AJAX Note Taking Web App With jQuery and PHP
File Size: | 64.8 KB |
---|---|
Views Total: | 3239 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
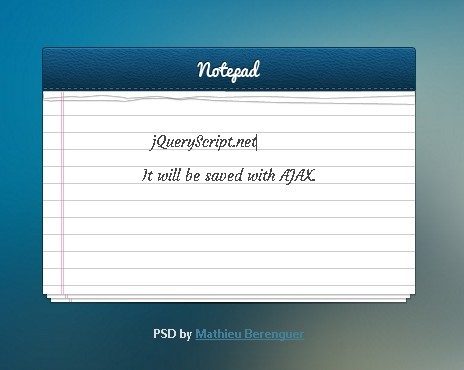
AJAX Note Taking Web App built with jQuery and PHP that allows your vistors write notes which can be auto saved into a text file with AJAX.
How to use it:
1. Markup
<textarea id="note"><?php echo $note_content ?></textarea>
2. Include jQuery Library
<script src="http://code.jquery.com/jquery-1.9.0.min.js"></script>
3. Javascript for ajax save notes
$(function(){ var note = $('#note'); var saveTimer, lineHeight = parseInt(note.css('line-height')), minHeight = parseInt(note.css('min-height')), lastHeight = minHeight, newHeight = 0, newLines = 0; var countLinesRegex = new RegExp('\n','g'); // The input event is triggered on key press-es, // cut/paste and even on undo/redo. note.on('input',function(e){ // Clearing the timeout prevents // saving on every key press clearTimeout(saveTimer); saveTimer = setTimeout(ajaxSaveNote, 2000); // Count the number of new lines newLines = note.val().match(countLinesRegex); if(!newLines){ newLines = []; } // Increase the height of the note (if needed) newHeight = Math.max((newLines.length + 1)*lineHeight, minHeight); // This will increase/decrease the height only once per change if(newHeight != lastHeight){ note.height(newHeight); lastHeight = newHeight; } }).trigger('input'); // This line will resize the note on page load function ajaxSaveNote(){ // Trigger an AJAX POST request to save the note $.post('index.php', { 'note' : note.val() }); } });
4. PHP
<?php $note_name = 'note.txt'; $uniqueNotePerIP = true; if($uniqueNotePerIP){ // Use the user's IP as the name of the note. // This is useful when you have many people // using the app simultaneously. if(isset($_SERVER['HTTP_X_FORWARDED_FOR'])){ $note_name = 'notes/'.$_SERVER['HTTP_X_FORWARDED_FOR'].'.txt'; } else{ $note_name = 'notes/'.$_SERVER['REMOTE_ADDR'].'.txt'; } } if(isset($_SERVER['HTTP_X_REQUESTED_WITH'])){ // This is an AJAX request if(isset($_POST['note'])){ // Write the file to disk file_put_contents($note_name, $_POST['note']); echo '{"saved":1}'; } exit; } $note_content = ''; if( file_exists($note_name) ){ $note_content = htmlspecialchars( file_get_contents($note_name) ); } ?>
This awesome jQuery plugin is developed by tutorialzine. For more Advanced Usages, please check the demo page or visit the official website.