Generating Animated Particles with jQuery and Canvas
File Size: | 76.1 KB |
---|---|
Views Total: | 3344 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
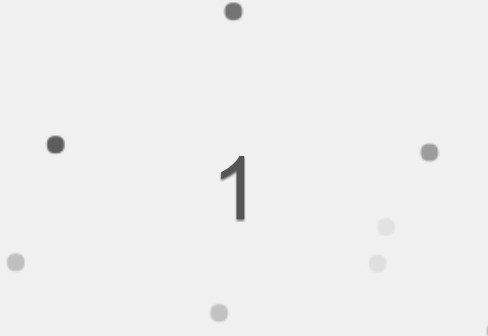
Canvas Particles is a JQuery plugin that renders animated, interactive, fully configurable particles using HTML5 canvas 2D API.
See also:
- jQuery Plugin For Particle System Background with Parallax Effect - Particleground
- Create An Animated Particle System with jQuery and Canvas
- Customizable Interactive Particles Animation with jQuery - jParticle
Basic usage:
1. Include the latest version of jQuery library and the jQuery Canvas Particles plugin at the bottom of the html page.
<script src="//code.jquery.com/jquery-2.1.4.min.js"></script> <script src="jquery.canvas.particles.min.js"></script>
2. The JavaScript to generate 10 circular particles on the container 'demo' that will stop the animation on mouse hover.
$("#demo").particles({ amount:10, duration:{random:true}, speed:{speed:1}, layout:"after", color:{random:true} }).click(function(){ $(this).particles("stop"); });
3. There are tons of options which can be passed to particles() as an object:
// amount of particles amount:10, // default state // "playing" or "stopped" state:"playing", // after finishing "change" or "remove" particle end:"change", // direction dir:{ x:1, // 1, 0 or -1 y:1, // 1, 0 or -1 xrand:true, //random dir.x yrand:true, //random dir.y rand:true, //random change of dir.x and dir.y xfunction:function(dx,px,dy,py,s,w,h){return px+=dx*s;}, //determines the x movement yfunction:function(dx,px,dy,py,s,w,h){return py+=(Math.sin(2*Math.PI*(px/w))*(dy*s))} //determines the y movement }, // image url to use as particle image:false, // radious radius:{ radius:5, // if radius random is true then this is max radius random:false, // random radius between radius.radius and radius.min min:3 // minimum radius if radius.random is false then it is omitted }, // each particle duration duration:{ duration:10000, // 1000 == 1s default 10s random:false, // random between duration.duration and duration.min min:1000, // minimum duration default 1s firststep:-2000 // time between duration end and restart if end=="change" }, // animation speed speed:{ speed:1, // default value to use on dir.xfunction and dir.yfunction random:false, // random between speed.speed and speed.min min:.2 //minimum speed default .2 times the movement }, // opacity opacity:{ opacity:1, // default opacity 1 if opacity.animation or opacity.random it is max opacity random:false, // random between opacity.opacity and opacity.min min:0, // minimum opacity default 0 animation:true, // if true opacity increases until max opacity decay:true // if true opacity decreases until min opacity }, // start position inside element position:{ x:0, // x position default 0 y:0, // y position default 0 random:false // if true get random point inside element }, // color color:{ color:{r:255,g:255,b:255}, // default color for particles rgb(255,255,255) random:false, // if true get color between color.color and color.min min:{r:0,g:0,b:0} // minimum color default rgb(0,0,0) }, // before or after element content layout:"before", // if particle position is outside element "back" return to the other side and "bounce" change direction bound:"back", // event handler when init is ready create:false, // event handler when a new particle is added return the new particle in event.detail.added addParticle:false, // event handler when the state change return "playing" or "stopped" in event.detail.state stateChange:false, // event handler when a particle is removed return the particle in event.detail.removed removeParticle:false
4. API methods.
// add new particle $(this).particles("add"); // add new particle with options $(this).particles("add",{ // options here }); // stop the animation $(this).particles("stop"); // destroy the plugin $(this).particles("destroy");
5. Events.
// create $(this).on("create.particles",function(event){}); $(this).particles( create:function(){} // Initialize with the create callback specified ); // add $(this).on("addParticle.particles",function(event){}); $(this).particles( addParticle:function(event){} // Initialize with the addParticle callback specified ); // remove $(this).on("removeParticle.particles",function(event){}); $(this).particles( removeParticle:function(event){} // Initialize with the removeParticle callback specified );
This awesome jQuery plugin is developed by UribeAbraham. For more Advanced Usages, please check the demo page or visit the official website.