Fully Configurable Dropdown Date Picker Plugin With jQuery
File Size: | 66.7 KB |
---|---|
Views Total: | 11749 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
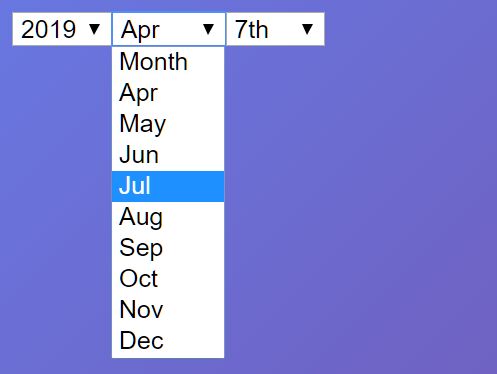
A simple yet fully configurable dropdown date picker plugin that converts the regular input field into separate "day", "month" and "year" dropdowns for more convenient date selection (e.g. DOB Picker).
Features:
- Min/max ages, dates, and years.
- Custom date format.
- Multi-language.
Installation:
# Yarn $ yarn add jquery-dropdown-datepicker # NPM $ npm install jquery-dropdown-datepicker --save
How to use it:
1. Download and include the latest version of jQuery Dropdown Datepicker plugin from dist
folder.
<!-- jQuery First --> <script src="https://code.jquery.com/jquery-3.4.1.slim.min.js" integrity="sha384-J6qa4849blE2+poT4WnyKhv5vZF5SrPo0iEjwBvKU7imGFAV0wwj1yYfoRSJoZ+n" crossorigin="anonymous"></script> <!-- Then Plugin's Script --> <script src="dist/jquery-dropdown-datepicker.min.js"></script> <!-- Or from a CDN --> <script src="https://unpkg.com/jquery-dropdown-datepicker"></script>
2. Attach the plugin to an input field and done.
<input type="text" id="date">
$(function(){ $('#date').dropdownDatepicker(); });
3. Set the initial date. Default: null.
$('#date').dropdownDatepicker({ defaultDate: '2019-04-05' });
4. Customize the date format.
$('#date').dropdownDatepicker({ defaultDateFormat: 'yyyy-mm-dd', displayFormat: 'dmy', submitFormat: 'yyyy-mm-dd' });
5. Set the min/max ages, dates, and years. Default: null.
$('#date').dropdownDatepicker({ minAge: null, maxAge: null, minYear: null, maxYear: null, minDate: null, maxDate: null, });
6. Enabled/disable past and/or future dates. Ideal for date range picker. Default: true.
$('#date').dropdownDatepicker({ allowPast: false, allowFuture: false, });
7. Localize the dropdown date picker.
$('#date').dropdownDatepicker({ dayLabel: 'Day', monthLabel: 'Month', yearLabel: 'Year', monthLongValues: ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'], monthShortValues: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'], initialDayMonthYearValues: ['Day', 'Month', 'Year'], daySuffixValues: ['st', 'nd', 'rd', 'th'] });
8. Customize the month format: short, long(default), or numeric.
$('#date').dropdownDatepicker({ monthFormat: 'short' });
9. More configuration options with default values.
$('#date').dropdownDatepicker({ submitFieldName: 'date', wrapperClass: 'date-dropdowns', dropdownClass: null, daySuffixes: true, monthSuffixes: true, required: false // is required field? sortYear: 'desc' // or 'asc' });
10. Execute functions when a date is changed.
$('#date').dropdownDatepicker({ onChange: function(day, month, year) { // do something } onDayChange: function(day, month, year) { // do something } onMonthChange: function(day, month, year) { // do something } onYearChange: function(day, month, year) { // do something } });
This awesome jQuery plugin is developed by tanvir0604. For more Advanced Usages, please check the demo page or visit the official website.