Collapsible Tree View With Checkboxes - jQuery hummingbird-treeview
File Size: | 32.2 KB |
---|---|
Views Total: | 77807 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
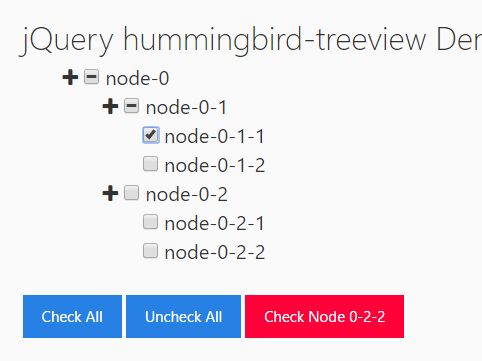
hummingbird-treeview is a jQuery plugin that transforms nested html lists into an expandable, collapsible, searchable, checkable, hierarchical tree structure with lots of useful options and APIs.
Also includes support for 'indeterminate' state in the checkbox inputs.
See also:
How to use it:
1. Create an HTML tree of nested unordered lists as follows. The data-str
attribute is used to add more custom functionality to the nodes.
<!-- Use a pseudo HTML list --> <div class="hummingbird-treeview-converter"> <li id="item_1" data-id="node_1">Node 1</li> <li id="item_2" data-id="node_1_1">-Node 1-1</li> <li id="item_3" data-id="node_1_1_1">--Node 1-1-1</li> <li id="item_4" data-id="node_1_2">-Node 1-2</li> <li id="item_5" data-id="node_2" data-str='data-toggle="tooltip" data-placement="auto" title="Custom Title"'>Node 2</li> ... </div> <!-- Use a real HTML list --> <div id="treeview_container" class="hummingbird-treeview"> <ul id="treeview" class="hummingbird-base"> <li data-id="0"> <i class="fa fa-plus"></i> <label> <input id="xnode-1" data-id="custom-1" type="checkbox" /> node-1 </label> <ul> <li data-id="1"> <i class="fa fa-plus"></i> <label> <input id="xnode-1-1" data-id="custom-1-1" type="checkbox" /> node-1-1 </label> <ul> <li> <label> <input class="hummingbird-end-node" id="xnode-1-1-1" data-id="custom-1-1-1" type="checkbox" /> node-1-1-1 </label> </li> </ul> </li> <li data-id="1"> <i class="fa fa-plus"></i> <label> <input id="xnode-1-2" data-id="custom-1-2" type="checkbox" /> node-1-2 </label> <ul> <li> <label> <input class="hummingbird-end-node" id="xnode-1-2-1" data-id="custom-1-2-1" type="checkbox" /> node-1-2-1 </label> </li> </ul> </li> </ul> </li> </ul> </div>
2. Download and load the jQuery hummingbird-treeview plugin's files along with the jQuery library into the document.
<link href="hummingbird-treeview.css" rel="stylesheet"> <script src="//code.jquery.com/jquery.min.js"></script> <script src="hummingbird-treeview.js"></script>
3. Load the Font Awesome (4 or 5) for the necessary icons.
<link rel="stylesheet" href="/path/to/font-awesome.min.css">
4. Just initialize the plugin and done.
$("#treeview").hummingbird();
5. Available data
attributes:
- data-height: Height of the tree view
- data-scroll: Determine if the tree view is scrollable
- data-id: Unique ID
- data-boldParents: set the text of all parent nodes to BOLD
- data-css: inject arbitrary CSS, except text colors and background colors
- data-nonHoverColor
- data-nonHoverColor_bg
- data-HoverColor
- data-HoverColor_bg
<div class="hummingbird-treeview-converter" data-height="450px" data-scroll="true" data-id="_example" data-boldParents="true"> ... </div>
6. Override the global settings.
// Font Awesome prefix // "fas" or "far" for Font Awesome 5 $.fn.hummingbird.defaults.SymbolPrefix = "fa" // Collapsed Symbol $.fn.hummingbird.defaults.collapsedSymbol = "fa-plus"; // Expand Symbol $.fn.hummingbird.defaults.expandedSymbol = "fa-minus"; // Collapse all nodes on init $.fn.hummingbird.defaults.collapseAll = true; // Enable checkboxes $.fn.hummingbird.defaults.checkboxes = "enabled"; // Set this to "disabled" to disable all checkboxes from nodes that are parents $.fn.hummingbird.defaults.checkboxesGroups= "enabled"; // New option singleGroupOpen to allow only one group to be open and collapse all others. // The number provided defines the level to which the function should be applied (starting at 0). $.fn.hummingbird.defaults.singleGroupOpen = -1; // Enable a mouse hover effect on items $.fn.hummingbird.defaults.hoverItems = false; // Set this to true to enable the functionality to account for n-tuples (doubles, triplets, ...). $.fn.hummingbird.defaults.checkDoubles = false; // Or "bootstrap" to use Bootstrap's styles $.fn.hummingbird.defaults.hoverMode = "html"; // Background color on hover $.fn.hummingbird.defaults.hoverColorBg1 = "#6c757c"; // Background color on non hover $.fn.hummingbird.defaults.hoverColorBg2 = "white"; // Text color on hover $.fn.hummingbird.defaults.hoverColorText1 = "white"; // Text color on non hover $.fn.hummingbird.defaults.hoverColorText2 = "black"; // Use Bootstrap colors $.fn.hummingbird.defaults.hoverColorBootstrap = "bg-secondary text-white"; // Set this to "enabled" to add collapse and expand functionality to a click on a parent node name. $.fn.hummingbird.defaults.clickGroupsToggle = "disabled";
7. API methods.
// check all nodes $("#treeview").hummingbird("checkAll"); // uncheck all nodes $("#treeview").hummingbird("uncheckAll"); // collapse all nodes $("#treeview").hummingbird("collapseAll"); // expand all nodes $("#treeview").hummingbird("expandAll"); // check a specific node $("#treeview").hummingbird("checkNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], }); // uncheck a specific node $("#treeview").hummingbird("uncheckNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], }); // expand a specific node $("#treeview").hummingbird("expandNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], expandParents:false }); // collapse a specific node $("#treeview").hummingbird("collapseNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], collapseChildren:false }); // Disables expand and collapse functionality of a node, which is identified by its id or data-id, which has to be defined in the attr parameter. The name parameter holds the name of the id or data-id. $("#treeview").hummingbird("disableToggle",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], }); // disable a specific node $("#treeview").hummingbird("disableNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], state:true, disableChildren:true, }); // enable a specific node $("#treeview").hummingbird("enableNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], state:true, disableChildren:true, }); // hide a node $("#treeview").hummingbird("hideNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], state:true, disableChildren:true, }); // show a node $("#treeview").hummingbird("showNode",{ sel:"id", // "id", "data-id" or "text" vals:["hum_1","hum_2","hum_3"], state:true, disableChildren:true, }); // disable parent node on collapse $("#treeview").hummingbird("showNode",{ sel:"id", vals: ["hum_5","hum_6"], state:true }); // add nodes $("#treeview").hummingbird('addNode',{ pos:['after','after'], anchor_sel:['text','id'], anchor_vals:['node-0-1-1-2','hum_5'], text:['New Node1','New Node2'], the_id:['new_id1','new_id2'], data_id:['new_data_id1','new_data_id2'] }); // remove a node $("#treeview").hummingbird('removeNode',{ sel:"id", // "id", "data-id" or "text" vals:"node-0-1-1-2", state:true, disableChildren:true, }); // get checked nodes var List = {"id" : [], "dataid" : [], "text" : []}; $("#treeview").hummingbird(getChecked",{ list:List, onlyEndNodes:true, onlyParents:false, fromThis:false }); // get unchecked nodes var List = {"id" : [], "dataid" : [], "text" : []}; $("#treeview").hummingbird(getUnchecked",{ list:List, onlyEndNodes:true, onlyParents:false }); // get indeterminate nodes var List = {"id" : [], "dataid" : [], "text" : []}; $("#treeview").hummingbird("getIndeterminate",{ list:List }); // save the state of the treeview for later rebuilding var treeState = {}; $("#treeview").hummingbird("saveState",{ save_state:treeState }); // restore the saved state $("#treeview").hummingbird("restoreState",{ restore_state:treeState }); // a function that controls the tri-state functionality $("#treeview").hummingbird("triState"); // Skip firing the CheckUncheckDone event in the following call. // This method can be called before any other method to skip firing the CheckUncheckDone event in the followed method. $("#treeview").hummingbird("skipCheckUncheckDone"); // remove all nodes which NOT match a search patter $("#treeview").hummingbird("filter",{ str:".txt|.jpg|test", caseSensitive: false, box_disable:false, onlyEndNodes:false, filterChildren:true }); // If the treeview is embedded in a scrollable (css option: overflow-y: scroll;) container, this container must be identified here as the treeview_container (using the id). Otherwise treeview_container should be set to "body". // The search_input parameter depicts the id of the input element for the search function. // The search_output defines an element to bind the search results on (like the ul in the example below). // The search_button is simply the button for the search. // A scrollOffset in pixels (negative or positive) can be defined to adjust the automatic scoll position. // The best value must be observed by testing. onlyEndNodes is per default false, thus set this to true if the search should include also parent nodes. // The optional parameter dialog is per default empty (""). This parameter can be used for special cases, to bind the treeview to a dynamical created object like a bootstrap modal (pop-up). // In such a case this parameter would be dialog:".modal-dialog". // Three other optional parameters, EnterKey, enter_key_1 and enter_key_2 are available. // EnterKey is per default true and can be set to false. If true the search_button can be triggered with the Enter key. // To avoid interference of the Enter key functionality it can be restricted and only be executable if enter_key_1 == enter_key_2. // These two parameters can be chosen arbitrarily. $("#treeview").hummingbird("search",{ treeview_container:"treeview_container", search_input:"search_input", search_output:"search_output", search_button:"search_button", scrollOffset:-515, onlyEndNodes:false });
8. Events available.
$("#treeview").on("nodeChecked", function(){ // when checked }); $("#treeview").on("nodeUnchecked", function(){ // when unchecked }); $("#treeview").on("CheckUncheckDone", function(){ // when checked or unchecked });
Changelog:
v3.0.5 (2022-08-17)
- New data-* attributes to set text colors and background colors for individual nodes: data-nonHoverColor, data-nonHoverColor_bg, data-HoverColor, data-HoverColor_bg.
v3.0.3 (2022-03-25)
- New method disableParentNodeOnCollapse
v3.0.3 (2022-03-12)
- The "2. Simple pseudo HTML plus" input format has now an additional parameter data-css to inject arbitrary CSS.
v3.0.1 (2022-02-25)
- allow special chars in vals
v3.0.0 (2021-06-02)
- Big upgrade to mass assignments. If you have used the treeview only interactively, no change needed. However, if you have used it programatically with respective methods, you have to change your syntax and concept!
v2.1.6 (2021-02-08)
- Added new method skipCheckUncheckDone
- Bugfix on disable/enable
v2.1.5 (2021-02-01)
- Added new methods hide / show.
- Added New feature data-str.
v2.1.4 (2020-11-19)
- bugfixes disabled nodes
v2.1.1 (2020-10-23)
- bug fix on disabled nodes which changed color on hover
2020-07-09
- JS Update
2020-07-08
- Bugfix
2020-06-30
- Added
data-id
todiv class="hummingbird-treeview-converter"
to set individual id's to address the treeview e.g. via$("#treeview_movies").hummingbird();
.
2020-03-18
- update enable, disable, li to label and color
2020-03-04
- New method: disableToggle
- New option: clickGroupsToggle
2020-01-31
- New option: Customizable hover effect.
2019-12-24
- JS Update
2019-12-04
- Added singleGroupOpen option
2019-11-26
- Add font-awesome 5 support
2019-08-31
- Implementation of case-insensitive filter search as default with ability to override.
2019-04-30
- add font-awesome 5 support
2018-11-19
- add parent nodes containing parent nodes
2018-11-14
- Add nowrap to hummingbird-base class in the CSS
2018-10-18
- add new filter functionality
2018-10-17
- add disable function to filter
2018-10-11
- new getChecked
2018-10-10
- add filter function
2018-07-25
- Support multiple treeviews on one page
2017-11-18
- Some fixes due to the new function that parent nodes can be disabled while the children are enabled. In this case, the disabled parent provides full tri-state functionality. Child nodes can be enabled/disabled on the fly. It is important that enabled parent nodes, which have all children disabled, are not allowed.
2017-11-16
- now always full tri-state functionality for disabled, this cannot be disabled anymore
2017-11-16
- add new option checkboxesGroups and update disableNode and enableNode
2017-09-01
- new getChecked atrr: text
- use new class name hummingbird-end-node
2017-08-30
- bug fixed on uncheckAll method
This awesome jQuery plugin is developed by hummingbird-dev. For more Advanced Usages, please check the demo page or visit the official website.