Search Data With Advanced Filter Criteria - Structured Filter
File Size: | 140 KB |
---|---|
Views Total: | 4314 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
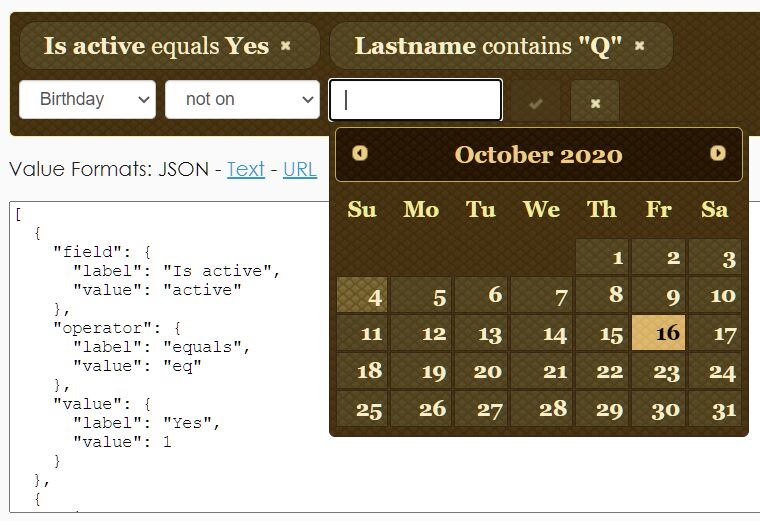
If you're looking for a filter plugin that enables the user to search content within your data using various filter combinations, you're at the right place.
Structured Filter is an advanced jQuery & jQuery UI filtering plugin that can be used to filter complex data using structured search conditions such as equal, greater than, less than, Yes/No, contain, and much more.
Default Filter Conditions:
- boolean: Yes (1), No (0)
- date: on (eq), not on (ne), after (gt), before (lt), between (bw), not between (nbw), is empty (null), is not empty (nn)
- list: any of (in), equal (eq)
- number: = (eq), != (ne), > (gt), < (lt), is empty (null), is not empty (nn)
- text: equals (eq), not equal (ne), starts with (sw), contains (ct), doesn't contain (nct), finishes with (fw), is empty (null), is not empty (nn)
- time: at (eq), not at (ne), after (gt), before (lt), between (bw), not between (nbw), is empty (null), is not empty (nn)
How to use it:
1. Install and import the Structured Filter plugin into the document.
# NPM $ npm i structured-filter --save
<!-- jQuery & jQuery UI --> <script src="/path/to/cdn/jquery.min.js"></script> <link href="/path/to/cdn/jquery-ui.min.css" rel="stylesheet" /> <script src="/path/to/cdn/jquery-ui.min.js"></script> <!-- jQuery Structured Filter --> <link href="/path/to/css/structured-filter.css" rel="stylesheet" /> <script src="/path/to/js/structured-filter.js"></script>
2. Prepare your data as follows:
// sample data for Structured-Filter var contacts=[ { type:"text", id:"Lastname", label:"Lastname"}, { type:"text", id:"Firstname", label:"Firstname"}, { type:"boolean", id:"active", label:"Is active"}, { type:"number", id:"age", label:"Age"}, {type:"list", id:"category", label:"Category", list:[ {id:'1', label:"Family"}, {id:'2', label:"Friends"}, {id:'3', label:"Business"}, {id:'4', label:"Acquaintances"}, {id:'5', label:"Other"} ] }, {type:"date", id:"bday", label:"Birthday"}, {type:"text", id:"phone", label:"Phone"}, {type:"text", id:"cell", label:"Mobile"}, {type:"text", id:"Address1", label:"Address"}, {type:"text", id:"City", label:"City"}, {type:"list", id:"State", label:"State", list:[ {id:"AL", label:"Alabama"}, {id:"AK", label:"Alaska"}, {id:"AZ", label:"Arizona"} ] }, {type:"text", id:"Zip", label:"Zip"}, {type:"list", id:"Country", label:"Country", list:[ {label: 'Afghanistan', id: 'AF'}, {label: 'Åland Islands', id: 'AX'}, // ... ] } ]
3. Initialize the plugin to generate a filter UI on the page. That's it.
<div id="myFilter"></div>
$('#myFilter').structFilter({ fields: contacts })
4. Add custom filter conditions to the filter UI. Each condition must contain field
, operator
, and value
properties as follows:
$('#myFilter').structFilter("addCondition", { "field": { "label": "Lastname", "value": "Lastname" }, "operator": { "label": "contains", "value": "ct" }, "value": { "label": "\"N\"", "value": "N" } });
5. All available options to customize the plugin.
$('#myFilter').structFilter({ // data to be filterable fields: [], // date format dateFormat: 'mm/dd/yy', // highlights the last added or modified filter highlight: true, // show/hide button labels buttonLabels: false, // show/hide submit button submitButton: false, // provides hidden fields with the conditions' values to be submitted with the form submitReady: false, // disables operators from conditions disableOperators: false })
6. API methods.
// remove all filters $('#myFilter').structFilter("clear"); // get the number of filters $('#myFilter').structFilter("length"); // remove a condition (zero indexed) $('#myFilter').structFilter("removeCondition", 0); // get or set the filter $('#myFilter').structFilter("val"); $('#myFilter').structFilter("val", data); $("#myFilter").structFilter("valText"); $("#myFilter").structFilter("valUrl");
7. Event handlers.
$('#myFilter').on("change.search", function(event){ // after conditions are changed }); $('#myFilter').on("submit.search", function(event){ // on submit });
This awesome jQuery plugin is developed by evoluteur. For more Advanced Usages, please check the demo page or visit the official website.