jQuery Plugin For Pagination On Dynamic Data - jqPaginator.js
File Size: | 7.81 KB |
---|---|
Views Total: | 8042 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
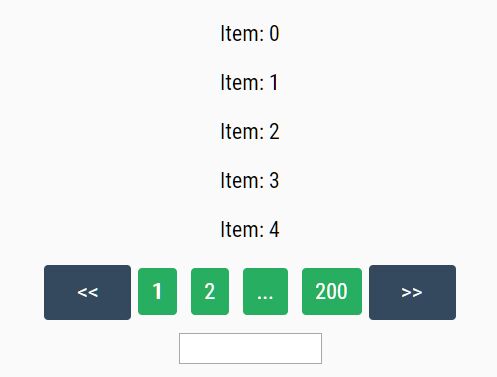
A small yet fully configurable paginator plugin to create pagination controls for dynamic data defined in a JavaScript array or a custom function.
How to use it:
1. The plugin requires the latest jQuery library (slim build is recommended) to work.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
2. Load the Stylesheet jqpaginator.css
for the basic styling of the paginator.
<link rel="stylesheet" href="./css/jqpaginator.css" />
3. Load the JavaScript jqpaginator.js
after jQuery.
<script src="./js/jqpaginator.js"></script>
4. Create an element to hold the pagination controls.
<div id="example"></div>
5. Define your data and specify the total number of records as follows:
function createData() { var arr = []; var i = 0; while (i < 1000) { arr.push("Item: " + i.toString()); i++; } return arr; } function dataFunc(done) { var data = []; while (data.length < itemsPerPage) { var num = Math.floor(Math.random() * 100); data.push(num); } var handler = function() { done(data, 100); }; setTimeout(handler, 1000); }
6. Determine how to render the data using the renderer
.
function renderer(data) { $('#content').empty(); $.each(data, function(i, v) { $('#content').append("<p>" + v + "</p>"); }); };
7. Initialize the plugin to generate a pagination on the webpage.
$('#example').jqpaginator({ data: createData(), render: renderer });
8. Customize the paginator by overriding the default options as follows:
$('#example').jqpaginator({ // items to show per page itemsPerPage: 10, // text for next/prev buttons buttonText: ["<<", ">>"], // shows pagination links showNumbers: true, // shows next/prev buttons showButtons: true, // shows an input which allows you to directly go to specific page showInput: true, // the margin between pagination links numberMargin: 2 });
9. API methods.
// get the current page num $('#example').jqpaginator('getPage'); // reload data $('#example').jqpaginator('reload'); // go to a specific page $('#example').jqpaginator('goto', 5); // destroy the paginator $('#example').jqpaginator('destroy');
10. Default CSS classes which can be used to customize the pagination controls using your own CSS.
parentcls: 'jqpaginator', wrapcls: 'jqp-wrap', pageListcls: 'jqp-pages', pageItemcls: 'jqp-page', inputcls: 'jqp-input', prevcls: 'jqp-prev', nextcls: 'jqp-next', activecls: 'jqp-active', disabledcls: 'jqp-disable',
This awesome jQuery plugin is developed by ZWhit196. For more Advanced Usages, please check the demo page or visit the official website.