Simple Responsive Photo Gallery with jQuery - CC Photo Gallery
File Size: | 2.73 KB |
---|---|
Views Total: | 5109 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
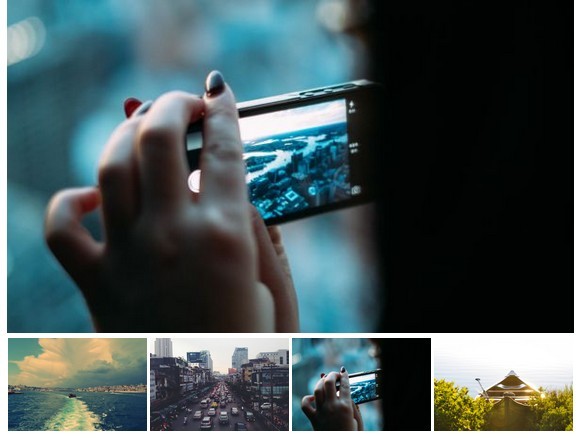
A simple jQuery implementation of an unobtrusive responsive photo gallery with a side or bottom thumbnail navigation accordion to the screen size.
How to use it:
1. The html structure for the photo gallery.
<div class="photo-gallery"> <div class="current-photo"> <img> </div> <div class="photo-list"> <ul> <li data-src="1.jpg"> <div class="thumbnail"> <img src="1.jpg"> </div> </li> <li data-src="2.jpg"> <div class="thumbnail"> <img src="2.jpg"> </div> </li> <li data-src="3.jpg"> <div class="thumbnail"> <img src="3.jpg"> </div> </li> ... </ul> </div> </div>
2. The required CSS styles.
.photo-gallery { margin: 20px auto; width: 70%; } .photo-gallery ul { padding: 0; overflow: auto; list-style: none; margin: 0; } .photo-gallery ul li { display: block; } .photo-gallery ul li .thumbnail { padding-bottom: 2px; cursor: pointer; } .photo-gallery ul li:last-child .thumbnail { padding-bottom: 0px; } .photo-gallery ul li img { width: 100%; } .photo-gallery .photo-list { margin-left: 80%; } .photo-gallery .current-photo { width: 80%; float: left; padding-right: 2px; } .photo-gallery .current-photo img { max-height: 100%; width: 100%; }
3. Make the photo gallery work well on small devices (screen size < 900px).
@media screen and (max-width: 900px) { .photo-gallery { width: 100%; padding: 0; } .photo-gallery .current-photo { width: 100%; float: none; } .photo-gallery .photo-list { width: 100%; margin-top: 5px; text-align: center; margin-left: 0; overflow: auto; } .photo-gallery ul li { float: left; width: 25%; } .photo-gallery ul li img { width: 98%; } }
4. Include jQuery library from a CDN.
<script src="//code.jquery.com/jquery-1.11.3.min.js"></script>
5. The JavaScript to enable the photo gallery.
$(function() { $('.photo-gallery ul li').click(function() { var src = $(this).data('src'); var $gallery = $(this).closest('.photo-gallery'); $gallery.find('.current-photo img').attr('src', src); }); $('.photo-gallery').each(function() { $(this).find('li')[0].click(); }); });
This awesome jQuery plugin is developed by mpayetta. For more Advanced Usages, please check the demo page or visit the official website.