jQuery Plugin For Bootstrap Modal Enhancement - eModal
File Size: | 1.42 MB |
---|---|
Views Total: | 11204 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
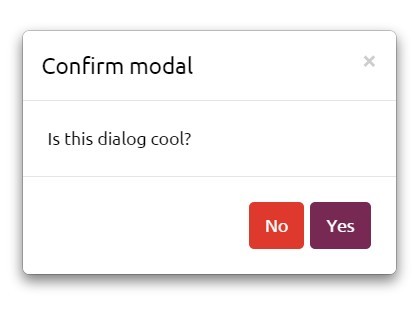
eModal is a jQuery modal popup plugin that makes it easy to display AJAX/iframe popup boxes and alert/confirm/prompt dialog boxes using Bootstrap 4/3 modal component.
How to use it:
1. Load the necessary jQuery library and Bootstrap framework in the document.
<link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/cdn/bootstrap.min.js"></script>
2. Load the jQuery eModal plugin after jQuery library.
<script src="dist/eModal.js"></script>
3. Create dialog boxes as follows:
var message = "Custom message here"; var title = "Hello World!"; // alert dialog eModal .alert(message, title) .then(function () { // do something }); // confirm dialog eModal .confirm(message, title) .then( function (/* DOM */) { // Confirm }, function (/*null*/) { // Cancel } ); // prompt dialog eModal .prompt({ size: eModal.size.sm, message: 'What\'s your name?', title: title }) .then( function (input) { // message: 'Hi ' + input + '!', title: title, imgURI: 'https://avatars0.githubusercontent.com/u/4276775?v=3&s=89' }, function () { // error }); // ajax popup var params = { buttons: [ { text: 'Close', close: true, style: 'danger' }, { text: 'New content', close: false, style: 'success', click: ajaxDemo } ], size: eModal.size.lg, title: title, url: 'https://example.com/api/' }; eModal .ajax(params) .then(function () { // do something }); // embed popup // ideal for Google Maps, Youtube Videos, etc params = { async: true, iframe: { url: 'https://www.youtube.com/embed/s8Iar_t7CW4', attributes: { 'id': 'youtube', 'allow': 'autoplay; encrypted-media', 'allowfullscreen': true } }, buttons: [ { close: true, text: 'Close' } ], onHide: hiddenModal, useBin: true, binId: 'youtube-demo' }; $.eModal .embed(params, title) .then(function(html){ console.info('Video is visible.'); });
4. Full plugin options.
var options = { // animation type animation: 'fade', // returns a Promise, that is resolved when the modal is closed async: false, // ajax options ajax: { 'dataType': false, // string 'error': false, // function 'loading': false, // boolean 'loadingHtml': LOADING, // string 'success': false, // function 'url': false, // string 'xhr': {} // object }, // unique identifier of the content saved in the recycle bin binId: false, // inline CSS styles bodyStyles: false, // custom buttons // Array, String, false, null buttons: [ {text: 'Ok', style: 'info', close: true, click: eventA }, {text: 'KO', style: 'danger', close: true, click: eventB } ], // confirm options confirm: { 'label': Object.keys(LABELS)[0], 'style': [] }, // additional CSS classes cssClass: false, // shows footer footer: true, // shows header header: true, // shows close button in the header headerClose: true, headerCloseHtml: '<button type="button" class="x close" data-dismiss="modal" aria-label="Close">' + '<span aria-hidden="true">×</span>' + '</button>', // string // height height: false, id: false, // unique ID iframe: { 'attributes': {}, 'loadingHtml': LOADING, 'url': false }, // message message: false, // Bootstrap modal options modalOptions: {}, // close by clicking the overlay overlayClose: true, // onHide function onHide: false, // position position: ['top', 'center'], // prompt options prompt: { 'autocomplete': false, // boolean 'autofocus': false, // boolean 'checkValidity': false, // boolean 'label': Object.keys(LABELS)[0], // string 'pattern': false, // string 'placeholder': false, // string 'required': true, // boolean 'style': [], // array 'type': 'text', // string 'value': false // string }, // small (sm), large (lg) and extra large (xl) size: EMPTY, // subtitle subtitle: false, // title title: 'Attention', // If set to true, $.eModal keeps the content uploaded in the body element of the modal in a recycle bin can // So that it can be recalled without a new upload from the web useBin: false, // width width: false, // custom wrapper element wrapSubtitle: '<small>', wrapTitle': '<h5>', };
5. API methods.
// close $.eModal.close // add new labels // it takes two arguments: confirm button label and reject button label $.eModal.label // return the jQuery object of the modal dialog $.eModal.modal // return the Promise to be able to perform a resolve or reject. $.eModal.defer // add a size to those provided by Bootstrap (sm, lg, xl) $.eModal.size // empty the recycle bin $.eModal.emptyBin
Changelog:
v2.1.3 (2024-04-19)
- Bugfixes
v2.1.2 (2023-09-23)
- Update
v2.1.1 (2023-09-14)
- Update
v2.1.0 (2022-05-19)
- Update
v2.0.0 (2022-05-17)
- Completely reengineered
v1.2.69 (2020-04-15)
- Fixed for Bootstrap 4.
2018-12-21
- Update
2018-12-20
- fixed for jQuery 3+.
- fix default settings size
2016-11-03
- use ajax.done instead success
2016-11-02
- Add Scroller
2016-01-19
- Set Q as option to AMD modules
2015-11-13
- Fix $modal not defined issue on first load
2015-10-14
- eModal Deferred from jQuery
This awesome jQuery plugin is developed by Reload-Lab. For more Advanced Usages, please check the demo page or visit the official website.