Create A Simple Vertical Timeline with jQuery and CSS
File Size: | 3.01 KB |
---|---|
Views Total: | 20023 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
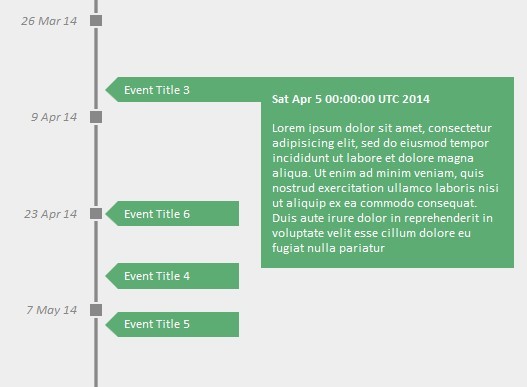
A simple, lightweight, vertical and animated jQuery & jQuery UI timeline widget which allows expanding the event markers to see more details.
How to use it:
1. Include the required jQuery library and jQuery UI in the Html page.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/jqueryui/1.10.4/jquery-ui.min.js"></script>
2. Create a container element to place the timeline.
<div id="testTimeline"> </div>
3. Create events for the timeline using Javascript array object.
$(function(){ $("#testTimeline").timeline({ data: [ {date: new Date(2014,6,06), type: "someType", title: "Event Title 1", description: "Description 1"}, {date: new Date(2014,2,14), type: "someType", title: "Event Title 2", description: "Description 2"}, {date: new Date(2013,1,01), type: "someType", title: "Event Title 3", description: "Description 3"} ], height: 600 // in pixel }); });
4. The Javascript to draw a timeline in the container 'testTimeline'.
$.widget('pi.timeline', { options: { data: [ {date: new Date(), type:"Type1", title:"Title1", description:"Description1"}, {date: new Date(), type:"Type2", title:"Title2", description:"Description2"} ], types: [ {name:"Type1", color:"#00ff00"}, {name:"Type2", color:"#0000ff"} ], display: "auto", height: 600 }, _create: function(){ this._refresh(); }, _refresh: function(){ var miliConstant = 86400000 var firstDate = this.options.data[0].date; var lastDate = this.options.data[0].date; for (i=0;i<this.options.data.length;i++) { if (this.options.data[i].date > lastDate) { lastDate = this.options.data[i].date; } else if (this.options.data[i].date < firstDate) { firstDate = this.options.data[i].date; } } var dayRange = (lastDate - firstDate) / miliConstant; var segSpace = Math.floor(this.options.height / (dayRange / 7)); var segLength = 7; if (segSpace < 80) { var segSpace = Math.floor(this.options.height / (dayRange / 14)); segLength = 14; } if (segSpace < 80) { var segSpace = Math.floor(this.options.height / (dayRange / 28)); segLength = 28; } if (segSpace < 80) { var segSpace = Math.floor(this.options.height / (dayRange / 56)); segLength = 56; } if (segSpace < 80) { var segSpace = Math.floor(this.options.height / (dayRange / 112)); segLength = 112; } var majorCount = Math.floor(this.options.height / segSpace) + 1; //Empty Current Element this.element.empty(); //Draw TimeLine this.element.append("<div class='tlLine' style='height: " + this.options.height + "px;'></div>") //Draw Major Markers var tempDate = new Date(firstDate.getTime()); for (i=0;i<majorCount;i++) { this.element.append("<div class='tlMajor' style='top: " + ((segSpace * i) - 7) + "px;'></div>"); this.element.append("<span class='tlDateLabel' style='top: " + ((segSpace * i) - 7) + "px;'>" + $.datepicker.formatDate( "d M y", tempDate) + "</span>"); tempDate.setDate(tempDate.getDate() + segLength); } //draw event markers for (i=0;i<this.options.data.length;i++) { var dayPixels = ((this.options.data[i].date - firstDate) / (lastDate - firstDate)) * this.options.height; //alert((this.options.data[i].date - firstDate) + ", " + (lastDate - firstDate) + ", " +dayPixels); this.element.append("<div class='tlDateDot' style='top: " + (dayPixels - 11) + "px;'></div>"); this.element.append("<div class='tlEventFlag' style='top: " + (dayPixels - 11) + "px;'>" + this.options.data[i].title + "</div>"); this.element.append("<div class='tlEventExpand' style='top: " + (dayPixels - 11) + "px;'><p><b>" + this.options.data[i].date + "</b></p><p>" + this.options.data[i].description + "<p></div>"); } $(".tlEventExpand").hide(); //$(".tlEventExpand").hide(); $(".tlEventFlag").click(function(){ var tempThis = $(this); $(".tlEventExpand").hide(); $(".tlEventFlag").animate({width:'100px'}, 200); if (tempThis.hasClass('active')) { tempThis.removeClass('active'); } else { $(".tlEventFlag").removeClass('active'); tempThis.addClass('active'); tempThis.animate({width:'120px'}, 200, function(){ tempThis.next('div').show(); }); } }); }, _destroy: function() {}, _setOptions: function() {}
5. The sample CSS to style your timeline.
#testTimeline { margin: 20px; position: relative; } .tlMajor { left: 80px; position: absolute; width: 10px; height: 10px; background-color: #888; border: 2px solid #eee; } .tlDateLabel { position: absolute; font-size: 12px; color: #888; text-align: right; font-style: italic; left: 0px; width: 70px; } .tlLine { position: absolute; width: 2px; background-color: #888; top: 0px; left: 85px; border: 1px solid #aaa; } .tlDateDot { left: 95px; position: absolute; width: 0; height: 0; border-top: 11px solid transparent; border-bottom: 11px solid transparent; border-right: 11px solid #5cac73; } .tlEventFlag { left: 106px; position: absolute; width: 100px; height: 14px; background-color: #5cac73; font-size: 12px; padding: 4px 0px 4px 5px; color: #fff; cursor: pointer; } .tlEventExpand { left: 230px; position: absolute; width: 200px; background-color: #5cac73; font-size: 12px; padding: 0 10px 0 10px; color: #fff; }
This awesome jQuery plugin is developed by BradGamma. For more Advanced Usages, please check the demo page or visit the official website.