jQuery Plugin For Responsive Touch-friendly Lightbox - imageLightbox
File Size: | 42.9 KB |
---|---|
Views Total: | 20688 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
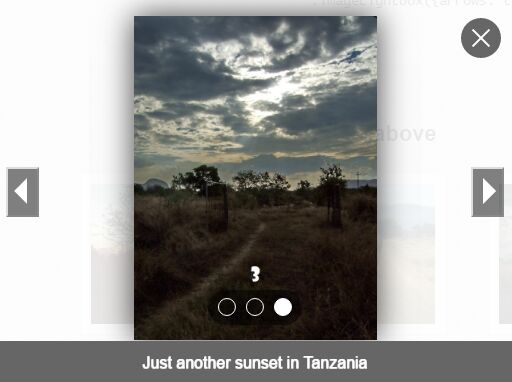
A simple, flexible, responsive, touch-friendly jQuery image lightbox/gallery plugin used for showcasing your images in an elegant way.
More features:
- Presents your images in a configurable lightbox popup.
- Clicking on the current image will goto the next.
- Automatically preloads the next image.
- Image captions.
- Close & navigation buttons.
- Configurable background overlay.
- Keyboard interactions.
- Works perfectly on both desktop and mobile.
Installation:
# Yarn $ yarn add imagelightbox # NPM $ npm install imagelightbox
Basic usage:
1. Load the jQuery imageLightbox plugin's files after jQuery as usual.
<link rel="stylesheet" href="dist/imagelightbox.css" /> <script src="//code.jquery.com/jquery.min.js"></script> <script src="dist/imagelightbox.umd.cjs"></script>
2. Insert your thumbnails into your webpage and link them them to the original images as displayed below:
<a href="1.jpg" data-imagelightbox="demo"> <img src="thumb-1.jpg"> </a> <a href="2.jpg" data-imagelightbox="demo"> <img src="thumb-1.jpg"> </a> <a href="3.jpg" data-imagelightbox="demo"> <img src="thumb-3.jpg"> </a> ...
3. If you want to display custom captions at the bottom of the images.
<a href="1.jpg" data-imagelightbox="demo" data-ilb2-caption="Caption 1"> <img src="thumb-1.jpg"> </a> <a href="2.jpg" data-imagelightbox="demo" data-ilb2-caption="Caption 2"> <img src="thumb-1.jpg"> </a> <a href="3.jpg" data-imagelightbox="demo" data-ilb2-caption="Caption 3"> <img src="thumb-3.jpg"> </a> ...
4. You might also need to embed videos into the lightbox.
<a data-ilb2-video='{"controls":"controls", "autoplay":"autoplay", "sources":[{"src":"images/video.m4v", "type":"video/mp4"}], "width": 1920, "height": 1080}' data-imagelightbox="x"> <img src="images/video-thumb.jpg"> </a>
5. Active the image lightbox plugin with default settings.
$('a[data-imagelightbox="demo"]').imageLightbox();
6. Add new images to the lightbox.
var gallery = $( selector ).imageLightbox(); var image = $( '<img />' ); gallery.addToImageLightbox( image );
7. All default configuration options.
$('a[data-imagelightbox="demo"]').imageLightbox({ selector: 'a[data-imagelightbox]', allowedTypes: 'png|jpg|jpeg|gif', animationSpeed: 250, activity: false, // activity indicator arrows: false, // navigation arrows button: false, // close button caption: false, enableKeyboard: true, history: false, fullscreen: false, gutter: 10, // percentage of client height offsetY: 0, // percentage of gutter navigation: false, overlay: false, preloadNext: true, quitOnEnd: false, quitOnImgClick: false, quitOnDocClick: true, quitOnEscKey: true });
8. Event handlers.
$(document) .on("start.ilb2", function (_, e) { // on init }) .on("next.ilb2", function (_, e) { // next image }) .on("previous.ilb2", function (_, e) { // previous image }) .on("quit.ilb2", function () { //after you quit the lightbox });
Changelog:
v2.1.0 (2024-03-28)
- Fixed typings for imageLightbox and startImageLightbox incorrectly having their params required
- Bumped minimum browser support to mostly follow browserslist recommendations
- Removed references to the (previously unused) options id, selector and offsetY
- Renamed most classes and IDs, all have the ilb- prefix now
- Fixed an issue with history for lightboxes without IDs
- Made video sizes optional
- Refactored the whole codebase, making the package smaller, enabling rapid navigation and making the code more maintainable
v1.2.0 (2022-08-10)
- Added TypeScript type declarations for imagelightbox
- Added unminified output to dist in addition to the minified files
- Changed: Clicking in the center of the image navigates to the next one
- Changed: The lightbox buttons are plain <div>s now
- Fixed an issue where rapidly navigating would get the lightbox in a broken state
v1.1.0 (2020-06-15)
- Added the option to explicitly specify video dimensions
v1.1.0 (2020-06-15)
- Added the option to explicitly specify video dimensions
v1.0.2 (2020-02-08)
- Bugfix
v1.0.2 (2019-06-27)
- Bugfix
v1.0.1 (2019-06-20)
- Fixed a bug with videos not being preloaded if they were added to an already initialized lightbox
v1.0.0 (2019-06-04)
- Major release.
v0.14.0 (2019-04-03)
- Removed origTargets
- Code refacting
- Update fullscreen code
- Bugfixes
- Adding onclick swipe to next only if not a video
v0.13.0 (2018-11-28)
- Added Expose openHistory function
- Fixed History and dynamic adding don't work together
- Removed origTargets
v0.12.0 (2018-10-12)
- Fixed All videos playing simultaneously
- Fixed error with history sometimes deleting path
- Use correct html element for close-button
- Updated style
v0.11.0 (2018-06-30)
- Added video functionality
v0.10.0 (2018-06-30)
- Added history functionality
- Added checks for multiple sets - fixes a bug
2018-05-17
- v0.9.1: Increased jquery version range
2018-05-08
- v0.9.0: Make allowedTypes work again, use empty string to allow any filetype
2017-11-04
- v0.8.1: Reverse transition direction to be more intuitive
2017-10-12
- v0.8.0: update
2017-09-28
- v0.7.9: fix the positioning; add better style name for opened lightbox
2017-09-18
- v0.7.8: Update call of imagelightbox to achieve deep links
2017-07-20
- v0.7.7: These edits make imagelightbox compatible with webpack out of the box
2017-06-20
- v0.7.6: Move "loaded" event to end of loading function where it actually belongs.
2017-06-15
- v0.7.5: clean up
This awesome jQuery plugin is developed by marekdedic. For more Advanced Usages, please check the demo page or visit the official website.