Dynamic Tree Selection Control In jQuery - ArtaraxTreeView
File Size: | 10 KB |
---|---|
Views Total: | 4225 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
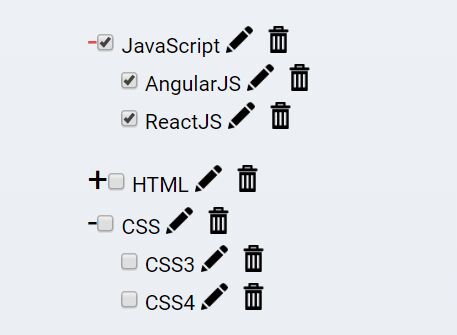
ArtaraxTreeView is a flexible, configurable tree plugin to dynamically render a tree selection control from a JSON/JS array for selecting parent/child nodes.
The tree is collapsible, expandable and checkable. You can also update/delete specified nodes using callback functions.
How to use it:
1. Insert jQuery JavaScript library and the jQuery ArtaraxTreeView plugin into your html page.
<link rel="stylesheet" href="/path/to/jquery.artaraxtreeview.css"> <script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/jquery.artaraxtreeview.js"></script>
2. Create an empty HTML list for the tree.
<ul class="treeview"></ul>
3. Prepare your hierarchical data for the tree.
var treeViewData = [ { 'Id' : 1, 'Title' : 'My Tree','ParentId' : null }, { 'Id' : 2, 'Title' : 'JavaScript', 'ParentId' : 1 }, { 'Id' : 3, 'Title' : 'HTML', 'ParentId' : 1 }, { 'Id' : 4, 'Title' : 'CSS', 'ParentId' : 1 }, { 'Id' : 5, 'Title' : 'AngularJS', 'ParentId' : 2 }, { 'Id' : 6, 'Title' : 'ReactJS', 'ParentId' : 2 }, { 'Id' : 7, 'Title' : 'HTML5', 'ParentId' : 3 }, { 'Id' : 8, 'Title' : 'CSS3', 'ParentId' : 4 }, { 'Id' : 9, 'Title' : 'CSS4', 'ParentId' : 4 } ];
4. Initialize the tree.
var artaraxTreeView = $.artaraxTreeView({ jsonData: treeViewData });
5. Render the tree inside the HTML list.
artaraxTreeView.loadTreeViewOnInsert(1); // for update mode artaraxTreeView.loadTreeViewOnUpdate(1);
6. Set the pre-selected nodes (only workss on update mode).
var artaraxTreeView = $.artaraxTreeView({ selectedIds: [7,8,9] });
7. Decide whether to display children nodes.
var artaraxTreeView = $.artaraxTreeView({ isDisplayChildren: true // default });
8. Set the mode of the tree.
var artaraxTreeView = $.artaraxTreeView({ mode: "deletable,updatable,autoSelectChildren" });
9. Callback functions available.
var artaraxTreeView = $.artaraxTreeView({ updateCallBack: function(obj){ // do something }, deleteCallBack: function(obj){ // do something } });
10. Get selected nodes.
var selectedIds = artaraxTreeView.getSelectedIds(); alert(JSON.stringify(selectedIds));
11. Remove all the chekced nodes.
artaraxTreeView.unCheckedAll();
Changelog:
2020-06-28
- Improvement.
2018-09-28
- JS Update
This awesome jQuery plugin is developed by H-Moradof. For more Advanced Usages, please check the demo page or visit the official website.