Super Simple jQuery Content Slider Plugin For jQuery
File Size: | 7.7 KB |
---|---|
Views Total: | 6298 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
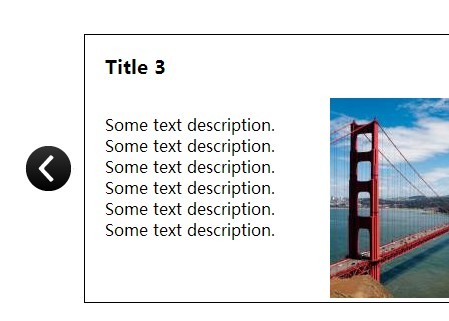
A very simple and lightweight jQuery plugin used to create a content slider with next/prev arrows navigation. The arrows navigation will automatically be hidden as your reach the first or last slide.
How to use it:
1. Create left/right arrows for next/prev navigation.
<div class="prev crousel-navigation"></div> <div class="next crousel-navigation"></div>
2. Wrap your content slides into a container using Html unordered list.
<div class="slider-container"> <ul> <li> <h3>Title 1</h3> <p>Some text description. </p> <div class="crousel-image-outer"> <img src="images/1.png"> </div> </li> <li> <h3>Title 2</h3> <p>Some text description. </p> <div class="crousel-image-outer"> <img src="images/2.png"> </div> </li> <li> <h3>Title 3</h3> <p>Some text description.</p> <div class="crousel-image-outer"> <img src="images/3.png"> </div> </li> </ul> </div>
3. Wrap the navigation controls & slider container into a position: relative
element. The Html structure should be like this:
<div class="main-slider-container"> <!-- Slider controls --> <!-- Slider container --> </div>
4. Add the following basic CSS snippets into your document.
.main-slider-container { position: relative; margin: 0 auto } .slider-container { position: absolute; overflow: hidden; border: 1px solid #000; } ul { position: relative; margin: 0; padding: 0; } li { list-style-type: none; position: relative; float: left; } .disable-link { pointer-events: none; } .prev { left: -60px; background: url(images/prev.png); } .next { right: -60px; background: url(images/next.png); } .disable-link.prev { background: none; } .disable-link.next { background: none; } .crousel-navigation { position: absolute; top: 110px; width: 50px; height: 50px; } /* Some other style to butify content */ .slider-container h3, .slider-container p { margin-left: 20px; margin-right: 20px; } .slider-container p { width: 200px; display: inline-block; vertical-align: top; } .slider-container .crousel-image-outer { margin-right: 20px; display: inline-block; width: 200px; }
5. Include jQuery library and the slider.js script in your document.
<script src="http://code.jquery.com/jquery-1.11.1.min.js"></script> <script src="libs/slider.js"></script>
6. Enable the content slider with default options.
$(document).ready(function() { $(".main-slider-container").slider(); });
7. Options available.
$(document).ready(function() { $(".main-slider-container").basicSlider({ // slider length crouselLength: 4, // slider width crouselWidth: 500, // current slide currentTab : 1 }); });
Change log:
2017-06-13
- Avoid name conflicts
This awesome jQuery plugin is developed by meerajwadhwa15. For more Advanced Usages, please check the demo page or visit the official website.