Android-style Analog Clock Time Picker Plugin With jQuery
File Size: | 31.4 KB |
---|---|
Views Total: | 14213 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
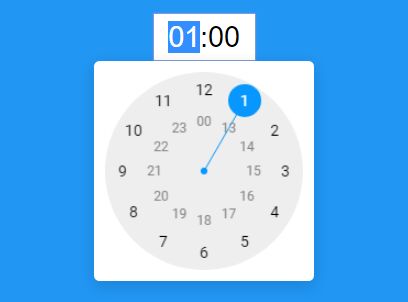
A fancy and mobile-friendly jQuery timepicker plugin which lets you create an interactive, Android inspired, Analog Clock-style time picker for your input field.
See also:
- KitKat Clock-Style Timer Picker with jQuery and CSS3 - KitKatClock
- Easy Clock Time Picker Plugin For Bootstrap - Clockface
- Fancy Clock-style Time Picker Plugin For jQuery - Timedropper
- jQuery Plugin To Select The Time Form A Clock-Style Interface
- Classic Clock Style Time Picker Plugin For jQuery
- jQuery Clock Style Time Picker Plugin For Bootstrap 3 - clockpicker
- Beautiful Animated jQuery Date Picker Plugin - datedropper
How to use it:
1. Create a normal text field and set the initial time in the value
attribute.
<input class="time" type="text" value="14:30" />
2. Download the plugin, and then insert the JavaScript file jquery-clock-timepicker.min.js
after jQuery but before the closing body tag.
<script src="//code.jquery.com/jquery.min.js"></script> <script src="jquery-clock-timepicker.min.js"></script>
3. Call the function clockTimePicker
on the input field and done.
$('.time').clockTimePicker();
4. All default settings which can be overridden with the options argument.
$('.time').clockTimePicker({ // displays the afternoon hours in the outer circle instead of the inner circle afternoonHoursInOuterCircle: false, // If you set this option to true, popup is always opened to select hours first before selecting the minutes. alwaysSelectHoursFirst: false, // auto resize autosize: false, // custom colors colors: { buttonTextColor: '#0797FF', clockFaceColor: '#EEEEEE', clockInnerCircleTextColor: '#888888', clockInnerCircleUnselectableTextColor: '#CCCCCC', clockOuterCircleTextColor: '#000000', clockOuterCircleUnselectableTextColor: '#CCCCCC', hoverCircleColor: '#DDDDDD', popupBackgroundColor: '#FFFFFF', popupHeaderBackgroundColor: '#0797FF', popupHeaderTextColor: '#FFFFFF', selectorColor: '#0797FF', selectorNumberColor: '#FFFFFF', signButtonColor: '#FFFFFF', signButtonBackgroundColor: '#0797FF' }, // Determine whether to show browser's context menu on right click contextmenu: false, // If true, the hours can be greater than 23. duration: false, // If true, the duration can be negative. // This settings only has effect if the setting duration is set to true. durationNegative: false, // font options fonts: { fontFamily: 'Arial', clockOuterCircleFontSize: 14, clockInnerCircleFontSize: 12, buttonFontSize: 20 }, // hides the unselectable number hideUnselectableNumbers: false, // i18n i18n: { okButton: 'OK', cancelButton: 'Cancel' }, // min/max times maximum: '23:59', minimum: '-23:59', // animation speed when switching modes modeSwitchSpeed: 500, // only shows clock on mobile device onlyShowClockOnMobile: false, // callbacks onAdjust: function(newVal, oldVal) { /*console.log('Value adjusted from ' + oldVal + ' to ' + newVal + '.');*/ }, onChange: function(newVal, oldVal) { /*console.log('Value changed from ' + oldVal + ' to ' + newVal + '.');*/ }, onClose: function() { }, onModeSwitch: function() { }, onOpen: function() { }, // width of the popup in the Desktop popupWidthOnDesktop: 200, // precision precision: 1, // if this option is set to true, a user cannot empty the field by hitting delete or backspace. required: false, // custom separator separator: ':', // if true, positive durations use the plus sign (+) as a prefix. useDurationPlusSign: false, // if true, the mobile phone vibrates while changing the time. vibrate: true });
5. You can also pass the options via data-OPTION
attributes as follows:
<input class="time" type="text" data-precision="2" data-minimum="09:00" data-maximum="21:00">
6. Set the time value programmatically:
$('.time').clockTimePicker('value', '10:05');
7. Close & open the time picker programmatically:
$('.time').clockTimePicker('show'); $('.time').clockTimePicker('hide');
8. Destroy the time picker:
$('.time').clockTimePicker('dispose');
9. Set & get value:
// Get value $('.time').clockTimePicker('value'); // OR $('.time').clockTimePicker('val'); // Set value $('.time').clockTimePicker('value', '08:00'); // OR $('.time').clockTimePicker('val', '08:00');
Changelog:
v2.6.4 (2023-04-28)
- Update
v2.6.3 (2022-10-27)
- New configuration setting contextmenu added.
v2.6.2 (2022-03-02)
- New configuration setting contextmenu added.
v2.6.1 (2022-02-25)
- Minified JavaScript without eval().
- Setting HTML value attribute upon time change.
- Allows to get/set value
v2.6.0 (2022-02-23)
- update
v2.5.0 (2021-10-31)
- Set the time value programmatically
- Added new methods
v2.4.0 (2021-02-12)
- Position popup "fixed" instead of "absolute" to prevent popup from cut-off in containers with overflow: hidden.
- Slow down scroll wheel event so that the clock timepicker doesn't spin uncontrollable when using touchpad.
- Update to jQuery 3.5
v2.3.5 (2020-03-11)
- Bugfixed.
- Blur input element on enter.
v2.3.4 (2020-02-19)
- Method onInputElementKeyUp completely refactored to simplify and to solve issue.
- Arrow keys and +/- sign behavior refactored. No wheelspin anymore, stop at minimum and maximum values.
- Bugfix for bluring input element when switching from one clock timepicker element to another one.
v2.3.3 (2020-02-18)
- More bugs fixed
v2.3.2 (2019-09-02)
- Using div and spans instead of input element on mobile phones to prevent context menu and cursors to show up.
- Rounding problem on initialization solved when using option precision.
- New option alwaysSelectHoursFirst inserted.
v2.3.1 (2018-09-04)
- Bugfix for entering durations with keyboard when useDurationPlusSign is set to true
- Removed unwanted Console.log
v2.3.0 (2018-08-09)
- Bugfix.
- Shows unselectable numbers when using minimum and maximum options.
- Hide unselectable numbers completely by using hideUnselectableNumbers option.
- Configure text colors for unselectable numbers with new options clockInnerCircleUnselectableTextColor and clockOuterCircleUnselectableTextColor.
- Use of data attributes to configure ClockTimePicker element.
- Bugfix for wrong hovering when using afternoonHoursInOuterCircle.
v2.2.5 (2018-03-21)
- Fixed a converting bug when switching between minus and plus sign.
- Option useDurationPlusSign implemented.
2018-02-08
- v2.2.2: Perfectionated to select hour/minute part with mouse click; Parse number settings as integer to prevent errors in calculations in case that a string is passed.
2018-02-07
- v2.2.0: Now this plugin works in both portrait and landscape mode on the mobile phone.
2018-02-06
- v2.1.10: Bluring issues fixed.
2017-12-09
- Calling callback events in the element's context.
2017-11-22
- v2.1.1
2017-09-13
- Totally revised. Event management changed so that onchange listener works as expected.
2017-09-11
- Event handling for onchange adjusted and new setting 'precision' added.
2017-08-19
- Added 'duration' and 'onlyShowClockOnMobile' options
2017-06-10
- Change event implemented to refresh the clock.
2017-06-09
- Fixed issues with onchange event on HTML element.
2017-05-22
- Keyboard input improved, Events added to config and config revised
This awesome jQuery plugin is developed by loebi-ch. For more Advanced Usages, please check the demo page or visit the official website.