Pick Colors From A Predefined Palette - jQuery choose-color.js
File Size: | 6.49 KB |
---|---|
Views Total: | 4415 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
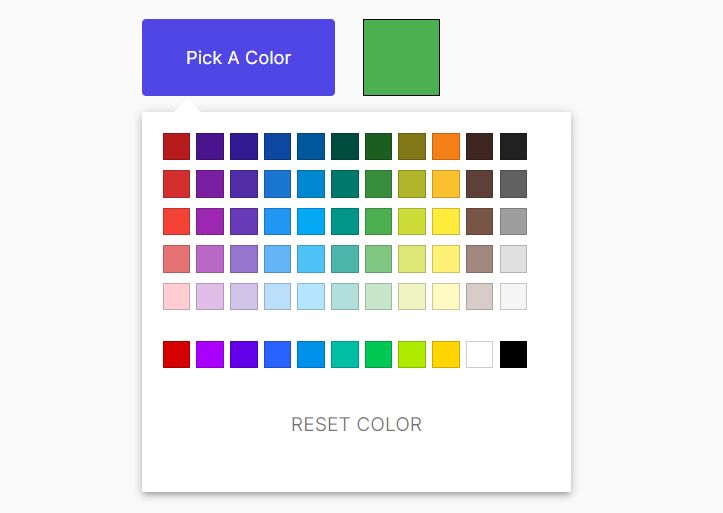
choose-color.js is a jQuery based color picker component that enables you to pick colors from a restricted palette defined in an HTML template.
How to use it:
1. Create the HTML for the color picker.
<!-- Trigger Button --> <button id="chooseColor">Pick A Color</button> <!-- Color Preview --> <div class="colorDisplay"></div> <!-- Hidden input to store the selected color value --> <input class="colorValue" type="hidden" name="">
2. Include the choose-color.js plugin's files on the page.
<link rel="stylesheet" href="choose-color.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="choose-color.js"></script>
3. Initialize the color picker and apply the selected value to the hidden input and the background color of the colorDisplay
element.
$(document).ready(() => { $.support.cors = true; const $button = $('#chooseColor') const $colorValue = $('.colorValue') const $colorDisplay = $('.colorDisplay') $($button).sbxColorChoice({ selecionarCor: (color) => { $($colorValue).val(color) $colorDisplay.css('background-color', color) console.log($($colorValue).val()); }, }) })
4. Override the default colors in the choose-color.html
to create your own palette.
<div class="co-color-list co-shadow-3dp" style="display:none" tabindex="-1"> <ul class="color-pallet"> <li class="co-hue-1" data-color="#B71C1C" style="background-color:#B71C1C"></li> <li class="co-hue-1" data-color="#4A148C" style="background-color:#4A148C"></li> <li class="co-hue-1" data-color="#311B92" style="background-color:#311B92"></li> <li class="co-hue-1" data-color="#0D47A1" style="background-color:#0D47A1"></li> <li class="co-hue-1" data-color="#01579B" style="background-color:#01579B"></li> <li class="co-hue-1" data-color="#004D40" style="background-color:#004D40"></li> <li class="co-hue-1" data-color="#1B5E20" style="background-color:#1B5E20"></li> <li class="co-hue-1" data-color="#827717" style="background-color:#827717"></li> <li class="co-hue-1" data-color="#F57F17" style="background-color:#F57F17"></li> <li class="co-hue-1" data-color="#3E2723" style="background-color:#3E2723"></li> <li class="co-hue-1" data-color="#212121" style="background-color:#212121"></li> ... <ul class="color-reset"> <li data-color="" class="co-button-flat co-width-100-percentage co-font-color-black-54"> <span class="reset-color-button">Reset color</span> </li> </ul> </div>
4. Set the color to apply when the Reset button is clicked.
$($button).sbxColorChoice({ selecionarCor: (color) => { $($colorValue).val(color) $colorDisplay.css('background-color', color) console.log($($colorValue).val()); }, reseteCor: '#FFFFFF', })
5. Customize the text to display in the Reset button.
$($button).sbxColorChoice({ selecionarCor: (color) => { $($colorValue).val(color) $colorDisplay.css('background-color', color) console.log($($colorValue).val()); }, textResetColorButton: 'Custom text', })
6. Ignore specific colors if needed.
$($button).sbxColorChoice({ selecionarCor: (color) => { $($colorValue).val(color) $colorDisplay.css('background-color', color) console.log($($colorValue).val()); }, removePallet: '.co-hue-1, .co-hue-2, .co-hue-3, .co-hue-4' })
This awesome jQuery plugin is developed by victormaestri. For more Advanced Usages, please check the demo page or visit the official website.