HTML5 Tag Cloud Plugin With JavaScript & jQuery - TagCanvas
File Size: | 71.1 KB |
---|---|
Views Total: | 9475 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
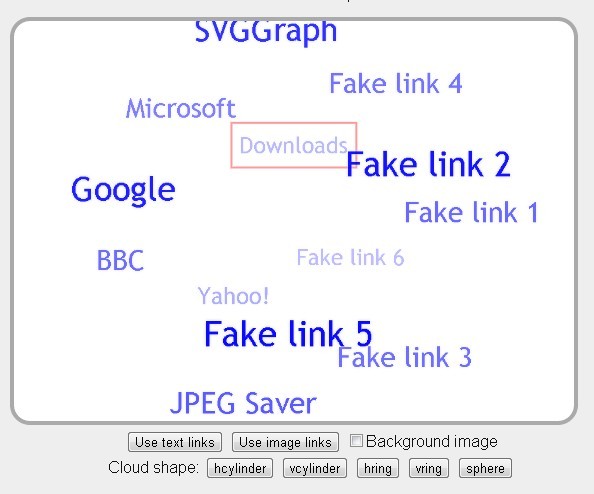
TagCanvas is a jQuery & Vanilla JavaScript Plugin for creating a HTML5 canvas based tag cloud with tons of customization options.
Features:
- Smooth animations.
- Plays a sound when entering a tag.
- Draggable tags.
- Pauses movement when interacting with tags.
- Custom shapes.
How to use it:
1. Load the TagCanvas library in the document.
<!-- Vanilla JS Version --> <script src="tagcanvas.min.js"></script> <!-- jQuery Version --> <script src="/path/to/cdn/jquery.min.js"></script> <script src="jquery.tagcanvas.min.js"></script>
2. Create an empty Canvas element for the tag cloud.
<canvas id="myCanvas"> ... </canvas>
3. Create a list of tag links as follows:
<div id="tags"> <ul> <li><a href="#">Tag 1</a></li> <li><a href="#">Tag 2</a></li> <li><a href="#">Tag 3</a></li> ... more tags here </ul> </div>
4. Initialize the plugin and render the tag cloud on the HTML5 canvas element you just created:
// Vanilla JS Version TagCanvas.Start('myCanvas','tags',{ // options here }); // jQuery Version $('#myCanvas').tagcanvas({ // options here }, 'tags')
5. Available plugin options to customize the tag cloud:
{ z1: 20000, z2: 20000, z0: 0.0002, freezeActive: false, freezeDecel: false, activeCursor: 'pointer', pulsateTo: 1, pulsateTime: 3, reverse: false, depth: 0.5, maxSpeed: 0.05, minSpeed: 0, decel: 0.95, interval: 20, minBrightness: 0.1, maxBrightness: 1, outlineColour: '#ffff99', outlineThickness: 2, outlineOffset: 5, outlineMethod: 'outline', // 'classic', 'block', 'colour', 'size', 'none' outlineRadius: 0, textColour: '#ff99ff', textHeight: 15, textFont: 'Helvetica, Arial, sans-serif', shadow: '#000', shadowBlur: 0, shadowOffset: [0,0], initial: null, hideTags: true, zoom: 1, weight: false, weightMode: 'size', // 'colour', 'both', 'bgcolour', 'bgoutline', 'outline' weightFrom: null, weightSize: 1, weightSizeMin: null, weightSizeMax: null, weightGradient: {0:'#f00', 0.33:'#ff0', 0.66:'#0f0', 1:'#00f'}, txtOpt: true, txtScale: 2, frontSelect: false, wheelZoom: true, zoomMin: 0.3, zoomMax: 3, zoomStep: 0.05, shape: 'sphere', // 'vcylinder', 'hcylinder', 'hring', 'vring', ... // Limits rotation of the cloud using the mouse. // A value of "x" limits rotation to the x-axis, "y" limits rotation to the y-axis. // A value of "xy" will prevent the cloud rotating in response to the mouse - the cloud will only move if the initial option is used to give it a starting speed. lock: null, tooltip: null, // 'native' for operating system tooltips; 'div' for div-based. tooltipDelay: 300, tooltipClass: 'tctooltip', radiusX: 1, radiusY: 1, radiusZ: 1, stretchX: 1, stretchY: 1, offsetX: 0, offsetY: 0, shuffleTags: false, noSelect: false, noMouse: false, imageScale: 1, paused: false, dragControl: false, dragThreshold: 4, centreFunc: Nop, splitWidth: 0, animTiming: 'Smooth', // or 'Linear' clickToFront: false, fadeIn: 0, padding: 0, bgColour: null, bgRadius: 0, bgOutline: null, bgOutlineThickness: 0, outlineIncrease: 4, textAlign: 'centre', // 'left', 'right' textVAlign: 'middle', // 'top', 'bottom' imageMode: null, // 'image', 'text', 'both' imagePosition: null, // 'left', 'right', 'top', 'bottom' imagePadding: 2, imageAlign: 'centre', // 'left', 'right' imageVAlign: 'middle', // 'top', 'bottom' noTagsMessage: true, centreImage: null, // Uses a built-in centreFunc callback function to draw the image at full size in the middle of the canvas. pinchZoom: false, repeatTags: 0, minTags: 0, imageRadius: 0, scrollPause: false, outlineDash: 0, outlineDashSpace: 0, outlineDashSpeed: 1, activeAudio: '', // audio path audioVolume: 1, audioIcon: 1, audioIconSize: 20, audioIconThickness: 2, audioIconDark: 0, altImage: 0, // Set to true to swap to the second image in the element when the tag is active. }
v2.11.1 (2022-03-10) v2.9.0 (2016-03-02) v2.5.0 (2014-07-04) v2.4.0 (2014-05-28) v2.2.0 (2013-07-08)
// pause the animation
TagCanvas.Pause(canvasId);
$(selector).tagcanvas("pause");
// resume the animation
TagCanvas.Resume(canvasId);
$(selector).tagcanvas("resume");
// reload the tags
TagCanvas.Reload(canvasId);
$(selector).tagcanvas("reload");
// update the tags
TagCanvas.Update(canvasId);
$(selector).tagcanvas("update");
// bring a tag to the front of the cloud
TagCanvas.TagToFront(canvasId, {
id: "tag1",
index: null, // Array index of tag to move to front
text: null, // Text content of tag to move to front
time: 500, // Duration of movement in milliseconds
callback: null, // Function to call after movement has completed
active: false, // If true, highlights the tag while it is in motion
});
$(selector).tagcanvas("tagtofront", {
// TagToFront options here
});
// move a tag to another position
TagCanvas.RotateTag(canvasId{
id: "tag1",
index: null, // Array index of tag to move to front
text: null, // Text content of tag to move to front
time: 500, // Duration of movement in milliseconds
callback: null, // Function to call after movement has completed
active: false, // If true, highlights the tag while it is in motion
lat: null, // Latitude of destination
lng: null, // Longitude of destination
});
$(selector).tagcanvas("rotatetag", {
// RotateTag options here
});
// set the cloud speed and direction
TagCanvas.SetSpeed(canvasId, [0.5, -0.25]);
$(selector).tagcanvas("setspeed", [0.5, -0.25]);
// remove the tag cloud
TagCanvas.Delete(canvasId);
$(selector).tagcanvas("delete");
Changelog:
This awesome jQuery plugin is developed by goat1000. For more Advanced Usages, please check the demo page or visit the official website.