Simple 3D Rotating Slider With jQuery And CSS3 Transforms
File Size: | 1.69 KB |
---|---|
Views Total: | 6582 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
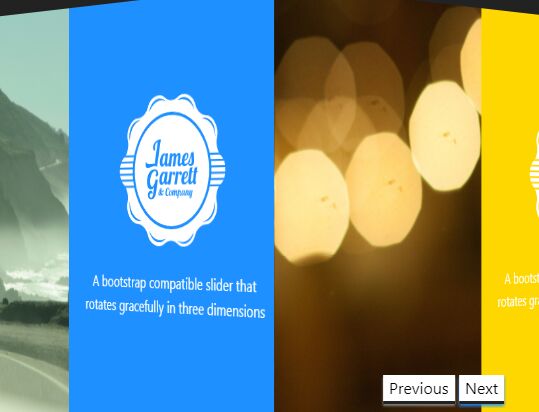
This is a simple, elegant, responsive, Bootstrap compatible 3D rotating slider that rotates gracefully in three dimensions, built using CSS3 2D/3D transforms and a little bit of jQuery script.
How to use it:
1. Include Bootstrap's style sheet for the basic styling of the 3D slider.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/css/bootstrap.min.css" integrity="sha384-/Y6pD6FV/Vv2HJnA6t+vslU6fwYXjCFtcEpHbNJ0lyAFsXTsjBbfaDjzALeQsN6M" crossorigin="anonymous" >
2. Add your own content to the slider cubes.
<div class="wrap"> <section class="cube-container"> <div id="cube"> <figure class="front"> <div class="col-md-8 col-sm-12" style="background-image: url('1.jpg');"></div> <div class="col-md-4" style="height:inherit;display:inline-block;"> <article> <img src="logo.svg" alt="logo" class="mb-4"> <p class="text-center">A bootstrap compatible slider that rotates gracefully in three dimensions</p> </article> </div> </figure> <figure class="back"> <div class="col-md-8" style="background-image:url('2.jpg');"></div> <div class="col-md-4" style="height:inherit;display:inline-block;"> <article> <img src="logo.svg" alt="logo" class="mb-4"> <p class="text-center">A bootstrap compatible slider that rotates gracefully in three dimensions</p> </article> </div> </figure> <figure class="right"> <div class="col-md-8" style="background-image:url('3.jpg');"></div> <div class="col-md-4" style="height:inherit;display:inline-block;"> <article> <img src="logo.svg" alt="logo" class="mb-4"> <p class="text-center">A bootstrap compatible slider that rotates gracefully in three dimensions</p> </article> </div> </figure> <figure class="left"> <div class="col-md-8" style="background-image:url('4.jpg');"></div> <div class="col-md-4" style="height:inherit;display:inline-block;"> <article> <img src="logo.svg" alt="logo" class="mb-4"> <p class="text-center">A bootstrap compatible slider that rotates gracefully in three dimensions</p> </article> </div> </figure> <figure class="top">5</figure> <figure class="bottom">6</figure> </div> </section> </div>
3. Create navigation buttons for the 3D slider.
<div class="button-wrap"> <button class="previous">Previous</button> <button class="next">Next</button> </div>
4. The main CSS/CSS3 styles.
.cube-container { width: 100vw; height: 100vh; position: relative; perspective: 2000px; background: #222; } #cube { width: 100vw; height: 100vh; position: absolute; transform-style: preserve-3d; } #cube figure { margin: 0; width: 100vw; height: 100vh; display: flex; position: absolute; background: #222; color: white; } #cube figure div { height: inherit; background-size: cover; background-position: center; } #cube .front { transform: rotateY(0deg) translateZ(50vw); } #cube .back { transform: rotateY(180deg) translateZ(50vw); background: gold; } #cube .right { transform: rotateY(90deg) translateZ(50vw); background: dodgerblue; } #cube .left { transform: rotateY(-90deg) translateZ(50vw); background: cadetblue; } #cube .top { transform: rotateX(90deg) translateZ(50vw); background: mintgreen; } #cube .bottom { transform: rotateX(-90deg) translateZ(50vw); background: lavender; } #cube { transform: translateZ(-50vw); } #cube { transition: transform 1.5s ease-in-out; } .button-wrap { position: absolute; bottom: 10px; right: 12.5%; transform: translateX(12.5%); } article { position: absolute; top: 50%; transform: translateY(-50%); } article img { max-height: 150px; } .button-wrap button { background: white; border: 0px; border-bottom: 3px solid #222; box-shadow: grey 0px 2px 2px 0px; cursor: pointer; } .button-wrap:hover button:not(:hover) { background: rgba(255, 255, 255, 0.6); } .button-wrap button:focus { box-shadow: dodgerblue 0px 2px 2px 0px; outline: none; }
5. Include the latest version of jQuery JavaScript library at the bottom of the web page.
<script src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
6. Enable the navigation buttons to rotate through the cubes when needed.
var counter = 0; $('.next').on('click', function(){ counter -= 90; var rotation = 'translateZ( -50vw ) rotateY( ' + counter + 'deg )'; $('#cube').css('transform', rotation); }); $('.previous').on('click', function(){ counter += 90; var rotation = 'translateZ( -50vw ) rotateY( ' + counter + 'deg )'; $('#cube').css('transform', rotation); });
This awesome jQuery plugin is developed by James Garrett. For more Advanced Usages, please check the demo page or visit the official website.