Advanced HTML5 Video Player - jQuery aksVideoPlayer.js
File Size: | 176 MB |
---|---|
Views Total: | 4993 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
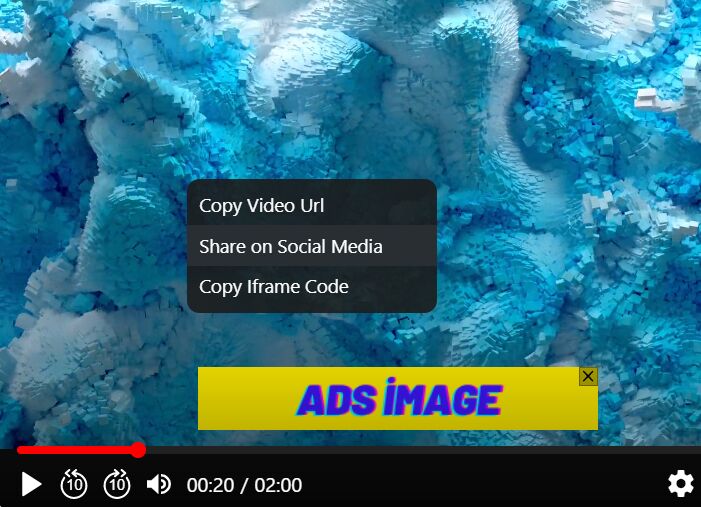
aksVideoPlayer.js is a full-featured, highly customizable HTML5 video player built on top of jQuery.
Features:
- Easy to implement.
- Custom video poster.
- Allows the user to choose the video quality.
- Allows the user to adjust the playback speed.
- Picture in picture.
- Fullscreen mode.
- Forward and Rewind buttons.
- Video captions and subtitles.
- Custom context menu.
- Allows you to insert your own ads to the video.
How to use it:
1. Load the aksVideoPlayer.js jQuery plugin's files in the document.
<link rel="stylesheet" href="/path/to/dist/aksVideoPlayer.min.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/dist/aksVideoPlayer.min.js"></script>
2. Load the Google IMA3 SDK if you'd like to integrate ads into your HTML5 videos.
<script src="https://imasdk.googleapis.com/js/sdkloader/ima3.js"></script>
3. Create a container to hold the video player.
<div id="video"></div>
4. Initialize the plugin and specify the path to your HTML5 video.
$("#video").aksVideoPlayer({ file: [ { file: "1080.mp4", label: "1080p" }, { file: "720.mp4", label: "720p" }, { file: "540.mp4", label: "540p" }, { file: "360.mp4", label: "360p" }, { file: "240.mp4", label: "240p" } ] });
5. Set the width and height the video player.
$("#video").aksVideoPlayer({ width: 640, height: 360, });
6. Specify the path to the video poster.
$("#video").aksVideoPlayer({ poster: "poster.webp" });
7. Add captions and subtitles to the video.
$("#video").aksVideoPlayer({ captions: [ { file: "subtitle.en.vtt", label: "English", kind: "captions", srclang: "en" }, { file: "subtitle.fr.vtt", label: "Français", kind: "captions", srclang: "fr" } ] });
8. Insert custom links to the context menu.
$("#video").aksVideoPlayer({ contextMenu: [ { type: "urlCopy", label: "Copy Video Url", url: "https://www. jqueryscript.net/" }, { type: "socialmedia", label: "Share on Social Media", socials: [ { label: "Facebook", url: "", colorBg: "#0066ff", color: "white", icon: '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 10 20"><defs/><path d="M8.174 3.32H10V.14A23.66 23.66 0 007.34 0C4.709 0 2.906 1.656 2.906 4.7v2.8H0v3.555h2.905V20h3.56v-8.945h2.789L9.697 7.5H6.466V5.05c0-1.027.276-1.73 1.708-1.73z" fill-rule="evenodd"/></svg>' }, { label: "Twitter", url: "", colorBg: "#0089ff", color: "white", icon: '<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 20 16"><defs/><path d="M17.944 3.987c.013.175.013.35.013.526C17.957 9.85 13.833 16 6.294 16c-2.322 0-4.48-.662-6.294-1.813.33.038.647.05.99.05 1.916 0 3.68-.637 5.089-1.725-1.802-.037-3.313-1.2-3.833-2.8.254.038.508.063.774.063.368 0 .736-.05 1.079-.137-1.878-.376-3.287-2-3.287-3.963v-.05c.546.3 1.18.488 1.853.512A4.02 4.02 0 01.838 2.775c0-.75.203-1.438.558-2.038a11.71 11.71 0 008.452 4.225 4.493 4.493 0 01-.102-.924c0-2.226 1.828-4.038 4.1-4.038 1.18 0 2.245.487 2.994 1.275A8.145 8.145 0 0019.442.3a4.038 4.038 0 01-1.802 2.225A8.316 8.316 0 0020 1.9a8.74 8.74 0 01-2.056 2.087z" fill-rule="evenodd"/></svg>' } ] }, { type: "iframe", label: "Copy Iframe Code", iframe: "<iframe></iframe>" } ] });
9. Insert custom ads to the video.
$("#video").aksVideoPlayer({ ads: [ { type: "google", url: 'https://pubads.g.doubleclick.net/gampad/ads?sz=640x480&' + 'iu=/124319096/external/single_ad_samples&ciu_szs=300x250&impl=s&gdfp_req=1&'+ 'env=vp&output=vast&unviewed_position_start=1&cust_params=' + 'deployment%3Ddevsite%26sample_ct%3Dskippablelinear&correlator=' }, { type: "image", src: "ads.png", width: 320, height: 50, link: "https://www.jqueryscript.net/", time: "00:20" }, { type: "video", src: "videoads.mp4", link: "https://www.jqueryscript.net/", time: "00:35", adstimer: "6" } ], });
10. Customize the video controls.
$("#video").aksVideoPlayer({ rewind: true, rewindValue: 10, forward: false, forwardValue: 10, preview: true, previewWidth: 140, previewHeight: 95, controller: true, autoplay: false, muted: true, volume: 1, loop: false, playbackRate: ["0.25", "0.5", "0.75", "1", "1.25", "1.5", "1.75", "2"], pictureinpicture: true, });
11. Customize & localize the labels.
$("#video").aksVideoPlayer({ playbackRateLabel: "Playing Speed", captionsLabel: "Subtitles", sourcesLabel: "Quality", playLabel: "Play", pauseLabel: "Pause", rewindLabel: "Rewind %s Seconds", forwardLabel: "Forward %s Seconds", settingsLabel: "Settings", fullScreenLabel: "Fullscreen", exitFullScreenLabel: "Exit Fullscreen", adsSkipLabel: "Skip Ad", closeLabel: "Close", pictureinpictureLabel: "Picture in Picture", });
This awesome jQuery plugin is developed by Ahmetaksungur. For more Advanced Usages, please check the demo page or visit the official website.