Get the Unicode & Name Of Pressed Key Using jQuery
File Size: | 4.82 KB |
---|---|
Views Total: | 700 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
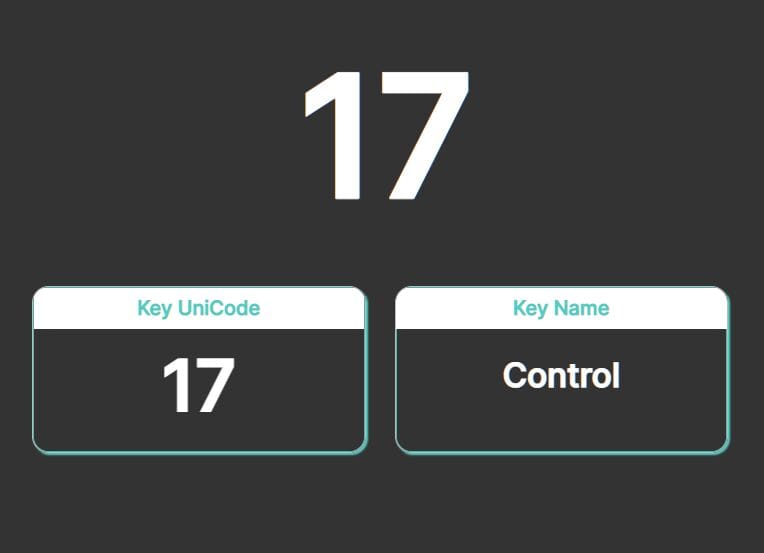
A tiny jQuery based web app that can be used for testing what key on a keyboard is being pressed.
The app detects the JavaScript keydown event and displays the Unicode and Name of a keyboard key when it is pressed.
How to use it:
1. Code the HTML for the key detector.
<div class="input"> <input type="text" class="text"> <span class="result"></span> </div> <div class="options"> <div class="key-code"> <h2>Key UniCode</h2> <span class="code" data-copy="copy-code"></span> </div> <div class="key-name"> <h2>Key Name</h2> <span class="name" data-copy="copy-name"></span> </div> <input class="copy"></input> </div> <span class="copy-code">Copied !!</span> <span class="copy-name">Copied !!</span>
2. Load the necessary jQuery JavaScript library in the document.
<script src="/path/to/cdn/jquery.slim.min.js"></script>
3. The main JavaScript (jQuery script) for the key detector app.
$(function() { $("input.text").focus(); $("input.text").on("keydown", function(e) { var keyUniCode = e.keyCode || e.which, keyText = e.key || e.code; $(this).val($(this).val().slice(0, 1)); $(".input .result").html(keyUniCode); $(".options .key-code .code").html(keyUniCode); $(".options .key-name .name").html(keyText); //Special Cases for some Keys if ($(".options .key-code .code").html() == "32") { $(".options .key-name .name").html("Space"); } if (keyUniCode == 18 || keyUniCode == 122 || keyUniCode == 120 || keyUniCode == 117 || keyUniCode == 116 || keyUniCode == 114 || keyUniCode == 112 || keyUniCode == 123 || keyUniCode == 121 || keyUniCode == 9) { e.preventDefault(); } }); $("body, html").on("click", function() { $("input.text").focus(); }); $(".options .code, .options .name").on("click", function() { var text = $(this).text(); copySpan = $("." + $(this).data("copy")); $("input.copy").val(text).select(); document.execCommand("Copy"); copySpan.animate({ left: 0 }, 600, function() { copySpan.delay(800).animate({ left: "-100%" }, 600); }); }); })
This awesome jQuery plugin is developed by Mohamed-Elhawary. For more Advanced Usages, please check the demo page or visit the official website.