Minimal Clean Pagination Plugin For jQuery - Pagination.js
File Size: | 17.7 KB |
---|---|
Views Total: | 2592 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
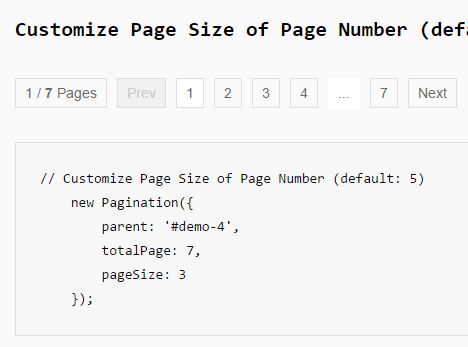
The Pagination.js jQuery plugin allows dynamically generating a minimal, clean, full-featured pagination control to page the large text content on the webpage.
How to use it:
1. To use this plugin, place the following JavaScript and CSS files into the document and we're ready to go.
<link href="css/pagination.css" rel="stylesheet"> <script src="//code.jquery.com/jquery.min.js"></script> <script src="js/pagination.js"></script>
2. Create a container element in which you want to place the pagination links.
<div class="demo" id="demo"></div>
3. Create a new pagination object and specify the number of total pages & page size.
new Pagination({ parent: '#demo', totalPage: 10, pageSize: 10 }); // or $('#demo').pagination({ totalPage: 10, pageSize: 10 });
4. Adjust the alignment of the pagination control.
new Pagination({ parent: '#demo', totalPage: 10, pageSize: 10, align: 'center' // 'left', 'right' });
5. Options and defaults.
$('#demo').pagination({ parent: null, totalPage: 0, currentPage: 1, pageSize: 5, showPages: true, showPrev: true, showNext: true, showPageNumbers: true, alwaysShowPrevNext: true, prevText: 'Prev', nextText: 'Next', ellipsisText: '...', align: 'left', beforeInit: null, afterInit: null, beforeRender: null, afterRender: null, beforeDrawPage: null, afterDrawPage: null, WRAP: '<div class="' + CLS_WRAP + '" id="{id}"></div>', LIST: '<ul class="' + CLS_LIST + '"></ul>', PAGES: '<li class="' + CLS_PAGE + ' ' + CLS_PAGES + ' ' + CLS_FIRST + '"><span class="text"><em class="' + CLS_INDEX + '">{current}</em> / <strong class="total">{total}</strong> Pages</span></li>', PREV: '<li class="' + CLS_PAGE + ' ' + CLS_PREV + '"><a class="text">{prev}</a></li>', NEXT: '<li class="' + CLS_PAGE + ' ' + CLS_NEXT + ' ' + CLS_LAST + '"><a class="text">{next}</a></li>', PAGE: '<li class="' + CLS_PAGE + ' ' + CLS_NAV + '" title="{page}"><a class="text">{page}</a></li>', FIRST: '<li class="' + CLS_PAGE + ' ' + CLS_NAV + '" title="1"><a class="text">1</a></li>', LAST: '<li class="' + CLS_PAGE + ' ' + CLS_NAV + '" title="{total}"><a class="text">{total}</a></li>', ELLIPSIS: '<li class="' + CLS_PAGE + ' ' + CLS_ELLIPSIS + '"><span class="text">{ellipsis}</span></li>', CURRENT: '<li class="' + CLS_PAGE + ' ' + CLS_NAV + ' ' + CLS_CURRENT + '"><span class="text">{page}</span></li>' });
6. API methods.
// init new Pagination().init({ // options }); // sets properties new Pagination().set({ align: 'right' }).init({ parent: '#demo', totalPage: 10 }); // gets properties new Pagination().get( prop ) // gets or sets the totalPage property var pager = new Pagination({ parent: '#demo', totalPage: 10 }).total(44); pager.total(); // -> 44 // gets or sets the current page var pager = new Pagination({ parent: '#demo', totalPage: 10 }).current(4); pager.current(); // -> 4 // gets or sets the pageSize propery var pager = new Pagination({ parent: '#demo-4', totalPage: 10, currentPage: 5 }).size(3); pager.size(); // -> 3 // reloads the plugin var pager = new Pagination({ parent: '#demo', totalPage: 10, currentPage: 5 }); pager.reload({ totalPage: 110, currentPage: 53, pageSize:7 }); // renders the pagination var pager = new Pagination({ parent: '#demo-7', totalPage: 10, currentPage: 5 }); pager.render(); // sets the alignment var pager = new Pagination({ parent: '#demo', totalPage: 11, currentPage: 5 }); pager.align('right'); // shows the pagination new Pagination().show(); // hides the pagination new Pagination().hide(); // disables the pagination new Pagination().disable(); // enables the pagination new Pagination().enable(); // destroys the pagination new Pagination().destroy(); // updates the pagination new Pagination().update(); // returns true/false new Pagination().isDisabled(); // prev page new Pagination().prev(); // next page new Pagination().next(); // goes to a specific page new Pagination().change( page );
7. Events.
// before change var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('beforeChange', function(args){ $.each(args, function(key,value){ console.log(value); }); }); // after change var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('afterChange', function(args){ $.each(args, function(key,value){ console.log(value); }); }); // before show var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.hide().on('afterChange', function(args){ $.each(args, function(key,value){ console.log(value); }); }).show(); // after show var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.hide().on('afterShow', function(args){ $.each(args, function(key,value){ console.log(value); }); }).show(); // before hide var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('beforeHide', function(args){ $.each(args, function(key,value){ console.log(value); }); }).hide(); // after hide var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('afterHide', function(args){ $.each(args, function(key,value){ console.log(value); }); }).hide(); // before disable var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('beforeDisable', function(args){ $.each(args, function(key,value){ console.log(value); }); }).disable(); // after disable var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('afterDisable', function(args){ $.each(args, function(key,value){ console.log(value); }); }).disable(); // before enable var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.disable().on('beforeEnable', function(args){ $.each(args, function(key,value){ console.log(value); }); }).enable(); // after enable var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.disable().on('afterEnable', function(args){ $.each(args, function(key,value){ console.log(value); }); }).enable(); // before destroy var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('beforeDestroy', function(args){ $.each(args, function(key,value){ console.log(value); }); }).destroy(); // after destroy var Pager = new Pagination({ parent: '#demo', totalPage: 15, align: 'right' }); Pager.on('afterDestroy', function(args){ $.each(args, function(key,value){ console.log(value); }); }).destroy();
This awesome jQuery plugin is developed by yaohaixiao. For more Advanced Usages, please check the demo page or visit the official website.