Modern Split Carousel Slider In jQuery - Split.js
File Size: | 23.1 KB |
---|---|
Views Total: | 4913 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
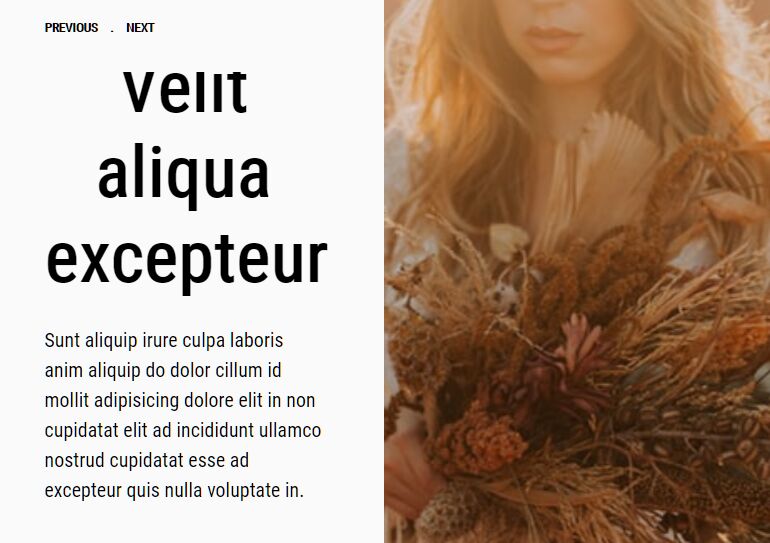
A modern jQuery based split slider/carousel plugin that enables the user to navigate through slides with split panel transitions powered by CSS3 animations.
How to use it:
1. Load the Split.js plugin's files in the HTML page which has jQuery library loaded.
<link rel="stylesheet" href="splitslider/split.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="splitslider/split.js"></script>
2. Add slides (descriptions, images, titles in this example) and navigation buttons to the carousel.
<div class="split myCarousel"> <div class="inner-wrapper"> <div class="slides-wrapper"> <!-- Left Panel: Descriptions --> <div class="slides content"> <div class="slide"> <div class="bodytext"> <h2 class="title">Image 1</h2> <p class="subtitle">Description 1</p> </div> </div> <div class="slide"> <div class="bodytext"> <h2 class="title">Image 2</h2> <p class="subtitle">Description 2</p> </div> </div> <div class="slide"> <div class="bodytext"> <h2 class="title">Image 3</h2> <p class="subtitle">Description 3</p> </div> </div> </div> <!-- Right Panel: Images Here --> <div class="slides photo"> <div class="slide"> <div class="image" style="background-image: url(https://www.jqueryscript.net/dummy/1.jpg);"></div> </div> <div class="slide"> <div class="image" style="background-image: url(https://www.jqueryscript.net/dummy/4.jpg);"></div> </div> <div class="slide"> <div class="image" style="background-image: url(https://www.jqueryscript.net/dummy/5.jpg);"></div> </div> </div> <!-- Left Panel: Titles --> <div class="slides title"> <div class="slide red"> <div class="bodytext "> <h2 class="title">Title 1</h2> <a class="link" href="#"><span>Link 1</span></a> </div> </div> <div class="slide blue"> <div class="bodytext "> <h2 class="title">Title 2</h2> <a class="link" href="#"><span>Link 2</span></a> </div> </div> <div class="slide green"> <div class="bodytext "> <h2 class="title">Title 3</h2> <a class="link" href="#"><span>Link 3</span></a> </div> </div> </div> <!-- Right Panel: Prices --> <div class="slides price"> <div class="slide"> <div class="bodytext "> <span class="title red">Special price</span> <span class="label" href="#">$998,500</span> </div> </div> <div class="slide"> <div class="bodytext "> <span class="title blue">Special price</span> <span class="label" href="#">$1,112,500</span> </div> </div> <div class="slide"> <div class="bodytext "> <span class="title green">Special price</span> <span class="label" href="#">$889,750</span> </div> </div> </div> <!-- Carousel Counter --> <div class="slides counter"> <div class="slide"> <span>01</span> </div> <div class="slide"> <span>02</span> </div> <div class="slide"> <span>03</span> </div> </div> <div class="counter-content"> <hr> <span>03</span> </div> </div> <!-- Navigation buttons --> <div id="arrows" class="navigation-wrapper"> <a href="#" class="navigation-arrow prev">previous</a> <span>.</span> <a href="#" class="navigation-arrow next">next</a> </div> </div> </div>
3. The core CSS styles for this example.
.myCarousel { position: relative; background-color: #fafafa; } .myCarousel .slides-wrapper .slides { position: absolute; } .myCarousel .slides-wrapper .slides.content { height: 60%; width: 45%; top: 10%; left: 0; } .myCarousel .slides-wrapper .slides.content .bodytext { padding: 0 50px; position: relative; top: 45%; transform: translateY(-50%); } .myCarousel .slides-wrapper .slides.content .title { font-size: 3.5em; margin: 0; margin-bottom: 20px; } .myCarousel .slides-wrapper .slides.content .subtitle { font-size: 1em; line-height: 1.5; } .myCarousel .slides-wrapper .slides.photo { height: 100%; width: 55%; top: 0; right: 0; } .myCarousel .slides-wrapper .slides.photo .image { width: 100%; height: 100%; background-size: cover; background-position: center; } .myCarousel .slides-wrapper .slides.title { height: 20%; width: 55%; bottom: 0; left: 0; } .myCarousel .slides-wrapper .slides.title .bodytext { padding: 0 50px; position: relative; top: 50%; transform: translateY(-50%); } .myCarousel .slides-wrapper .slides.title .title { margin: 0; color: #fff; margin-bottom: 5px; font-family: serif; font-size: 2.25em; letter-spacing: 1px; } .myCarousel .slides-wrapper .slides.title .link { position: relative; color: #fff; text-transform: uppercase; font-size: 0.65em; letter-spacing: 2px; margin-left: 3px; } .myCarousel .slides-wrapper .slides.title .link:after { content: ""; position: absolute; top: 2px; right: -15px; width: 7px; height: 7px; transform: rotate(45deg); border: 1px solid; border-left: 0; border-bottom: 0; transition: right 0.3s; } .myCarousel .slides-wrapper .slides.title .link:hover span { opacity: 0.5; } .myCarousel .slides-wrapper .slides.title .link:hover:after { right: -18px; } .myCarousel .slides-wrapper .slides.title .red { background-color: lightyellow; } .myCarousel .slides-wrapper .slides.title .red .title, .myCarousel .slides-wrapper .slides.title .red .link { color: #333; } .myCarousel .slides-wrapper .slides.title .blue { background-color: lightblue; } .myCarousel .slides-wrapper .slides.title .green { background-color: lightpink; } .myCarousel .slides-wrapper .slides.price { height: 20%; width: 25%; bottom: 0; left: 55%; background-color: #333; } .myCarousel .slides-wrapper .slides.price > * { transition-delay: 0.2s; } .myCarousel .slides-wrapper .slides.price .bodytext { padding: 0 50px; position: relative; top: 50%; transform: translateY(-50%); } .myCarousel .slides-wrapper .slides.price .bodytext span { display: block; color: #fff; } .myCarousel .slides-wrapper .slides.price .bodytext .title { text-transform: uppercase; margin-bottom: 10px; } .myCarousel .slides-wrapper .slides.price .bodytext .title.red { color: lightyellow; } .myCarousel .slides-wrapper .slides.price .bodytext .title.blue { color: lightblue; } .myCarousel .slides-wrapper .slides.price .bodytext .title.green { color: lightpink; } .myCarousel .slides-wrapper .slides.price .bodytext .label { font-size: 2em; } .myCarousel .slides-wrapper .slides.counter { position: absolute; top: 40%; right: 0; width: 50px; height: 50px; background-color: #333; color: #fff; line-height: 50px; text-align: center; font-size: 12px; } .myCarousel .slides-wrapper .counter-content { position: absolute; top: 40%; margin-top: 50px; right: 0; width: 50px; background-color: #333; } .myCarousel .slides-wrapper .counter-content hr { position: relative; display: block; transform: rotate(-45deg); width: 20px; } .myCarousel .slides-wrapper .counter-content span { display: block; text-align: center; line-height: 50px; color: white; font-size: 12px; } .myCarousel #arrows { position: absolute; top: 0; left: 0; padding: 20px 0 0 50px; } .myCarousel #arrows span { margin: 0 10px; text-transform: uppercase; font-size: 10px; line-height: 1; font-weight: bold; } .myCarousel #arrows .prev, .myCarousel #arrows .next { width: auto; height: auto; border: none !important; text-transform: uppercase; font-size: 10px; line-height: 1; font-weight: bold; color: #000; } .myCarousel #arrows .prev.disable, .myCarousel #arrows .next.disable { display: none; } .myCarousel #arrows .prev:hover, .myCarousel #arrows .next:hover { opacity: 0.5; }
4. Initialize the carousel and apply transitions to these slides. All possible transitions:
- left-to-right
- right-to-left
- bottom-to-top
- top-to-bottom
- fly-away-in
- fade-in-out
- bottom-to-top-zoom-in
- bottom-to-top-fade-out
- fly-in-top
- fly-in-bottom
- bottom-to-top-slow
- content-horizontal-vertical
- bottom-to-top-with-fade
- fade-in-out-slow
$('.myCarousel').split({ theme: 'bottom-to-top-fade-out,bottom-to-top-zoom-in,bottom-to-top-fade-out,fade-in-out,fade-in-out' });
5. Determine the transition delay. Default: 0.
$('.myCarousel').split({ theme: 'bottom-to-top-fade-out,bottom-to-top-zoom-in,bottom-to-top-fade-out,fade-in-out,fade-in-out', delay: '50' });
6. Set the height of the carousel. Default: 500.
$('.myCarousel').split({ theme: 'bottom-to-top-fade-out,bottom-to-top-zoom-in,bottom-to-top-fade-out,fade-in-out,fade-in-out', height: '600' });
7. Determine whether to loop through the slides. Default: false.
$('.myCarousel').split({ theme: 'bottom-to-top-fade-out,bottom-to-top-zoom-in,bottom-to-top-fade-out,fade-in-out,fade-in-out', infinite: 'true' });
This awesome jQuery plugin is developed by notbigmuzzy. For more Advanced Usages, please check the demo page or visit the official website.