Full-featured Dynamic Data Table Plugin - Folium
File Size: | 19.6 KB |
---|---|
Views Total: | 5850 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
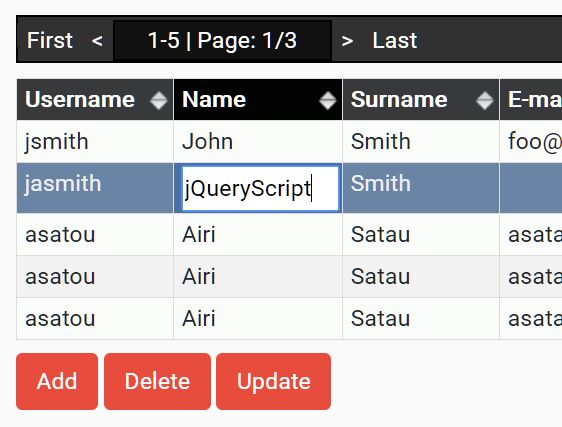
Folium is a jQuery data table plugin for creating spreadsheet-like CRUD data grids with sorting, filtering, pagination, and custom cell rendering support.
Key features:
- Sortable table rows.
- Filterable with a search field.
- Add/Remove/Update table rows.
- Editable table cells.
- Render tabular data in a custom format.
- Scrollable with fixed table header.
- Pagination bar for large data set.
- Callback functions wnen you click/double click a table row
How to use it:
1. To use the plugin, include jQuery library and the Folium's files on the web page.
<link href="/path/to/folium.css" rel="stylesheet"> <script src="/path/to/jquery.min.js"></script> <script src="/path/to/folium.js"></script>
2. Define your tabular data (columns & rows) in the JavaScript as follows:
// Columns const colData = [{ columnId: "id1", displayText: "ID 1" },{ columnId: "id2", displayText: "ID 2" },{ columnId: "id3", displayText: "ID 3" }], // Rows rowData = [{ id1: 'Cell 1.1', id2 : "Cell 1.2", id3 : "Cell 1.3" },{ id1: 'Cell 2.1', id2 : "Cell 2.2", id3 : "Cell 2.3" },{ id1: 'Cell 3.1', id2 : "Cell 3.2", id3 : "Cell 3.3" }]
3. Polulate your HTML table with the tabular data you defiend.
<table id="example""></table>
const myTable = $('#foliumTableId').FoliumTable({ columns: colData, rows: rowData });
4. Enable the user to sort the table by clicking on the table header.
const myTable = $('#foliumTableId').FoliumTable({ columns: colData, rows: rowData, sortable: true });
5. Enable the user to edit table cells.
const myTable = $('#foliumTableId').FoliumTable({ columns: colData, rows: rowData, editable: true });
6. Enable the user to filter through table rows with a search box.
<input type="text" id="searchBox" />
$('#searchBox').on('keyup',function(){ const searchBoxText = $('#searchBox').val(); foliumTable.search(searchBoxText); // allows the first column foliumTable.search(searchBoxText, 0); });
7. Add a pagination bar to the table and specify the number of table rows to display per page.
const myTable = $('#foliumTableId').FoliumTable({ columns: colData, rows: rowData, pagination: { active: true, size: 5 } });
8. Add/remove/update table rows.
// add a new row myTable.addRow({ id1: 'ID 4.1', id2: "ID 4.2", id3: "ID 4.3" }); // delete row 1 myTable.deleteRows(0); // detete row 1,2 myTable.deleteRows([0, 1]); // update a row myTable.updateRow(0, { id1: 'new value' });
9. Custom cell rendering.
const myTable = $('#foliumTableId').FoliumTable({ columns: colData, rows: rowData, cellRenderer : function(rowIndex, columnIndex, data, rowObject) { if (columnIndex === 5) return `<a href="${rowObject.linkUrl}">${data}</a>`; return data; } });
10. API methods.
// returns the selected row index myTable.rselectedRow() // returns the selected row index according to the table model myTable.selectedRowInModel(); // returns the selected column index myTable.selectedColumn(); // returns the number of rows myTable.rowCount(); // returns the number of columns myTable.columnCount(); // returns the row from the model according to the index parameter myTable.getRow(index); // returns the model of the table myTable.getRows(): // returns the current page number myTable.currentPage(); // returns the id of the table myTable.getId(); // clears the data myTable.clear();
11. Event handlers.
// on click myTable.on('rowClicked', rowIndex => { console.log(`Clicked Row Index: ${rowIndex}`); }); // double click myFoliumTable.on('rowDoubleClicked', rowIndex => { console.log(`Clicked Row Index: ${rowIndex}`); });
Changelog:
v1.1.3beta (2019-11-21)
- Changed search function behaviour
2019-10-22
- Fixed a bug that causes not to re-render the table when a row is updated in rows as array mode.
2019-10-12
- Fixed bugs that were causing not to render the table when pagination is not active
This awesome jQuery plugin is developed by cemozden. For more Advanced Usages, please check the demo page or visit the official website.