Create A Simple iOS Style Slider with jQuery and CSS - UI Slider
File Size: | 8.03 KB |
---|---|
Views Total: | 4546 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
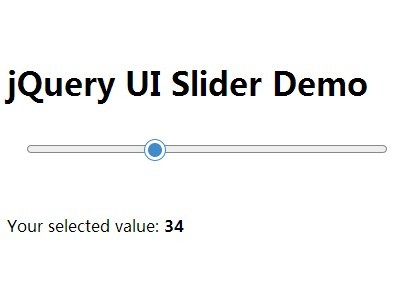
UI Slider is a simple jQuery plugin that converts an DIV elements into an iOS-Style range slider with some customization options.
How to use it:
1. Load the necessary jQuery library and the jQuery UI Slider plugin in the document.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="js/jquery.ui-slider.js"></script>
2. Load the required jquery.ui-slider.css
in the head section of the document. Feel free to modify the styles to your taste.
<link rel="stylesheet" href="css/jquery.ui-slider.css">
3. Create an empty container element for the range slider.
<div class="ui-slider-control"></div>
4. You can also create an element to display the current value you select.
<p id="value">0</p>
5. Initialize the plugin with options and output the current value using handleAction()
function.
$(document).ready(function () { var position = 30; $('.ui-slider-control') .UISlider({ min: 1, max: 100, value: position, smooth: false }) .on('change', function (event, value) { $('#value').text(value); }); $('#value').text(position); });
6. More usages.
// Create: $(elem).UISlider({ min: 0, max: 99, value: 0, smooth: true, seekOnTrack: true, seekOnOwner: true, vertical: false, // true - to use vertical orientation createElements: true, allowFocus: false }); // Read: $(elem).UISlider('value'); // => 50 $(elem).UISlider('max'); // => 99 // Update: $(elem).UISlider('value', 3); $(elem).UISlider('update', {min: 1, max: 10}); // Destroy: $(elem).UISlider('destroy'); // Events: $(elem).on('thumbmove', function (value) { ... }); // when slider thumb is moving $(elem).on('change', function (value) { ... } ); // when user changes are complete
Change log:
2014-09-03
- Added vertical style; 'start' and 'end' events; 'allowFocus' property.
2014-07-14
- Fixed API behavior
This awesome jQuery plugin is developed by DenisIzmaylov. For more Advanced Usages, please check the demo page or visit the official website.