High-performance Swiper In jQuery - SwipeScroll
File Size: | 17.9 KB |
---|---|
Views Total: | 1354 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
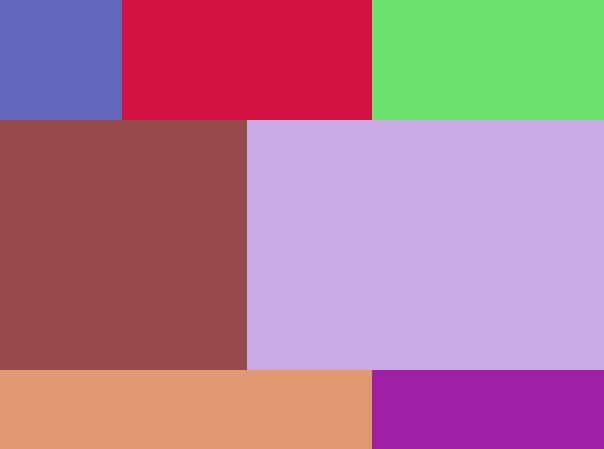
A simple yet configurable swiper plugin that allows you to scroll through a set of elements with swipe events on tablet and mobile devices.
Ideal for mobile-first scrollers, carousels, content sliders, and much more.
More Features:
- Compatible with CSS3 animations.
- Also supports mouse drag events on desktop.
- Auto snaps to the edge when the swipe ends.
- Supports both vertical and horizontal directions.
- Supports momentum scrolling.
- With a custom scrollbar or not.
How to use it:
1. Add your content to the swiper list.
<div id="scrollerWrapper"> <ul id="scroller"> <li class="scroller-child">1</li> <li class="scroller-child">2</li> <li class="scroller-child">3</li> ... </ul> </div>
2. The example CSS to create a swipable carousel from the list you just created.
#scrollerWrapper { overflow:hidden; width:100%; height:400px; position:relative; } #scroller { position:relative; top:0; left:0; height:100%; } .scroller-child { float:left; height:100%; }
3. Load the Swipe Scroll's JavaScript after jQuery.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/src/SwipeScroll.js"></script>
4. Initialize the swiper and done.
var carousel = $("#scroller").SwipeScroll({ // options here });
5. Use custom CSS3 animations instead.
var carousel = $("#scroller").SwipeScroll({ cssAnimationClass: "swipescroll-animation" });
.swipescroll-animation { -webkit-transition:all 500ms cubic-bezier(0.190, 1.000, 0.220, 1.000); -webkit-transition-timing-function:cubic-bezier(0.190, 1.000, 0.220, 1.000); -moz-transition:all 500ms cubic-bezier(0.190, 1.000, 0.220, 1.000); -moz-transition-timing-function:cubic-bezier(0.190, 1.000, 0.220, 1.000); -o-transition:all 500ms cubic-bezier(0.190, 1.000, 0.220, 1.000); -o-transition-timing-function:cubic-bezier(0.190, 1.000, 0.220, 1.000); transition:all 500ms cubic-bezier(0.190, 1.000, 0.220, 1.000); transition-timing-function:cubic-bezier(0.190, 1.000, 0.220, 1.000); }
6. Create navigation controls to scroll through the items manually.
<div id="arrows"> <a id="arrowLeft" href="#Previous">< PREVIOUS</a> <a id="arrowRight" href="#Next">NEXT ></a> </div>
var NUM_CHILDREN_VISIBLE = 1; var scroller = null; function sizeScroller(numChildrenVisible){ var scrollerChildren = $(".scroller-child"); var scrollerWidth = (100 / numChildrenVisible) * scrollerChildren.length; var childWidth = 100 / scrollerChildren.length; $("#scroller").css("width", scrollerWidth + "%"); scrollerChildren.css("width", childWidth + "%"); } function initArrows(numChildrenToScroll){ var arrowLeft = $("#arrowLeft"); var arrowRight = $("#arrowRight"); arrowLeft.on("click", function(){ scroller.SwipeScroll.scrollPrevious(numChildrenToScroll); return false; }); arrowRight.on("click", function(){ scroller.SwipeScroll.scrollNext(numChildrenToScroll); return false; }); }
7. Display a custom scrollbar.
var carousel = $("#scroller").SwipeScroll({ scrollbars: true });
8. Enable Both Directions.
var carousel = $("#scroller").SwipeScroll({ bidirectional: true });
9. More configuration options.
var carousel = $("#scroller").SwipeScroll({ // FPS for rendering fps: 30, // Seconds to scroll over timing: 0.5, // Min distance in pixels needed to scroll dragThreshold: 32, // Percent of element parent size overDrag: 0.25, // Pixels/second dragMaxSpeed: 1024, // Factor into speed when dragging dragSpeedFactor: 0.5, // Momentum useMomentum: true, // Snap useSnap: true, // Easing method when css animation is not used scrollEasing: null });
10. Event handlers.
scroller.on(SwipeScroll.EVENT_CHANGING, function(evt, args){ console.log("CHANGING TO CHILD INDEX: " + args.selectedIndex); }); scroller.on(SwipeScroll.EVENT_CHANGED, function(evt, args){ console.log("CHANGED TO CHILD INDEX: " + args.selectedIndex); });
This awesome jQuery plugin is developed by koga73. For more Advanced Usages, please check the demo page or visit the official website.