Mobile-friendly Numeric Keypad Plugin For jQuery - NumPad
File Size: | 12.9 KB |
---|---|
Views Total: | 31887 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
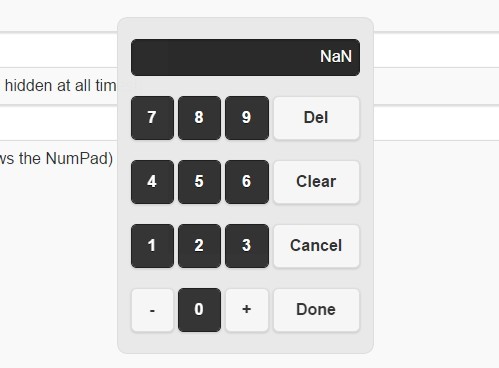
NumPad is a jQuery plugin used to create mobile-friendly numeric keypads for text fields, DIV elements or table columns. Works perfectly with any frameworks such as jQuery mobile, Bootstrap and much more.
Basic usage:
1. Load jQuery library and the jQuery NumPad plugin's files in your project.
<link rel="stylesheet" href="path/to/jquery.numpad.css"> <script src="//code.jquery.com/jquery-1.11.2.min.js"></script> <script src="path/to/jquery.numpad.js"></script>
2. Include the jQuery Mobile to implement the Numpad plugin on your mobile project.
<link rel="stylesheet" href="//code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css"> <script src="//code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
3. Set NumPad defaults for jQuery mobile. These defaults will be applied to all NumPads within this document.
$.fn.numpad.defaults.gridTpl = '<table class="ui-bar-a"></table>'; $.fn.numpad.defaults.backgroundTpl = '<div class="ui-popup-screen ui-overlay-a"></div>'; $.fn.numpad.defaults.displayTpl = '<input data-theme="b" type="text" />'; $.fn.numpad.defaults.buttonNumberTpl = '<a data-role="button" data-theme="b"></a>'; $.fn.numpad.defaults.buttonFunctionTpl = '<a data-role="button" data-theme="a"></a>'; $.fn.numpad.defaults.onKeypadCreate = function(){$(this).enhanceWithin();};
4. Instantiate NumPad once the page is ready to be shown.
(document).on('pageshow', function(){ // for text input $('#text').numpad(); // for password field $('#password').numpad({ displayTpl: '<input data-theme="b" type="password">' }); // for numpad button $('#numpadButton-btn').numpad({ target: $('#numpadButton'), }); // for div element $('#numpad4div').numpad(); // for table columns $('#numpad4column').numpad(); });
5. Options and defaults.
// Target jQuery element target: false, // Name of the event to trigger opening the numpad openOnEvent: 'click', // Template for the background overlay behind the Keypad backgroundTpl: '<div></div>', // Template for the keypad grid gridTpl: '<table></table>', // Template for the display field above the keypad displayTpl: '<input type="number" />', // Template for the grid cell, where the display field is located displayCellTpl: '<td colspan="4"></td>', // Template for each row of the grid rowTpl: '<tr></tr>', // Template for each regular cell of the grid cellTpl: '<td></td>', // Template for the number buttons buttonNumberTpl: '<button></button>', // Template for the functional buttons (like clear, done, etc.) buttonFunctionTpl: '<button></button>', // Grid table CSS class gridTableClass: '', // Text for buttons textDone: 'Done', textDelete: 'Del', textClear: 'Clear', textCancel: 'Cancel', // Target element the keypad should be appended to appendKeypadTo: false, // Positions position: 'fixed', positionX: 'center', positionY: 'middle', // Called once a numpad is created onKeypadCreate: false // Called once a numpad is opened onKeypadOpen: false, // Called once a numpad is closed onKeypadClose: false, // Called everytime the user changes the value in the display of the numpad by pressing a virtual button. onChange: false
Change logs:
2016-09-16
- FIX rounded borders bug in Bootstrap
- FIX display shows NAN if attached to a non-input
2016-02-13
- NEW methods to open/close numpad programmatically
2015-07-30
- v1.3.1: minor fixes.
2015-07-23
- v1.3: Fractions and negative numbers
2015-07-17
- NEW: onChange callback and change event for target
- Fixed maxlength html property
2015-07-08
- Added
onKeypadOpen
andonKeypadClose
callbacks and correspondingnumpad.open
andnumpad.close
events
2015-03-26
- Bootstrap demo + minor fixes
This awesome jQuery plugin is developed by kabachello. For more Advanced Usages, please check the demo page or visit the official website.