Versatile & Flexible Image Viewer Plugin - viewer.js
File Size: | 3 MB |
---|---|
Views Total: | 10285 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
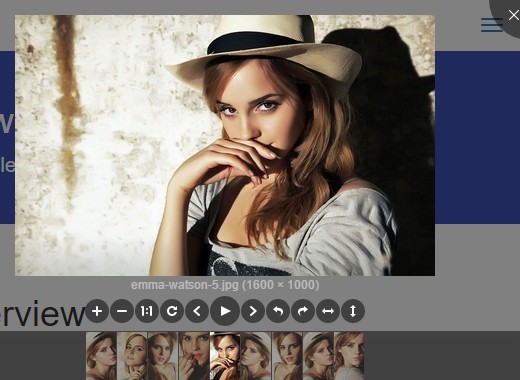
Viewer is a powerful, multi-functional, mobile-friendly and highly configurable image viewer plugin used to present your favorite images in an elegant way.
Features:
- Responsive and touch friendly.
- Keyboard support.
- Modal and Inline modes.
- Allows to zoom, rotate, flip images.
- Tons of configuration options and APIs.
Installation:
# NPM $ npm install viewerjs --save
Basic usage:
1. Include references to viewer.css and viewer.js on the web page.
<link rel="stylesheet" href="dist/viewer.min.css"> <script src="dist/viewer.min.js"></script>
2. Or import the Viewer.js as an ES module.
import Viewer from 'viewerjs';
3. To use it as a jQuery plugin, download the jQuery Wrapper and then include the following JavaScript files on the page.
<link rel="stylesheet" href="dist/viewer.min.css"> <script src="jquery.js"></script> <script src="dist/viewer.min.js"></script> <script src="dist/jquery-viewer.min.js"></script>
4. Attach the image viewer plugin to a single image.
<div> <img id="image" src="1.jpg" alt="Image Alt"> </div>
// As a Vanilla JS plugin const viewer = new Viewer(document.getElementById('image'), { // options here }); // As a jQuery plugin $('#image').viewer({ // options here });
5. Attach the image viewer plugin to a group of images.
<div> <ul id="images"> <li><img src="1.jpg" alt="Alt 1"></li> <li><img src="2.jpg" alt="Alt 2"></li> <li><img src="3.jpg" alt="Alt 3"></li> </ul> </div>
// As a Vanilla JS plugin const viewer = new Viewer(document.getElementById('image'), { // options here }); // As a jQuery plugin $('#image').viewer({ // options here });
6. Config the image viewer with the following options.
/** * Enable a modal backdrop, specify `static` for a backdrop * which doesn't close the modal on click. * @type {boolean} */ backdrop: true, /** * Show the button on the top-right of the viewer. * @type {boolean} */ button: true, /** * Show the navbar. * @type {boolean | number} */ navbar: true, /** * Specify the visibility and the content of the title. * @type {boolean | number | Function | Array} */ title: true, /** * Show the toolbar. * @type {boolean | number | Object} */ toolbar: true, /** * Custom class name(s) to add to the viewer's root element. * @type {string} */ className: '', /** * Define where to put the viewer in modal mode. * @type {string | Element} */ container: 'body', /** * Filter the images for viewing. Return true if the image is viewable. * @type {Function} */ filter: null, /** * Enable to request fullscreen when play. * @type {boolean} */ fullscreen: true, /** * Define the initial index of image for viewing. * @type {number} */ initialViewIndex: 0, /** * Enable inline mode. * @type {boolean} */ inline: false, /** * The amount of time to delay between automatically cycling an image when playing. * @type {number} */ interval: 5000, /** * Enable keyboard support. * @type {boolean} */ keyboard: true, /** * Indicate if show a loading spinner when load image or not. * @type {boolean} */ loading: true, /** * Indicate if enable loop viewing or not. * @type {boolean} */ loop: true, /** * Min width of the viewer in inline mode. * @type {number} */ minWidth: 200, /** * Min height of the viewer in inline mode. * @type {number} */ minHeight: 100, /** * Enable to move the image. * @type {boolean} */ movable: true, /** * Enable to rotate the image. * @type {boolean} */ rotatable: true, /** * Enable to scale the image. * @type {boolean} */ scalable: true, /** * Enable to zoom the image. * @type {boolean} */ zoomable: true, /** * Enable to zoom the current image by dragging on the touch screen. * @type {boolean} */ zoomOnTouch: true, /** * Enable to zoom the image by wheeling mouse. * @type {boolean} */ zoomOnWheel: true, /** * Enable to slide to the next or previous image by swiping on the touch screen. * @type {boolean} */ slideOnTouch: true, /** * Indicate if toggle the image size between its natural size * and initial size when double click on the image or not. * @type {boolean} */ toggleOnDblclick: true, /** * Show the tooltip with image ratio (percentage) when zoom in or zoom out. * @type {boolean} */ tooltip: true, /** * Enable CSS3 Transition for some special elements. * @type {boolean} */ transition: true, /** * Define the CSS `z-index` value of viewer in modal mode. * @type {number} */ zIndex: 2015, /** * Define the CSS `z-index` value of viewer in inline mode. * @type {number} */ zIndexInline: 0, /** * Define the ratio when zoom the image by wheeling mouse. * @type {number} */ zoomRatio: 0.1, /** * Define the min ratio of the image when zoom out. * @type {number} */ minZoomRatio: 0.01, /** * Define the max ratio of the image when zoom in. * @type {number} */ maxZoomRatio: 100, /** * Define where to get the original image URL for viewing. * @type {string | Function} */ url: 'src', /** * Event shortcuts. * @type {Function} */ ready: null, show: null, shown: null, hide: null, hidden: null, view: null, viewed: null, zoom: null, zoomed: null
6. API methods.
// show the viewer immediately or not viewer.show(boolean); // hide the viewer immediately or not viewer.hide(boolean); // view the second image viewer.view(1); // previous image viewer.prev([loop=false]); // next image viewer.next([loop=false]); // play the image gallery in fullscreen or not viewer.play(boolean); // stop the autoplay viewer.stop(); // enter fullscreen modal mode viewer.full(); // exit fullscreen modal mode viewer.exit(); // show the current ratio of the image with percentage. viewer.tooltip(); // toggle the image size between its natural size and initial size. viewer.toggle(); // reset the plugin. viewer.reset(); // update the plugin. viewer.reset(); // destroy the plugin viewer.destroy(); // move images viewer.move(1); viewer.move(-1, 0); // Move left viewer.move(1, 0); // Move right viewer.move(0, -1); // Move up viewer.move(0, 1); // Move down // Move the image to an absolute point. // x: The left value of the image // y: The top value of the image viewer.moveTo(x, y) // zoom in / out the image viewer.zoom(0.1); // zoom the image to a special ratio with or without tooltip viewer.zoomTo(0); // Zoom to zero size (0%) viewer.zoomTo(1); // Zoom to natural size (100%) viewer.zoomTo(.5, true); viewer.zoomTo(.5, false); // rotate the image using CSS3 transform property viewer.rotate(90); viewer.rotateTo(360); // Rotate a full round // flip the image using CSS3 transform property viewer.scale(-1); // Flip both horizontal and vertical viewer.scale(-1, 1); // Flip horizontal viewer.scale(1, -1); // Flip vertical // scale the image using CSS3 transform property viewer.scaleX(-1); // Flip horizontal viewer.scaleY(-1); // Flip vertical
7. Events handlers.
// Vanilla JavaScript image.addEventListener(Event, function () {}); // jQuery image.on(Event, function () {}); image.addEventListener('viewed', function () { // do something }); image.addEventListener('ready', function () { // do something }); image.addEventListener('show', function () { // do something }); image.addEventListener('shown', function () { // do something }); image.addEventListener('hide', function () { // do something }); image.addEventListener('hidden', function () { // do something }); image.addEventListener('view', function () { // event.detail.originalImage // event.detail.index // event.detail.image }); image.addEventListener('viewed', function () { // event.detail }); image.addEventListener('zoom', function () { // event.detail.originalEvent // event.detail.oldRatio // event.detail.ratio }); image.addEventListener('zoomed', function () { // event.detail });
Changelog:
v1.5.0 (2019-12-14)
- Force reflow element in a new way to avoid side effect
- Add a new option: slideOnTouch
- Detect if the queried image is existing when update image list
v0.6.0 (2017-10-08)
- Refactor in ES6.
- Build CSS code with PostCSS.
- Removed build event.
- Renamed built event to ready.
- Removed event namespace.
v0.5.1 (2016-03-11)
- Fixed the issue of the "button" option
- Fixed the issue of the "$.fn.viewer.setDefault" static method
v0.5.0 (2016-01-21)
- Add more available values to the "title", "toolbar" and "navbar" options.
- Support to toggle the visibility of title, toolbar and navbar between different screen widths.
- Exit fullscreen when stop playing.
- Fixed title not generated bug.
v0.4.0 (2016-01-01)
- Supports to zoom from event triggering point.
v0.3.1 (2015-12-28)
- Supports to zoom from event triggering point.
- Fix a bug of the index of viewing image.
v0.3.0 (2015-12-24)
- Add 2 new options: "view" and "viewed"
- Add 2 new events: "view" and "viewed"
- Add keyboard support: stop playing when tap the Space key
- Fix lost transition after call full method in inline mode
- Fix incorrect tooltip after switch image quickly
v0.2.0 (2015-10-18)
- Added one new method: "moveTo"
- Improved the image loading and showing.
v0.1.1 (2015-10-07)
- Fixed the issue of modal closing after zoomed in and zoomed out.
This awesome jQuery plugin is developed by fengyuanchen. For more Advanced Usages, please check the demo page or visit the official website.