Lightweight jQuery Date Input Picker - pickadate
File Size: | 165 KB |
---|---|
Views Total: | 34946 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
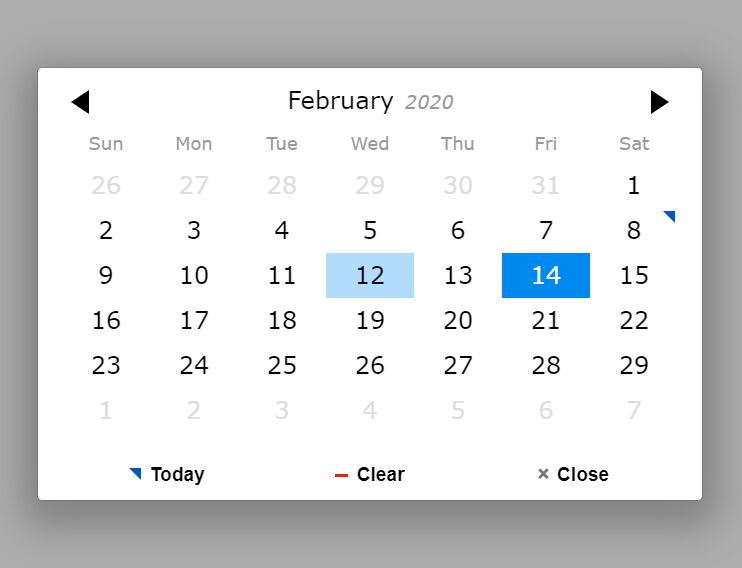
pickadate is a lightweight, fully responsive, accessible, and mobile-compatible date & time picker plugin for the web.
This is the #1 jQuery date picker plugin around the web.
Key Features:
- Mobile friendly. Supports both desktop and touch devices.
- WCAG compliant.
- Supports more than 40 languages.
- RTL supported as well.
- Tons of customization options.
- Powerful API.
How to use it :
1. Include a Pickadate theme of your choice on the page.
<!-- Default Theme (Core) --> <link rel="stylesheet" href="lib/themes/pickadate.default.css"> <!-- Default Theme (Date Picker) --> <link rel="stylesheet" href="lib/themes/default.date.css"> <!-- Default Theme (Time Picker If Needed)--> <link rel="stylesheet" href="lib/themes/default.time.css"> <!-- Classic Theme (Core) --> <link rel="stylesheet" href="lib/themes/pickadate.classic.css"> <!-- Classic Theme (Date Picker) --> <link rel="stylesheet" href="lib/themes/classic.date.css"> <!-- Classic Theme (Time Picker If Needed)--> <link rel="stylesheet" href="lib/themes/classic.time.css"> <!-- For RTL Layout --> <link rel="stylesheet" href="lib/themes/rtl.css">
2. Create an input field for the date & time picker.
<input id="exampleDate" class="datepicker" name="date" type="text" value="14 August, 2014" data-value="2014-08-08" /> <input id="exampleTime" class="timepicker" type="time" name="time" valuee="2:30 AM" data-value="0:00" />
3. Include jQuery library and the pickadate.js plugin's files on your web page.
<!-- jQuery --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Core --> <script src="/path/to/lib/picker.js"></script> <!-- Date Picker --> <script src="/path/to/lib/picker.date.js"></script> <!-- Time Picker --> <script src="/path/to/lib/picker.time.js"></script> <!-- Language --> <script src="/path/to/lib/translations/fr_FR.js"></script> <!-- Required For Legacy Browsers (IE 8-) --> <script src="/path/to/lib/legacy.js"></script>
4. Call the function on the input filed and one.
// date picker $('.datepicker').pickadate(); // time picker $('.timepicker').pickatime();
5. Possible options for the date picker.
$('.datepicker').pickadate({ // The title label to use for the month nav buttons labelMonthNext: 'Next month', labelMonthPrev: 'Previous month', // The title label to use for the dropdown selectors labelMonthSelect: 'Select a month', labelYearSelect: 'Select a year', // Months and weekdays monthsFull: [ 'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December' ], monthsShort: [ 'Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec' ], weekdaysFull: [ 'Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday' ], weekdaysShort: [ 'Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat' ], // Today and clear today: 'Today', clear: 'Clear', close: 'Close', // Picker close behavior closeOnSelect: true, closeOnClear: true, // Update input value on select/clear updateInput: true, // The format to show on the `input` element format: 'd mmmm, yyyy', // Classes klass: { table: prefix + 'table', header: prefix + 'header', navPrev: prefix + 'nav--prev', navNext: prefix + 'nav--next', navDisabled: prefix + 'nav--disabled', month: prefix + 'month', year: prefix + 'year', selectMonth: prefix + 'select--month', selectYear: prefix + 'select--year', weekdays: prefix + 'weekday', day: prefix + 'day', disabled: prefix + 'day--disabled', selected: prefix + 'day--selected', highlighted: prefix + 'day--highlighted', now: prefix + 'day--today', infocus: prefix + 'day--infocus', outfocus: prefix + 'day--outfocus', footer: prefix + 'footer', buttonClear: prefix + 'button--clear', buttonToday: prefix + 'button--today', buttonClose: prefix + 'button--close' } });
6. Possible options for the time picker.
$('.timepicker').pickadate({ // Clear clear: 'Clear', // The format to show on the `input` element format: 'h:i A', // The interval between each time interval: 30, // Picker close behavior closeOnSelect: true, closeOnClear: true, // Update input value on select/clear updateInput: true, // Classes klass: { picker: prefix + ' ' + prefix + '--time', holder: prefix + '__holder', list: prefix + '__list', listItem: prefix + '__list-item', disabled: prefix + '__list-item--disabled', selected: prefix + '__list-item--selected', highlighted: prefix + '__list-item--highlighted', viewset: prefix + '__list-item--viewset', now: prefix + '__list-item--now', buttonClear: prefix + '__button--clear' } });
7. Event handlers (callback functions).
$('.datepicker').pickadate({ onStart: function() { console.log('Hello there :)') }, onRender: function() { console.log('Whoa.. rendered anew') }, onOpen: function() { console.log('Opened up') }, onClose: function() { console.log('Closed now') }, onStop: function() { console.log('See ya.') }, onSet: function(context) { console.log('Just set stuff:', context) } }); // or var $input = $('.datepicker').pickadate() var picker = $input.pickadate('picker') picker.on('open', function(){ // do something }); picker.off('open', function(){ // do something });
8. API methods.
var $input = $('.datepicker').pickadate() var picker = $input.pickadate('picker') // open picker.open(); // open without focus picker.open(false); // close picker.close(); // close with focus picker.close(true); // reinit picker.start(); // destroy picker.stop(); // render picker.render(); // clear picker.clear(); /* parameters: value (default) select highlight view min max open start id disable enable */ picker.get(parameter); /* parameters: clear select highlight view min max disable enable interval */ picker.set(parameter);
9. For more examples, please check the Doc Page.
Changelog:
2020-02-08
- Doc & Demo Updated
2019-05-26
- v3.6.4
2019-03-12
- Fixed languages.
2018-04-17
- Fixed languages.
2018-04-03
- bind click and focus events when editable
2018-04-02
- update input settings.
2018-03-14
- update
2018-03-05
- fix hover states
2018-02-25
- Prevent paint when hidden
2018-02-19
- add Khmer translation
2018-02-14
- Add a timeout so IE8 has time to finish rendering
v3.5.6 (2015-04-20)
- Fixed issue where script was executed before body was loaded.
- Fixed re-binding events to P.$holder after a re-render.
- Added Persian translations.
- Fix in Chinese translations.
- Fix in Japanese translations.
v3.5.5 (2015-02-09)
- Fixed date picker to use UTC dates.
- Fixed time picker to use local times.
- Fixed color for “clear” button on time picker.
- Translations fixes for Italian, Slovak, French, Russian, Spanish, Hindi, Brazilian Portuguese
- Added Common JS to UMD.
- Added “close” button.
- Allow clear method to be muted.
- Added valueSubmit to get value of hidden input element.
- Added ability to set min/max date & time using formatted strings.
- Added French accessibility labels.
- Fixed time offset issues by using UTC based times.
- Fixed the main files in bower.json.
- Fixed flickering on webkit browsers.
- Fixed adding an ID to the hidden element to remain unique.
- Fixed “today” button to be disabled when the date is disabled.
- Fixed time picker not scrolling to correct position with the “classic” theme.
- Fixed mutation of date object passed to normalize.
- Fixed IE8 getComputedStyle error.
- Fixed IE8 error on changing input type.
- Fixed IE8 error of picker in iframe.
- Fixed iOS8 bug of picker not opening in view.
- Fixed bug where it was possible to unbind internal bindings.
- Updated docs the trigger method’s ability to pass data to event callbacks.
- Fixed hidden input to move into container when option is used.
- Added ARIA label for dates and times.
- Removed the Sizzle dependency.
- Added closeOnSelect and closeOnClear boolean options.
v3.5.4 (2014-10-06)
- Fixed date picker to use UTC dates.
- Fixed time picker to use local times.
- Fixed color for “clear” button on time picker.
- Translations fixes for Italian, Slovak, French, Russian, Spanish
v3.5.3 (2014-07-06)
- Fixed time offset issues by using UTC based times.
- Fixed the main files in bower.json.
- Added French accessibility labels.
- Fixed flickering on webkit browsers.
- Fixed adding an ID to the hidden element to remain unique.
- Added Common JS to UMD.
- Allow clear method to be muted.
- Updated docs the trigger method’s ability to pass data to event callbacks.
- Fixed “today” button to be disabled when the date is disabled.
v3.5.1 (2014-05-16)
- Fixed Nepali translations.
- Fixed month nav pointer styling with Bootstrap (border-box issue).
- Fixed scrollbar width checker.
- Fixed picker.get('select', 'yyyy-mm-dd') when select is null.
- Added Vietnamese translations.
- Fixed enabling date when firstDay is set.
- Fixed $ conflict in Arabic translations.
- Improved differentiation between “selected” and “highlighted” dates/times.
v3.5.0 (2014-04-14)
- Fixed page scrolling issue when modal view is open in the default theme.
- Fixed Hungarian translations typo.
- Fixed issue with script freezing when min is true and “today” is disabled.
- Fixed parsing months as 1-indexed when value is a string.
- Improved Grunt script to build a cleaner project.
- Fixed alternate API syntax not returning the correct value.
- Added a note on how to appropriately use the yy format.
- Added Nepali translations.
- Added the hiddenName option to use the visible input’s name as the hidden input’s name.
- Added missing semi-colon to legacy.js.
- Year selector appears before the month selector.
- Fixed issue where the clear method did not reset the select value to null.
- Added a note on how to use a separate button to open/close the picker.
v3.4.0 (2014-02-16)
- added a new feature so you can sARIA support added. :star2:
- Fixed: Date parser recognizes a string value and uses month index as 1.
- Fixed: Date and time parser fall back to default format if none is specified.
- Fixed set('disable', true) crashing with max: true in options.
- Fixed: Fixed time picker not parsing midnight correcly.
- Fixed Firefox bug with querying for active element with $.contains.
- Fixed month selector navigation from month with more days to one with less.
- Fixed issue where right-clicks caused picker to close in Firefox.
- Fixed IE issue with month & year selector not working correctly.
- Improved time picker setting a time relative to “now”.
- Improved disabling/enabling dates and times.
- Spanish translations typo fixed.
- Added the off method.
- Added Galician translations.
- Added Slovenian translations.
- Added Icelandic translations.
- Added option to disable dates & times within a range.
- Added option to set the select, highlight, and view using a string and parsing format.
- Added some performance improvents.
- Added more tests and documentation.
- Fixed picker.get('select') when there’s no value.pecify a set of animations
v3.3.2 (2013-12-05)
- Adjusted font size for narrow screens.
- Fixed select menu click on Firefox.
- Fixed issue with stop method called within onClose.
- Fixed issue with value not being parsed when formatSubmit is used.
v3.3.1 (2013-12-05)
- Fixed border from preventing picker from opening.
- Added option to enable dates/times disabled within a range.
- Added traditional Chinese.
- Fixed jQuery Mobile and MagnificPopup click issues.
- Fixed setting min limit on time picker.
- Fixed Firefox and IE bug for finding activeElement.
- Added option to set things with muted callbacks.
- Fixed French translations capitalization.
- Fixed time picker scrolling.
- Added setting a time using a native JavaScript date objects.
- Added option to keep an editable input element.
v3.3.0 (2013-10-14)
- Improved disabled dates validation.
- Fixed transparency issue in IE8 on XP.
- Added functionality to reset disabled dates/times.
- Dropdown styling tweaked.
- Fixed issue with forms not submitting on Firefox.
- Fixed issue with selected time scrolling into view.
- Added hiddenPrefix option for hidden input element’s name attribute.
- Fixed issue with passing focus to an element with custom jQuery builds.
- Resolved jQuery conflict.
- Fixed issue with time picker intervals and the min selectable time.
- Added option to disabled/enable dates using JavaScript Date objects.
- Tweaked functionality in enabling/disabling dates and times.
- Improved support for RTL languages and keyboard navigation.
- Added rtl.css for styling RTL languages appropriatey.
v3.2.2 (2013-9-20)
- Added generic Arabic translations.
- Fixed jQuery conflict in picker extension files.
- Time picker “disabled” attribute fix.
- Fixed issue with IE losing key bindings when clicked within picker.
- Improved delegated click handling on picker elements.
v3.2.1 (2013-8-25)
- bugs fixed
v3.1.2 (2013-7-7)
- Fixed month navigation with disabled dates.
v3.1.1 (2013-6-29)
- Corrected “no-drop” cursor on input element for certain browsers.
- Fixed CSS for disabled dates with unfocused input.
- Corrected unescaped translations.
v3.1.0 (2013-6-23)
- Fix for freezing with unexpected date format.
- Fix for “mm” and “m” formats opening with incorrect month.
- Border styling adjusted for disabled times.
v3.0.4 (2013-5-26)
- Fix for using
firstDay
with month starting on Sunday. - Improved disabled dates validation.
v3.0.5 (2013-6-15)
- Fix for getFirstWordLength not being defined.
- Corrected Norwegian translation.
This awesome jQuery plugin is developed by amsul. For more Advanced Usages, please check the demo page or visit the official website.