Simple Table Rows Sorting Plugin with jQuery
File Size: | 48.9 KB |
---|---|
Views Total: | 6353 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
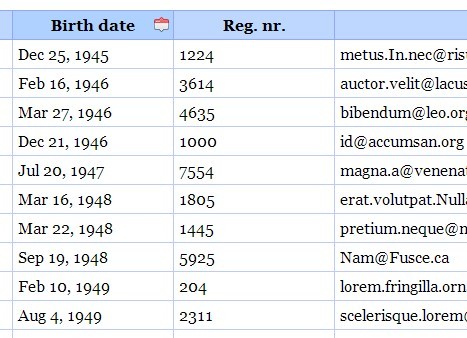
A simple lightweight jQuery plugin which allows your to sort table rows with custom sorting rules (e.g. numeric, date, alphabetical, etc.).
How to use it:
1. Load the jQuery Simple Table Sort plugin after jQuery library.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script> <script src="jquery.simpleTableSort.js"></script>
2. Create a sortable table following the Html structure like this:
<table id="bigTable"> <thead> <tr> <tr> <th class="sort-alphabetical">Name</th> <th class="sort-numeric">Postal</th> <th class="sort-alphabetical">City</th> <th class="sort-alphabetical">Country</th> <th class="sort-date">Birth date</th> <th class="sort-numeric">Reg. nr.</th> </tr> </thead> <tbody> <!-- lots of data --> </tbody> </table>
3. Call the plugin on the big table to enable the table sort functionality.
/** * Changes the prefix of the used css classes in the plugin. * These classes will be appended to the head columns to determine which state they were. * If the prefix 'sort' is already used in your project you can change it here */ prefix: 'sort', /** * The sort order in which your table entries will be sorted first. * Possible entries: * - asc: Ascending order (a-z, 0-9, ...) * - desc: Descending order (z-a, 9-0, ...) */ order: 'asc', /** * If the table is dynamic, meaning the table gets updated by AJAX or something else, this setting should be set to true. * Otherwise the initial table data is restored. * * This is for performance reason: If it's a static table the rows don't have to be reprocessed every sort change. */ dynamic: false, /** * With this option set to 'true' the state classes of the columns (e.g. 'sort-asc' and 'sort-desc') won't be removed when a different column is sorted. * However, the last sort state is never 'forgotten', it will always be the opposite of the last sorted (or the default) */ multiSortStates: false, /** * Adds the possibility to sort the table entries when the table is created. * Accepts index values of table head column (zero-based). */ autoSort: null, /** * You can add your own sort methods here. * * Example: Change "dd-mm-yyy" formatted date to JavaScript compatible "mm-dd-yyyy": * sortMethods: { * myDate: function(a, b) { *a = a.split('.'); *a = a[1]+'.'+a[0]+'.'+a[2]; * *b = b.split('.'); *b = b[1]+'.'+b[0]+'.'+b[2]; * *return new Date(a) > new Date(b) ? 1 : -1; * } * } */ sortMethods: { numeric: function(a, b) { return (parseInt(a) - parseInt(b)); }, float: function(a, b) { return (parseFloat(a) - parseFloat(b)); }, alphabetical: function(a, b) { return (a.toLowerCase() > b.toLowerCase()) ? 1 : -1; }, date: function(a, b) { a = new Date(a); b = new Date(b); if (priv.helper.isDate(a) && priv.helper.isDate(b)) { return (a > b) ? 1 : -1; } return 0; } }, /** * If you have no control over the table structure and it lacks of a table head, then set this value to true * and the first row of your table will be treatened as table head column */ fixTableHead: false, /** * Table head columns can be excluded from to be sorted by adding the index. * * Example which excludes the second row (zero-based): * excludeSortColumns: [ 1 ] */ excludeSortColumns: [], /** * Callbacks */ onBeforeSort: function () {}, onAfterSort: function () {}
4. Essential CSS styles for the table sort plugin.
th.sort-disabled { background-image: none; } th.sort-alphabetical.sort-asc { background-image: url('assets/sort-alphabet.png') } th.sort-alphabetical.sort-desc { background-image: url('assets/sort-alphabet-descending.png') } th.sort-numeric.sort-asc { background-image: url('assets/sort-number.png') } th.sort-numeric.sort-desc { background-image: url('assets/sort-number-descending.png') } th.sort-date.sort-asc { background-image: url('assets/sort-date.png') } th.sort-date.sort-desc { background-image: url('assets/sort-date-descending.png') } th.sort-customMail.sort-asc { background-image: url('assets/sort-rating.png') } th.sort-customMail.sort-desc { background-image: url('assets/sort-rating-descending.png') }
This awesome jQuery plugin is developed by dan-lee. For more Advanced Usages, please check the demo page or visit the official website.