Create Conversation Chats With jQuery - ConvoJs
File Size: | 170 KB |
---|---|
Views Total: | 7765 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
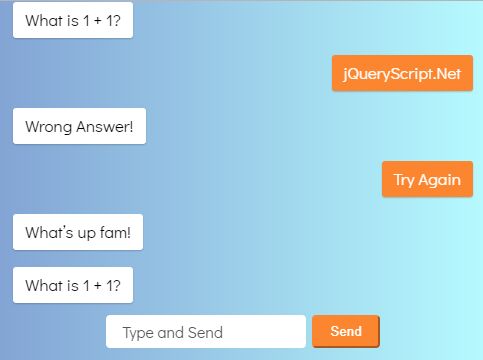
ConvoJs is a fancy jQuery chat bot plugin which lets you create interactive, dynamic, mobile-friendly conversation chats on the web app.
Basic usage:
1. Import jQuery JavaScript library and the jQuery ConvoJs plugin's files into the html document.
<link href="css/convo.css" rel="stylesheet"> <script src="https://code.jquery.com/jquery-3.2.1.min.js"></script> <script src="js/convo.js"></script>
2. Create the HTML for the chat UI.
<div class="top-bar"></div> <div class="header-tools"> <a href="javascript:void(0)"> <img src="logo.png" alt="Your Logo Here" id="logo"/> </a> </div> <div class="convo__wrapper"> <ul class="bubble__wrapper"> </ul> </div> <div class="cui__response"> </div> <div class="background"> </div>
3. Prepare your own chat messages, choices, questions for the chat bot.
var chatz = [{ "path": "intro", "messages": [{ "text": "What’s up fam!", "author": "ConvoJs" }, { "text": "What is 1 + 1?", "author": "ConvoJs" } ], "choices": [ { "path": "block1", "text": "Meh", "type": "input" }, { "path": "block1", "text": "Meh", "type": "button", "expected": "2", "pathTrue": "block1-correct", "pathFalse": "block1-wrong" } ] }, { "path": "block1-correct", "messages": [{ "text": "You got it, good job", "author": "ConvoJs" } ], "choices": [{ "path": "block2", "text": "Continue", "write": false } ] }, { "path": "block1-wrong", "messages": [{ "text": "Wrong Answer!", "author": "ConvoJs" } ], "choices": [{ "path": "intro", "text": "Try Again", "write": false } ] },{ "path": "block2", "messages": [ { "text": "Now, what is 2 + 3?", "author": "ConvoJs" } ], "choices": [ { "path": "block2", "text": "Meh", "type": "input" }, { "path": "block2", "text": "Meh", "type": "button", "expected": "5", "pathTrue": "block2-correct", "pathFalse": "block2-wrong" } ] }, { "path": "block2-correct", "messages": [{ "text": "Fantastic! ", "author": "ConvoJs" } ], "choices": [{ "path": "block__", "text": "That's all for now!", "write": false } ] }, { "path": "block2-wrong", "messages": [{ "text": "Wrong Answer!", "author": "ConvoJs" } ], "choices": [{ "path": "intro", "text": "Try Again", "write": false } ] }];
4. Initialize the chat bot.
$(".bubble__wrapper").convo({ data: chatz });
5. Update for response input.
$("[contenteditable]").focusout(function () { var element = $(this); if (!element.text().trim().length) { element.empty(); } });
6. All possible plugin options to customize the chat bot.
$(".bubble__wrapper").convo({ headerTopColor: null, logo: null, pageColor: null, inputCharLimit: 65, element: $(".bubble__wrapper") });
This awesome jQuery plugin is developed by chrismuiruriz. For more Advanced Usages, please check the demo page or visit the official website.