Online Ping Tool With jQuery Ping And Terminal Plugins
File Size: | 4.83 KB |
---|---|
Views Total: | 3286 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
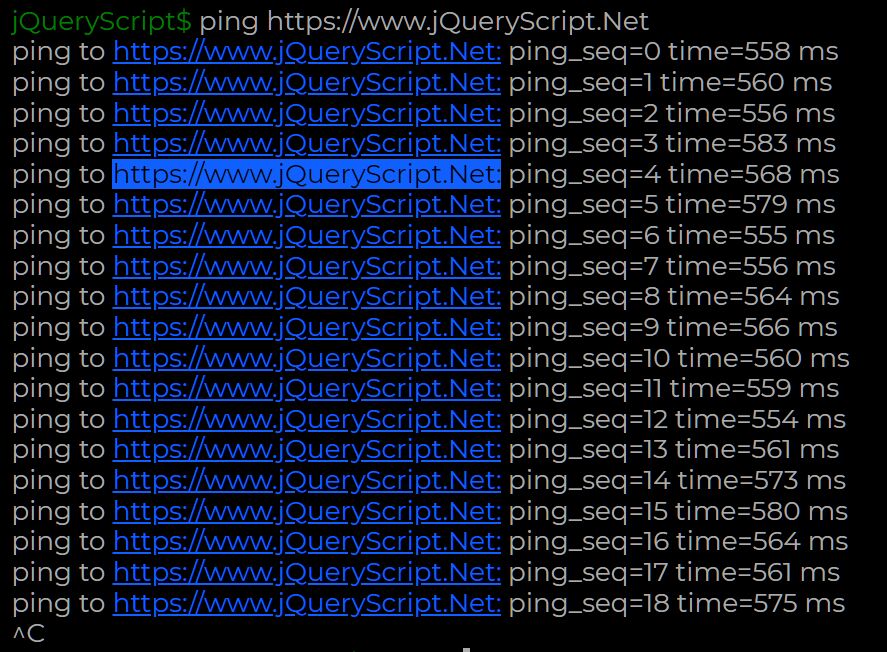
A JavaScript solution that makes use of jQuery Terminal.js and Ping.js plugins to create a client-side online ping tool (web app) similar to the Windows ping command.
To ping a website, type "ping" along with the URL or IP address you want to ping, and then hit Enter in the terminal. Hit Control+C to break the ping command.
The tool pings every second. If the response time is over 1.5 s, the ping times out.
How to use it:
1. Load the necessary jQuery, jQuery Terminal.js, and Ping.js in the document.
<!-- jQuery --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Terminal Plugin --> <script src="/path/to/cdn/jquery.terminal.min.js"></script> <link rel="stylesheet" href="/path/to/cdn/jquery.terminal.min.css"/> <!-- Ping.js Library --> <script src="/path/to/cdn/ping.js"></script>
2. Create a container to hold the online ping terminal.
<div id="terminal"></div>
3. The main JavaScript (jQueryscript) to activate the online ping tool.
function pinger() { var pinging, ad, timer, suc, total, loc, holder; var times = []; $('#terminal').terminal(function(command, term) { var cmd = $.terminal.parse_command(command); var address = cmd.args[0]; if (cmd.name == 'ping' && address != undefined) { times = []; loc = address; if(address.substring(0,7) != 'http://' && address.substring(0,8) != 'https://') { ad = "http://" + address; } else{ ad = address; } term.echo("PINGING " + address); var i = 0; total = 0; suc = 0; var output = ""; var p = new Ping(); pinging = true; (function loop() { p.ping(ad, function(err, data) { // Also display error if err is returned. if (err || data >= 1500) { output = "Request timeout for ping_seq " + i; } else{ holder = data; output = "Transmission to " + address + ": ping_seq=" + i + " time=" + data + " ms"; } }); if(output) { term.echo(output); total = i + 1; i++; if(output.substring(0,1) == 'T') { suc++; times.push(holder); } } term.set_prompt(""); p = new Ping(); timer = setTimeout(loop, 1000); })(); } else if (cmd.name == 'ping' && address == undefined) { term.echo("ping: no address to ping"); } else if (cmd.name){ term.echo(cmd.name + ": command not found"); } }, { greetings: 'Connection to https://www.yourwebsite.com [[;green;]success]\n'+ 'Login time: ' + new Date() + '\n----------------------------\nThe program pings every second\nIf the response time is over 1.5 s, the ping times out\nStart by typing the ping command and the address as the argument \nStop the pinging by hitting CTRL and C at the same time\n----------------------------', width: 600, height: 350, prompt: "[[;white;]yourwebsite.com:projects visitor$ ]", keydown: function(e, term) { if (pinging) { if (e.which == 67 && e.ctrlKey) { // CTRL+c term.echo("^C"); clearTimeout(timer); pinging = false; term.echo("--- " + loc + " ping statistics ---"); var ploss = (total - suc) * 100.0 / total; var round = ploss.toFixed(1); term.echo(total + " pings transmitted, " + suc + " successful pings, " + round + "% loss"); var min = Math.min(...times); var max = Math.max(...times); var avg = (times.reduce((a,b) => a + b, 0) / times.length).toFixed(1); var sqrs = times.map(function(value){ var diff = value - avg; var sqr = diff * diff; return sqr; }); var sqrAvg = sqrs.reduce((a,b) => a + b, 0) / sqrs.length; var std = (Math.sqrt(sqrAvg)).toFixed(1); term.echo("Time data: min/max/avg/stddev = " + min + "/" + max + "/" + avg + "/" + std); term.set_prompt("[[;white;]yourwebsite.com:projects visitor$ ]"); } return false; } } }); }
4. Run the online ping tool.
pinger();
This awesome jQuery plugin is developed by eagleyuan21. For more Advanced Usages, please check the demo page or visit the official website.