3D Rotating Content Slideshow with jQuery and CSS3
File Size: | 8.44 KB |
---|---|
Views Total: | 7161 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
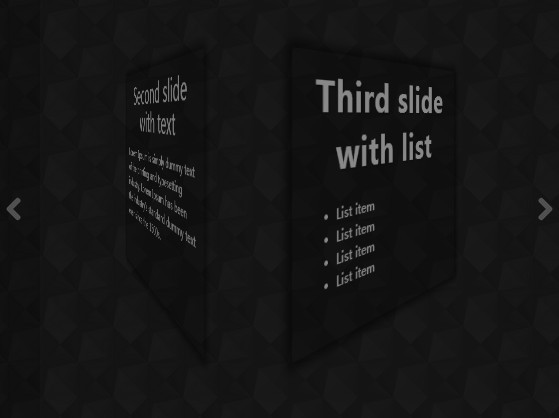
A small and cool jQuery plugin for creating a responsive & fullscreen presentation that allows you to slide through a set of Html contents like an 3D rotating/flipping cube.
See also:
- Animated 3D Cube Slideshow
- Simple 3D Flipping Cube Slideshow with jQuery and CSS3 - BoxRoll Slider
- Simple jQuery Slider Plugin with 3D Flip Effect - Impulse Slider
- Creating A 3D Flipping Gallery with jQuery and CSS3
- Responsive jQuery Slideshow with 3D CSS3 Transforms - Refine Slide
- Flexible 3D Flipping Cube Pluigin - HexaFlip
How to use it:
1. Create the Html for the 3D rotating slideshow. Wrap the slides you wish to rotate in the section
container with CSS class of 'slide'.
<div id="slideShow" class="slides-wrapper"> <section class="slide"> Section 1 </section> <section class="slide"> Section 2 </section> <section class="slide"> Section 3 </section> </div>
2. The required CSS to style the 3D rotating slideshow and to make it responsive using CSS3 media queries.
body { font-size: 24px; font-family: 'Roboto Condensed', sans-serif; overflow: hidden; min-height: 500px; min-width: 300px; background: url(diagmonds.png); position: absolute; width: 100%; height: 100%; } .hidden { display: none; } .slides-wrapper { -webkit-transform-style: preserve-3d; -webkit-perspective: 700px; -webkit-perspective-origin: 50% 100px; -webkit-transform: translateZ(0); transform-style: preserve-3d; perspective: 700px; perspective-origin: 50% 100px; transform: translateZ(0); position: absolute; width: 40%; height: 40%; top: 25%; left: 30%; z-index: 2; } .slide { width: 100%; min-height: 100%; max-height: 120%; line-height: 1.5; position: absolute; opacity: 0; font-size: 1em; color: #eee; left: 0; -webkit-transition: 0.7s all cubic-bezier(0.260, 0.860, 0.440, 0.985); transition: 0.7s all cubic-bezier(0.260, 0.860, 0.440, 0.985); -webkit-transform: translateZ(0); transform: translateZ(0); background: rgba(0,0,0,0.5); border-radius: 3px; box-shadow: 0 0 20px 10px #000; box-sizing: border-box; padding: 5%; overflow: hidden; } .slide.current { opacity: 1; -webkit-transform: translate3d(0, 0, 125px); transform: translate3d(0, 0, 125px); } .slide.next { opacity: 0; -webkit-transform: rotateY(90deg) translate3d(-20%, 0, 155px); -webkit-transform-origin: top left; transform: rotateY(90deg) translate3d(0, 0, 125px); transform-origin: top left; left: 80%; } .slide.prev { -webkit-transform: rotateY(-90deg) translate3d(20%, 0, 155px); -webkit-transform-origin: top right; transform: rotateY(-90deg) translate3d(0, 0, 125px); transform-origin: top right; opacity: 0; left: -80%; } .slide ul, .slide ol { margin: 1em 0; } .slide li { list-style-position: inside; } .nav-button { position: fixed; z-index: 10; min-width: 150px; width: 5%; height: 100%; border: none; background-color: transparent; background-position: center; background-repeat: no-repeat; text-indent: -99999px; cursor: pointer; } .nav-button:focus { outline: none; } .nav-button:hover { background-color: rgba(0, 0, 0, 0.2); } .nav-button:active { background-color: rgba(20, 20, 20, 0.3); } .nav-button.prev { background-image: url(arrow-left.png); left: 0; } .nav-button.next { background-image: url(arrow-right.png); right: 0; } @media all and (max-width: 1280px) { body { font-size: 14px; } .nav-button { background-size: 60%; min-width: 100px; } } @media all and (max-width: 1500px) { body { font-size: 22px; } } @media all and (max-width: 1024px) { body { font-size: 18px; } } @media all and (max-width: 900px) { body { font-size: 16px; } } @media all and (max-width: 750px) { .nav-button { width: 100%; height: 50px; background-size: contain; } .nav-button.prev { bottom: 0; } .nav-button.next { top: 0; } #slideShow { width: 70%; left: 15%; } }
3. Include the necessary jQuery javascript library at the bottom of your web page.
<script src="//ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
4. The Javascript to enable the 3D rotating slideshow. Add the following Javascript snippet into your JS file.
var myPresentation = function() { var wrapper = null; var defClass = null; var slides = null; var slidesNum = null; var nextButton = document.createElement('a'); var prevButton = document.createElement('a'); var currentSlide = parseInt(window.location.hash.replace('#', '') || 0); function config(_params) { var params = _params || {}; wrapper = params.wrapper || document.getElementById('slideShow'); slides = params.slides || wrapper.getElementsByClassName('slide'); slidesNum = slides.length; defClass = params.defClass || 'slide'; } function init() { if (!wrapper) { config(); } document.body.appendChild(nextButton); document.body.appendChild(prevButton); nextButton.className = 'next nav-button'; prevButton.className = 'prev nav-button'; cb_addEventListener(nextButton, 'click', goNext); cb_addEventListener(prevButton, 'click', goBack); cb_addEventListener(document, 'keyup', keyUpEv); showSlide(currentSlide); checkButtons() } function goNext() { if (slides[currentSlide + 1]) { ++currentSlide; step(); } } function goBack() { if (slides[currentSlide - 1]) { --currentSlide; step(); } } function step() { showSlide(currentSlide); window.location.hash = currentSlide; checkButtons(); return false; } function checkButtons() { if (currentSlide === 0) { prevButton.className += ' hidden'; } else if (currentSlide === slidesNum - 1) { nextButton.className += ' hidden'; } else { nextButton.className = nextButton.className.replace(' hidden', ''); prevButton.className = prevButton.className.replace(' hidden', ''); } } function keyUpEv(event) { if (event.keyCode === 37) { goBack(); } else if (event.keyCode === 39) { goNext(); } } function showSlide(step) { var i = slidesNum; if (-1 < step && step < i) { while(i--) { slides[i].className = defClass; } slides[step].className += ' current'; if (step > 0) { slides[step-1].className += ' prev'; } if (step + 1 < slidesNum) { slides[step+1].className += ' next'; } } else { return false; } } return { config: config, init: init }; }(); /** * Cross-browser Event Listener **/ function cb_addEventListener(obj, evt, fnc) { if (obj && obj.addEventListener) { obj.addEventListener(evt, fnc, false); return true; } else if (obj && obj.attachEvent) { return obj.attachEvent('on' + evt, fnc); } return false; }; myPresentation.config({ wrapper: document.getElementById('slideShow') }); myPresentation.init();
This awesome jQuery plugin is developed by anilkabobo. For more Advanced Usages, please check the demo page or visit the official website.