Create A Rotatable & Scalable Photo Grid With Canvas Table
File Size: | 117 KB |
---|---|
Views Total: | 3262 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
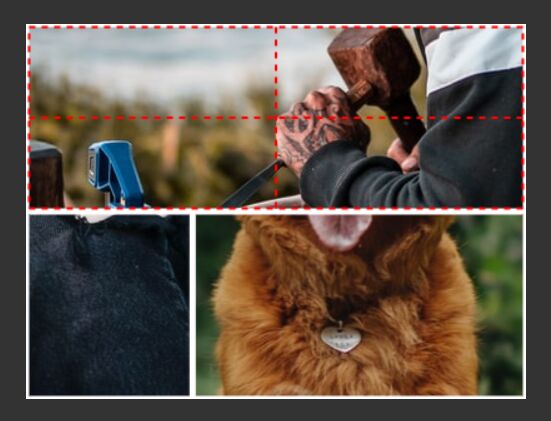
Canvas Table is a simple jQuery based image editing tool to dynamically generate a customizable photo grid using HTML5 canvas.
Click/tap on the photo grid to rotate, scale, and/or pan around an image and then click the Generate button to render a new photo grid that is downloadable as a PNG image (base64 encoded).
How to use it:
1. Create an empty <canvas />
element on which the plugin renders the image editing area.
<canvas id="canvas"></canvas>
2. Create the Scale and Rotate slider controls.
<label for="scaleRange">Scale</label> <input id="scaleRange" type="range" disabled="disabled" min="0.1" max="2" step="0.1" value="1" /> <label for="rotateRange">Rotate</label> <input id="rotateRange" type="range" disabled="disabled" min="-360" max="360" step="1" value="0" />
3. Create a Generate button to render a photo grid after images have been edited.
<button id="createButton" type="button">Create Table</button>
4. Create an empty <img />
to hold the photo grid image.
<button id="createButton" type="button">Create Table</button>
5. Download and insert the JavaScript canvas-table.js
after loading the latest jQuery library.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/canvas-table.js"></script>
6. Initialize the plugin on the <canvas />
element and define the paths to the images as follows:
const $canvasTable = $("#canvas").canvasTable({ // css grid template areas templateAreas: ["photo1 photo1 photo1", "photo2 photo3 photo3"], images: [ { area: "photo1", source: "1.jpg" }, { area: "photo2", source: "2.jpg" }, { area: "photo3", source: "3.jpg" } ], onSelected(selectedArea) { $("#scaleRange").attr("disabled", false); $("#rotateRange").attr("disabled", false); $("#scaleRange").val(selectedArea.image.scale); $("#rotateRange").val(selectedArea.image.rotate); } });
7. Enable the controls & generate button.
$("#scaleRange").on("input", (event) => { $canvasTable.changeScale(event.target.value); }); $("#rotateRange").on("input", (event) => { $canvasTable.changeRotate(event.target.value); }); $("#createButton").click(() => { $canvasTable.setDefault(); const canvasSource = $canvasTable.createPhoto(); $("#previewTable").attr("src", canvasSource); });
8. Customize the appearance of the canvas table.
const $canvasTable = $("#canvas").canvasTable({ width: 500, height: 500, templateAreas: [], images: [], gap: 1, fillStyle: "#fff", strokeStyle: "red", strokeDash: 0, strokeWidth: 3, frameImage: "", frameWidth: 0, showAlignmentLines: true, onSelected: () => null });
9. API methods:
// scale the image $canvasTable.changeScale(string | number ); // rotate the image $canvasTable.changeRotate(string | number ); // generate a photo grid $canvasTable.createPhoto(type: 'image/png');
Changelog:
2020-06-22
- Changed preview
2020-06-22
- Changed preview
This awesome jQuery plugin is developed by enessefak. For more Advanced Usages, please check the demo page or visit the official website.