Dynamic List Filter & Search In jQuery - Search Filtering
File Size: | 4.19 KB |
---|---|
Views Total: | 4903 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
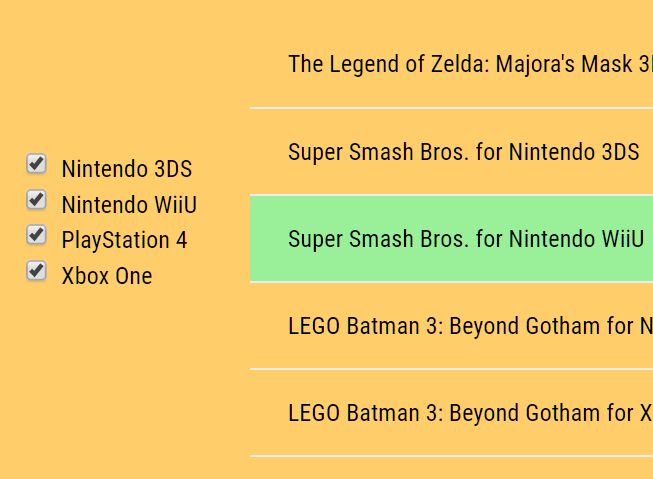
A super tiny jQuery filtering script that provides collection (category) filter and instant search functionalities for a long dynamic list on the webpage.
How to use it:
1. Define your data (e.g. products, books, contacts) containing titles, ID, and category names in a JS array as follows:
const data = [ { title: "The Legend of Zelda: Majora's Mask 3D", id: 1, category: "Nintendo 3DS", }, { title: "Super Smash Bros.", id: 2, category: "Nintendo 3DS", }, { title: "Super Smash Bros.", id: 3, category: "Nintendo WiiU", }, { title: "LEGO Batman 3: Beyond Gotham", id: 4, category: "Nintendo WiiU", }, { title: "LEGO Batman 3: Beyond Gotham", id: 5, category: "Xbox One", }, { title: "LEGO Batman 3: Beyond Gotham", id: 6, category: "PlayStation 4", }, { title: "Far Cry 4", id: 7, category: "PlayStation 4", }, { title: "Far Cry 4", id: 8, category: "Xbox One", }, { title: "Call of Duty: Advanced Warfare", id: 9, category: "PlayStation 4", }, { title: "Call of Duty: Advanced Warfare", id: 10, category: "Xbox One", }, ];
2. Assign matched ID to the list items.
<ul id="games"> <li data-id="1">The Legend of Zelda: Majora's Mask 3D for Nintendo 3DS</li> <li data-id="2">Super Smash Bros. for Nintendo 3DS</li> <li data-id="3">Super Smash Bros. for Nintendo WiiU</li> <li data-id="4">LEGO Batman 3: Beyond Gotham for Nintendo WiiU</li> <li data-id="5">LEGO Batman 3: Beyond Gotham for Xbox One</li> <li data-id="6">LEGO Batman 3: Beyond Gotham for PlayStation 4</li> <li data-id="7">Far Cry 4 for PlayStation 4</li> <li data-id="8">Far Cry 4 for Xbox One</li> <li data-id="9">Call of Duty: Advanced Warfare for PlayStation 4</li> <li data-id="10">Call of Duty: Advanced Warfare for Xbox One</li> </ul>
3. Create a search field to filter through these list items by typing keywords.
<form id="search" action="" method="get"> <fieldset> <input type="search" placeholder="Enter a game name"> <input type="submit" value="Search"> </fieldset> </form>
4. Create checkboxes to filter through these list items by category.
<form action="" method="get"> <fieldset> <ul> <li> <label> <input type="checkbox" value="Nintendo 3DS" checked> Nintendo 3DS </label> </li> <li> <label> <input type="checkbox" value="Nintendo WiiU" checked> Nintendo WiiU </label> </li> <li> <label> <input type="checkbox" value="PlayStation 4" checked> PlayStation 4 </label> </li> <li> <label> <input type="checkbox" value="Xbox One" checked> Xbox One </label> </li> </ul> </fieldset> </form>
5. Load the necessary jQuery JavaScript library in the document.
<script src="/path/to/cdn/jquery.min.js"></script>
6. The main jQuery script to enable the filters.
$(() => { const $checkboxes = $('input[type=checkbox]'); const $games = $('#games'); const $form = $('form#search'); const $input = $('input[type=search]'); let searchIds = data.map(({ id }) => id); let checkboxIds = data.map(({ id }) => id); const filterFunc = (_, elem) => { const id = parseInt(elem.dataset.id, 10); return searchIds.includes(id) && checkboxIds.includes(id); }; $checkboxes.on('change', () => { const values = Array.from($checkboxes.filter(':checked') .map((_, elem) => elem.value)); checkboxIds = data.filter(({ category }) => ( values.includes(category) )).map(({ id }) => id); $games.hide(); $games.filter(filterFunc).show(); }); $form.on('submit', (e) => { e.preventDefault(); const terms = $input.val(); searchIds = data.filter(({ title }) => ( title.toLowerCase().includes(terms.toLowerCase()) )).map(({ id }) => id); $games.hide(); $games.filter(filterFunc).show(); }); });
This awesome jQuery plugin is developed by johnisom. For more Advanced Usages, please check the demo page or visit the official website.