Elegant Context Menu Inspired By Material Design
File Size: | 20.8 KB |
---|---|
Views Total: | 1246 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
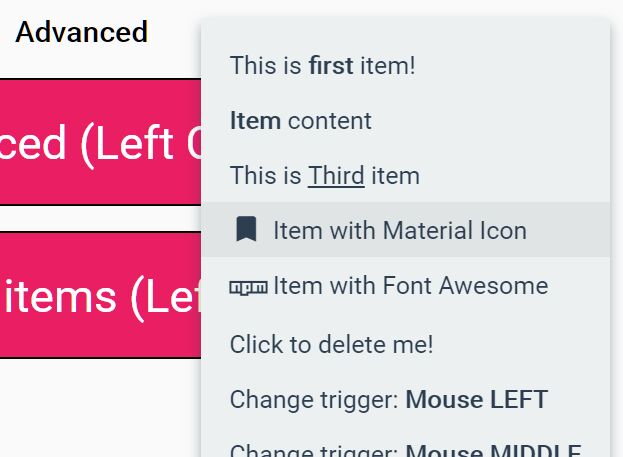
This is a jQuery plugin that creates an animated, Android (Material Design) inspired popup menu to replace the native browser context (right-click) menu on any element within the document.
More Features:
- Smooth open/close animations based on CSS transitions.
- Semantic markup and SEO-friendly.
- Custom trigger events: right click, left click, middle click, etc.
- Allows you dynamically add items to the context menu.
- Easy to customize the styles with SCSS variables.
- Easy to implement without any JS call.
How to use it:
1. Load the complied stylesheet in the header of the document.
<link rel="stylesheet" href="./dist/jquery.context-menu.min.css" />
2. Insert menu items into an HTML unordered list named 'context-menu-content'.
<ul class="context-menu-content"> <li> <a href="#"> Menu 1 </a> </li> <li> <a href="#"> Menu 2 </a> </li> <li> <a href="#"> Menu 3 </a> </li> ... </ul>
3. Insert the menu list into a container in which you want to display the context menu and then determine the trigger event using the following CSS classes:
- .context-menu-trigger: required CSS class
- .context-menu-button-right: right click
- .context-menu-button-middle: middle click
<div class="context-menu-trigger context-menu-button-right"> <ul class="context-menu-content"> <li> <a href="#"> Menu 1 </a> </li> <li> <a href="#"> Menu 2 </a> </li> <li> <a href="#"> Menu 3 </a> </li> ... </ul> Left Click Menu </div>
4. Load jQuery library and the jQuery context-menu.js script at the end of the document. That's it.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="./dist/jquery.context-menu.min.js"></script>
5. You can also append the context menu to a container using JavaScript:
// create a new context menu instance var contextMenu = $('#your-container').contextMenu(); // determine the trigger event contextMenu.button = mouseButton.LEFT; // add an item to the context menu contextMenu.menu().addItem('This is <b>first</b> item!'); // add an item with callback function contextMenu.menu().addItem('This is <u>Third</u> item', function () { alert('Hello world!'); }); // remove an item contextMenu.menu().addItem('Click to delete me!', function () { contextMenu.menu().removeItem(1); }); // change trigger button contextMenu.menu().addItem('Change trigger: <b>Mouse LEFT</b>', function () { contextMenu.button = mouseButton.LEFT; contextMenu.update(); }); // event handlers // onclick contextMenu.menu().addItem('onclick event', function () { contextMenu.onclick = function () { $('#log').append('You are clicked!<br>'); this.close(); } }); // onopen contextMenu.menu().addItem('onopen event', function () { contextMenu.onopen = function () { $('#log').append('Context menu opened!<br>'); } }); // initialize the context menu contextMenu.init();
6. To customize the styles, override the default variables in the jquery.context-menu.scss
as follows:
$context-menu-font-family: -apple-system , BlinkMacSystemFont , "Segoe UI" , Roboto, Helvetica, Arial, sans-serif !default; $context-menu-font-weight: 400 !default; $context-menu-margin: 4px !default; $context-menu-padding: 12px 0px !default; $context-menu-border: none !default; $context-menu-border-radius: 3px !default; $context-menu-background: #ecf0f1 !default; $context-menu-foreground: #2c3e50 !default; $context-menu-shadow: 0px 4px 8px rgb(44, 62, 80, 0.4) !default; $context-menu-min-width: 140px !default; $context-menu-max-width: 280px !default; $context-menu-max-height: 480px !default; $context-menu-item-margin: 0px !default; $context-menu-item-padding: 8px 18px !default; $context-menu-item-cursor: pointer !default; $context-menu-item-background: $context-menu-background !default; $context-menu-item-foreground: $context-menu-foreground !default; $context-menu-item-hover-background: rgba(0, 0, 0, 0.05) !default; $context-menu-item-hover-foreground: $context-menu-foreground !default; $context-menu-item-transition: none !default;
This awesome jQuery plugin is developed by isaeken. For more Advanced Usages, please check the demo page or visit the official website.