3D Cover Flow-Style Image Carousel Plugin with jQuery - Cloud 9 Carousel
File Size: | 461 KB |
---|---|
Views Total: | 49290 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
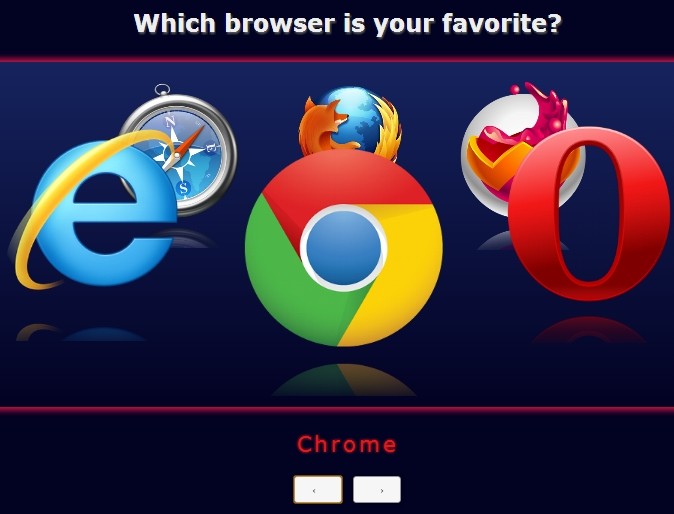
Cloud 9 Carousel is a cool jQuery & Html5 based carousel plugin that has the ability to continuously rotate/slide a set of images with the familiar 3D 'cover flow' effect.
See also:
- jQuery Carousel Image Gallery with Cover-flow Effect - jqcarousel
- Cover-flow Effect with Image Reflection Effect Using jQuery and jQuery UI - qpcoverflow
- Responsive Image Cover Flow Plugin with jQuery and CSS3 - flipster
How to use it:
1. Include the jQuery javascipt library and jQuery cloud 9 carousel plugin at the bottom of the document.
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.0/jquery.min.js"></script> <script src="jquery.cloud9carousel.js"></script>
2. Include the jQuery reflection.js to add instant reflection effects to your images. (Not required but recommended)
<script src="jquery.reflection.js"></script>
3. Wrap the images in an DIV container.
<div id="showcase"> <img class="cloud9-item" src="1.png" alt="Alt"> <img class="cloud9-item" src="2.png" alt="Alt"> <img class="cloud9-item" src="3.png" alt="Alt"> </div>
4. Create an empty container to display the image caption specified in the alt
attribute.
<p id="item-title"> </p>
5. Create a navigation for the image carousel.
<div class="nav"> <button class="left">←</button> <button class="right">→</button> </div>
6. The sample CSS to style the image carousel.
#wrap { /* fixed width, centered in viewport */ width: 980px; left: -490px; margin-left: 50%; position: relative; } #showcase { width: 100%; height: 460px; background: #16235e; /* Old browsers */ background: -moz-linear-gradient(top, #16235e 0%, #020223 100%); /* FF3.6+ */ background: -webkit-gradient(linear, left top, left bottom, color-stop(0%, #16235e), color-stop(100%, #020223)); /* Chrome, Safari4+ */ background: -webkit-linear-gradient(top, #16235e 0%, #020223 100%); /* Chrome10+, Safari5.1+ */ background: -o-linear-gradient(top, #16235e 0%, #020223 100%); /* Opera 11.10+ */ background: -ms-linear-gradient(top, #16235e 0%, #020223 100%); /* IE10+ */ background: linear-gradient(to bottom, #16235e 0%, #020223 100%); /* W3C */ -webkit-box-shadow: 0px 0px 13px 5px #DB1242; -moz-box-shadow: 0px 0px 13px 5px #DB1242; box-shadow: 0px 0px 13px 5px #DB1242; border-radius: 8px; margin-top: 12px; visibility: hidden; } #showcase img { cursor: pointer; } #item-title { color: #F31414; font-size: 29px; letter-spacing: 0.13em; text-shadow: 1px 1px 6px #C72B2B; text-align: center; margin-top: 30px; margin-bottom: 22px; } .nav { text-align: center; } .nav > button { width: 64px; height: 36px; color: #666; font: bold 16px arial; text-decoration: none; text-align: center; margin: 5px; text-shadow: 0px 1px 0px #f5f5f5; background: #f6f6f6; border: solid 2px rgba(0, 0, 0, 0.4); -webkit-border-radius: 5px; -moz-border-radius: 5px; border-radius: 5px; -webkit-box-shadow: 0 0 9px 1px rgba(0, 0, 0, 0.4); -moz-box-shadow: 0 0 9px 1px rgba(0, 0, 0, 0.4); box-shadow: 0 0 9px 1px rgba(0, 0, 0, 0.4); cursor: pointer; } .nav > button:active, .nav > button.down { background: #dfdfdf; border: solid 2px rgba(0, 0, 0, 0.6); box-shadow: none; }
7. The javascript.
<script> $(function() { var showcase = $("#showcase") showcase.Cloud9Carousel( { yPos: 42, yRadius: 48, mirrorOptions: { gap: 12, height: 0.2 }, buttonLeft: $(".nav > .left"), buttonRight: $(".nav > .right"), autoPlay: true, bringToFront: true, onRendered: showcaseUpdated, onLoaded: function() { showcase.css( 'visibility', 'visible' ) showcase.css( 'display', 'none' ) showcase.fadeIn( 1500 ) } } ) function showcaseUpdated( showcase ) { var title = $('#item-title').html( $(showcase.nearestItem()).attr( 'alt' ) ) var c = Math.cos((showcase.floatIndex() % 1) * 2 * Math.PI) title.css('opacity', 0.5 + (0.5 * c)) } // Simulate physical button click effect $('.nav > button').click( function( e ) { var b = $(e.target).addClass( 'down' ) setTimeout( function() { b.removeClass( 'down' ) }, 80 ) } ) $(document).keydown( function( e ) { switch( e.keyCode ) { /* left arrow */ case 37: $('.nav > .left').click() break /* right arrow */ case 39: $('.nav > .right').click() } } ) }) </script>
8. All the default options.
// null: calculated automatically xOrigin: null, yOrigin: null, xRadius: null, yRadius: null, // scale of the farthest item farScale: 0.5, // enable CSS transforms transforms: true, // enable smooth animation via requestAnimationFrame() smooth: true, // fixed frames per second (if smooth animation is off) fps: 30, // positive number speed: 4, // [ 0: off | number of items (integer recommended, positive is clockwise) ] autoPlay: 0, // autoplay delay autoPlayDelay: 4000, bringToFront: false, itemClass: 'cloud9-item', frontItemClass: null, handle: 'carousel'
9. API methods.
// Spin the carousel $("#carousel").data("carousel").go( Count ); // Spin the carousel to a specific item $("#carousel").data("carousel").goTo( Index ); // Returns the 0-based index of the item nearest to the front (integer) $("#carousel").data("carousel").nearestIndex(); // Returns a reference to the item object of the item that is nearest to the front (Item object) $("#carousel").data("carousel").nearestItem(); // Returns the interpolated number of items rotated from the initial zero position. // In a carousel with 5 items that made three clockwise revolutions, the value will be -15. // If the carousel then further spins half-way to the next item, then the value would be -15.5 (float) $("#carousel").data("carousel").itemsRotated(); // Returns an interpolated value of the item "index" at the front of the carousel. // If, for example, the carousel was 20% past item 2 toward the next item, then floatIndex() would return 2.2 (float) $("#carousel").data("carousel").floatIndex(); // Disable the carousel (currently irreversible). $("#carousel").data("carousel").deactivate();
Change logs:
2017-11-22
- Added optional classname for automatically tagging the frontmost item
2016-11-30
- Added goTo(index) method.
2016-07-02
- Added onAnimationFinished() event
2015-03-04
- Made left/right button controls optional
2014-12-23
- Carousel item image properties preserved properly after mirroring
2014-04-26
- Carousel item image properties preserved properly after mirroring
2014-02-16
- CSS transforms detected and enabled by default
2014-02-14
- Removed redundant resizing code from Item.moveTo()
- Set smooth animation mode as default
- Removed redundant resizing code from Item.moveTo()
This awesome jQuery plugin is developed by specious. For more Advanced Usages, please check the demo page or visit the official website.