Touch-enabled Book/Page Flip Effect In jQuery
File Size: | 7.98 KB |
---|---|
Views Total: | 13153 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
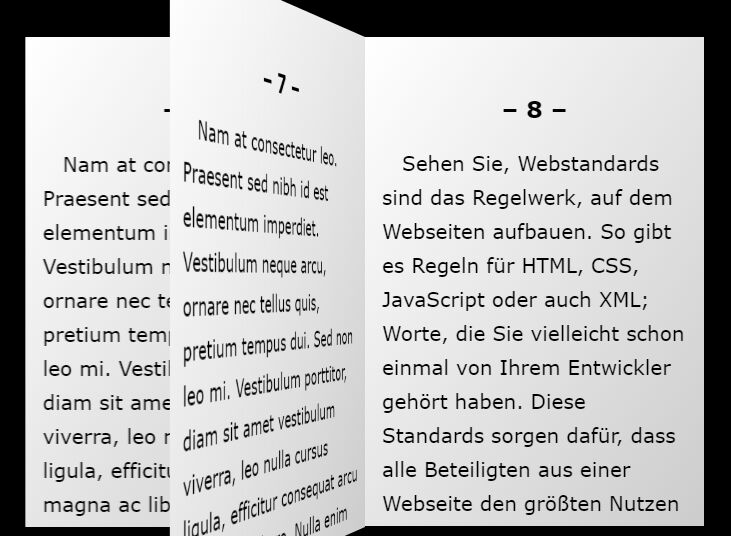
A tiny jQuery script to create a mobile-friendly 3D page flip effect when you switch between page contents just like turning a real book or magazine.
Based on CSS perspective, transition, and transform properties. Supports swipe left & swipe right events based on hammer.js library.
How to use it:
1. Split your web content into several pages containing front/back content as follows:
<div class="scene"> <article class="book"> <section class="page active"> <div class="front"> <h1>Book/Page Flip Effect</h1> <p>A tiny jQuery script to create a mobile-friendly 3D page flip effect.</p> </div> <div class="back"> <h1>– 1 –</h1> <p>Page 1</p> </div> </section> <section class="page"> <div class="front"> <h1>– 2 –</h1> <p>Page 2 </p> </div> <div class="back"> <h1>– 3 –</h1> <p>Page 3</p> </div> </section> <section class="page"> <div class="front"> <h1>– 4 –</h1> <p>Page 4</p> </div> <div class="back"> <h1>– 5 –</h1> <p>Page 5</p> </div> </section> </article> </div>
2. The primary CSS/CSS3 styles for the page flip effect.
.scene { width: 45%; height: 90%; margin: 5% 5% 5% 50%; perspective: 1000px; } .book { position: relative; width: 100%; height: 100%; transform-style: preserve-3d; } .page { cursor: pointer; position: absolute; color: black; width: 100%; height: 100%; transition: 1.5s transform; transform-style: preserve-3d; transform-origin: left center; } .front, .back { position: absolute; width: 100%; height: 100%; padding: 10% 5% 5%; box-sizing: border-box; backface-visibility: hidden; background: -webkit-gradient(linear, 0% 0%, 100% 100%, from(#FFFFFF), to(#CCCCCC)); background: linear-gradient(to bottom right, #fff, #ccc); } .back { transform: rotateY(180deg); } .page.active { z-index: 1; } .page.flipped { transform: rotateY(-180deg); } .page.flipped:last-of-type { z-index: 1; } @media only screen and (min-device-width : 768px) and (max-device-width : 1024px) and (orientation : portrait) { .scene { width: 90%; height: 90%; margin: 5%; } }
3. Load the needed jQuery and hammer.js libraries in the document.
<script src="/path/to/jquery.min.js"></script> <script src="/path/to/hammer.min.js"></script> <script src="/path/to/jquery.hammer.min.js"></script>
4. The main script to enable the page flip effect.
var currentPage = 0; $('.book') .on('click', '.active', nextPage) .on('click', '.flipped', prevPage); $('.book').hammer().on("swipeleft", nextPage); $('.book').hammer().on("swiperight", prevPage); function prevPage() { $('.flipped') .last() .removeClass('flipped') .addClass('active') .siblings('.page') .removeClass('active'); } function nextPage() { $('.active') .removeClass('active') .addClass('flipped') .next('.page') .addClass('active') .siblings(); }
This awesome jQuery plugin is developed by Timo Hausmann. For more Advanced Usages, please check the demo page or visit the official website.