Dynamic Event Calendar For Bootstrap 4
File Size: | 8.97 KB |
---|---|
Views Total: | 38780 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
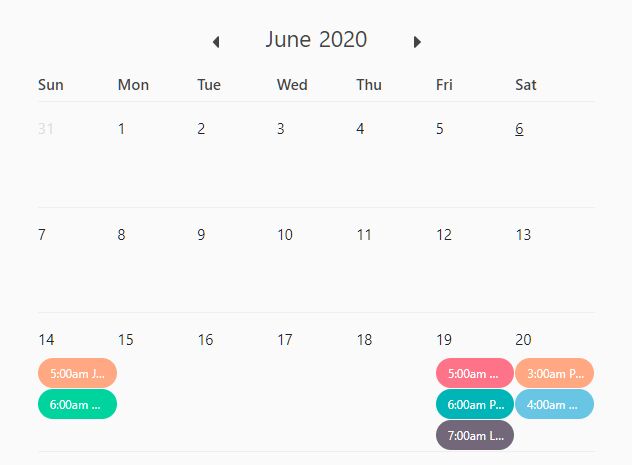
A minimal clean calendar component for Bootstrap 4 that dynamically displays events on a month view.
Dependencies:
- jQuery
- Bootstrap 4 framework
- Moment.js
How to use it:
1. To use the component, make sure you have jQuery library and Bootstrap 4 framework loaded in the document.
<link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/cdn/bootstrap.min.js"></script>
2. Load the latest moment.js to handle date & time.
<script src="/path/to/cdn/moment.min.js"></script>
3. Load the calendar's stylesheet in the document.
<link rel="stylesheet" href="css/calendar.css" />
4. Create a DIV container to hold the event calendar.
<div id="calendar"></div>
5. Import the loading spinner module if needed.
<link rel="stylesheet" href="css/spinner.css" />
import {Spinner} from './spinner.js';
6. Define & import your events as follows:
// mockData.js export const mockData = [ { time: '2020-06-13T21:00:00 Z', cls: 'bg-orange-alt', desc: 'Jack, Stephen' }, { time: '2020-06-13T22:00:00 Z', cls: 'bg-green-alt', desc: 'Nathan, Luke' }, { time: '2020-06-18T21:00:00 Z', cls: 'bg-red-alt', desc: 'Nathan, Stephen' }, { time: '2020-06-18T22:00:00 Z', cls: 'bg-cyan-alt', desc: 'Peter, Luke' }, { time: '2020-06-18T23:00:00 Z', cls: 'bg-purple-alt', desc: 'Lora, Sandy' }, { time: '2020-06-19T20:00:00 Z', cls: 'bg-sky-blue-alt', desc: 'Nathan, Luke' }, { time: '2020-06-19T19:00:00 Z', cls: 'bg-orange-alt', desc: 'Peter, Luke' }, { time: '2020-05-22T21:00:00 Z', cls: 'bg-sky-blue-alt', desc: 'Peter, Lora' }, { time: '2020-07-02T19:00:00 Z', cls: 'bg-purple-alt', desc: 'Peter, Luke' } ];
import {mockData} from './mockData.js';
7. Import the calendar component.
import {Calendar} from './calendar.js';
8. Initialize the calendar and load event data into the calendar asynchronously.
document.addEventListener("DOMContentLoaded", async ()=>{ const cal = Calendar('calendar'); const spr = Spinner('calendar'); await spr.renderSpinner().delay(0); cal.bindData(mockData); cal.render(); });
Changelog:
2022-06-03
- updated moment.js dependency
This awesome jQuery plugin is developed by ylli2000. For more Advanced Usages, please check the demo page or visit the official website.