inline; } .confirm-btn input, .cancel-btn input { background: none; border: none; font-weight: bold; margin: 0; padding: 9px 15px 12px 8px; cursor: pointer; overflow: visible; width: auto; height: 35px; outline: none; } .confirm-btn .confirmbtn { color: #fff; } .confirm-btn span, .cancel-btn span { height: 100%; position: absolute; top: 0; left: -7px; width: 7px; } .confirm-btn span.confirm-left { background-position: 0 0; } .cancel-btn span.cancel-left { background-position: 0 -40px; } .cancel { float: left; height: 35px; margin-left: 20px; } .cancel-btn { background-position: 100% -40px; display: inline-block; height: 35px; position: relative; cursor: pointer; } *+html .cancel-btn { display: inline; } .cancel-btn .cancelbtn { color: #333333; }
5. Bind the click function on the trigger button and set the options for the popup window.
$(function() { var popup = $('.mini-popup').miniPopup({ // layer selector maskClass: 'mini-popup-mask', // popup container selector container: 'body', // modal mode modal: true, // animation speed speed: 300, // adaptive while window resized adaptive: true, // close button selector closeButton: '.close, .cancel', // layer opacity opacity: 0.5, // before popup open trigger the function beforeOpen: function() {}, // before popup close trigger the function beforeClose: function() {} }); $('.ShowDemo').click(function() { popup.miniPopup('open'); }); });
6. Public methods.
// open a popup window $fn.miniPopup('open'); // close a popup window $fn.miniPopup('close');
This awesome jQuery plugin is developed by vanzheng. For more Advanced Usages, please check the demo page or visit the official website.