Morphing Button Modal With jQuery And CSS3
File Size: | 6.89 KB |
---|---|
Views Total: | 252 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
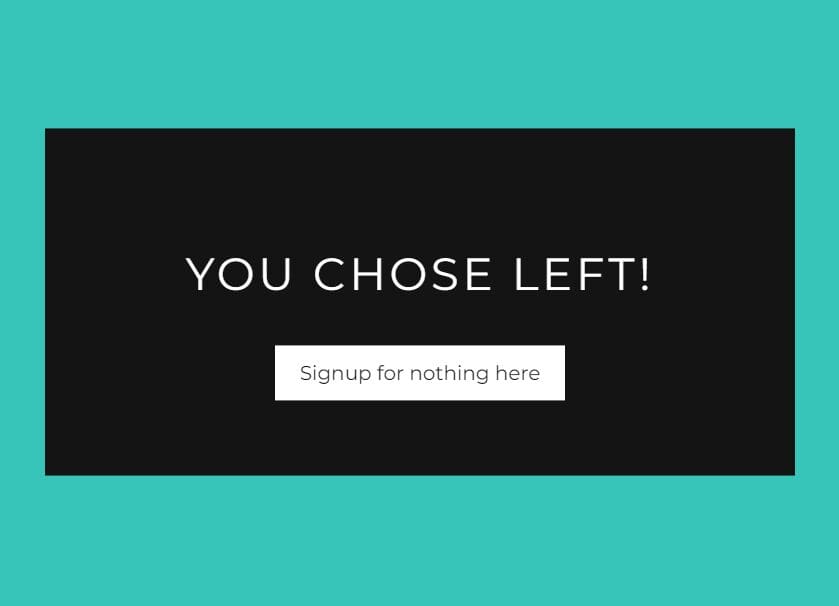
A creative button group that morphs the button into an inline modal using jQuery and CSS3 transforms & transitions. Inspired by Material Design.
It is not just something that will enhance the user's visual experience, but also something that will improve their experience while interacting with the site.
How to use it:
1. Create a button group that contains two action buttons as follows:
<div class="buttons-ctn"> <a href="" class="button button--left"><span>Left</span></a> <a href="" class="button button--right"><span>Right</span></a> </div>
2. Create the modal for each button.
<div class="button__content button__content--left"> <h2>You chose left!</h2> <a href="">Signup for nothing here</a> </div> <div class="button__content button__content--right"> <h2>You chose right!</h2> <a href="">Signup for nothing here</a> </div>
3. The necessary CSS styles.
.buttons-ctn { will-change: transform; position: absolute; top: 50%; left: 50%; margin-left: -140px; margin-top: -30px; transform-style: preserve-3d; -webkit-backface-visibility: hidden; backface-visibility: hidden; } .button { will-change: transform; position: relative; float: left; display: inline-block; padding: 20px; width: 140px; text-align: center; line-height: normal; transition: all 0.35s cubic-bezier(0.175, 0.885, 0.32, 1.275); } .button--left { background: #141414; color: white; } .button--right { background: #ebebeb; color: #141414; } .button--active { cursor: default; } .button--active span { opacity: 0; } .button__content { display: block; position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); padding: 60px 20px; text-align: center; width: 600px; visibility: hidden; opacity: 0; z-index: 10; color: white; } .button__content--left { color: white; } .button__content--left a { color: #141414; background: white; } .button__content--right { color: #141414; } .button__content--right a { color: white; background: #141414; } .button__content--active { opacity: 1; visibility: visible; } .button__content a { display: inline-block; padding: 10px 20px; } .button__content h2 { font-size: 36px; text-transform: uppercase; letter-spacing: 3px; font-weight: 300; } @media (max-width: 650px) { .button__content { width: 295px; } }
4. Load the latest jQuery library at the end of the document.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
5. The main script to activate the button modal.
var button = $('.button'); var content = $('.button__content'); var win = $(window); var expand = function() { if (button.hasClass('button--active')) { return false; } else { var W = win.width(); var xc = W / 2; var that = $(this); var thatWidth = that.innerWidth() / 2; var thatOffset = that.offset(); var thatIndex = that.index(); var other; if (!that.next().is('.button')) { other = that.prev(); } else { other = that.next(); } var otherWidth = other.innerWidth() / 2; var otherOffset = other.offset(); // content box stuff var thatContent = $('.button__content').eq(thatIndex); var thatContentW = thatContent.innerWidth(); var thatContentH = thatContent.innerHeight(); // transform values for button var thatTransX = xc - thatOffset.left - thatWidth; var otherTransX = xc - otherOffset.left - otherWidth; var thatScaleX = thatContentW / that.innerWidth(); var thatScaleY = thatContentH / that.innerHeight(); that.css({ 'z-index': '2', 'transform': 'translateX(' + thatTransX + 'px)' }); other.css({ 'z-index': '0', 'opacity': '0', 'transition-delay': '0.05s', 'transform': 'translateX(' + otherTransX + 'px)' }); that.on('transitionend webkitTransitionEnd', function() { that.css({ 'transform': 'translateX(' + thatTransX + 'px) scale(' + thatScaleX +',' + thatScaleY + ')', }); that.addClass('button--active'); thatContent.addClass('button__content--active'); thatContent.css('transition', 'all 1s 0.1s cubic-bezier(0.23, 1, 0.32, 1)'); that.off('transitionend webkitTransitionEnd'); }); return false; } }; var hide = function(e) { var target= $(e.target); if (target.is(content)) { return; } else { button.removeAttr('style').removeClass('button--active'); content.removeClass('button__content--active').css('transition', 'all 0.2s 0 cubic-bezier(0.23, 1, 0.32, 1)'); } }; var bindActions = function() { button.on('click', expand); win.on('click', hide); }; var init = function() { bindActions(); }; init();
This awesome jQuery plugin is developed by ettrics. For more Advanced Usages, please check the demo page or visit the official website.