Simple AJAX-enabled Inline Editing Plugin For jQuery
File Size: | 17.3 KB |
---|---|
Views Total: | 1453 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
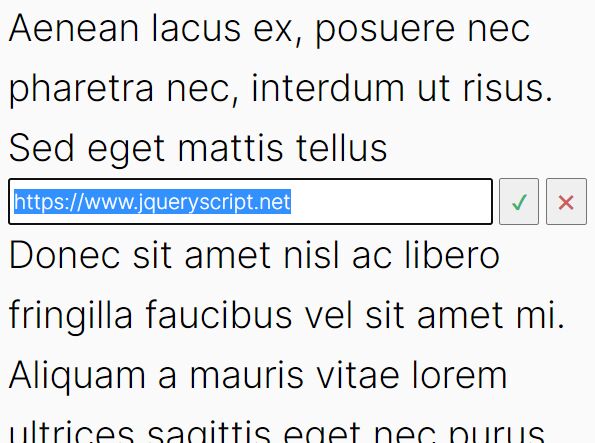
An easy-to-use inline editing (also called in-place editing) jQuery plugin that sends edited data as a JS object to the server via AJAX requests ("POST", "GET", or "PUT").
How to use it:
1. Insert the jQuery SimpleEdit plugin's files into the document.
<link rel="stylesheet" href="/path/to/css/jquery-simpleedit.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/js/jquery-simpleedit.js"></script>
2. Call the plugin on the element that should be editable. Available HTML data attributes:
- data-name: Field name
- data-pk: Primary key
- data-url: Path to the server-side script that processes the submitted value
<a href="#" id="url" data-name="url" data-pk="1" data-url="/post"> https://www.jqueryscript.net </a>
$(function(){ $('#url').simpleedit() });
4. You can set the options in the JavaScript as follows:
$('#url').simpleedit({ name: 'url' pk: 1, url: '/post', })
5. Get the edited content:
$('#url').simpleedit(function (changedText) { console.log(changedText) })
6. Pass the parameters to the simpleedit()
as follows:
$('#url').simpleedit( '/path/to/server/', function(){ // callback 1 }, 'cancel', function(){ // callback 2 } )
7. Success & error handlers:
$('#url').simpleedit( success: function(response) { console.log(response.msg) } error: function(response) { console.log(response.msg) } )
8. All default configs.
$.fn.simpleedit.defaults = { availableOptions: { mode: ['inline'], onblur: ['cancel', 'submit', 'ignore'], type: [ 'text', ], }, ajaxOptions: { type: 'POST', }, buttons: { submit: `<button class="submit"><span>✔</span></button>`, abort: `<button class="abort"><span>✖</span></button>`, spinner: `<button class="loader"><span class="simpleedit-spinner">⯋</span></button>`, }, callbacks: [], error: null, mode: 'inline', onblur: 'cancel', success: null, type: 'text', }
$.fn.simpleedit.defaults.ajaxOptions = { type: 'PUT', }
This awesome jQuery plugin is developed by WalterWoshid. For more Advanced Usages, please check the demo page or visit the official website.