Animated Expandable Message Box In jQuery
File Size: | 3.43 KB |
---|---|
Views Total: | 279 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
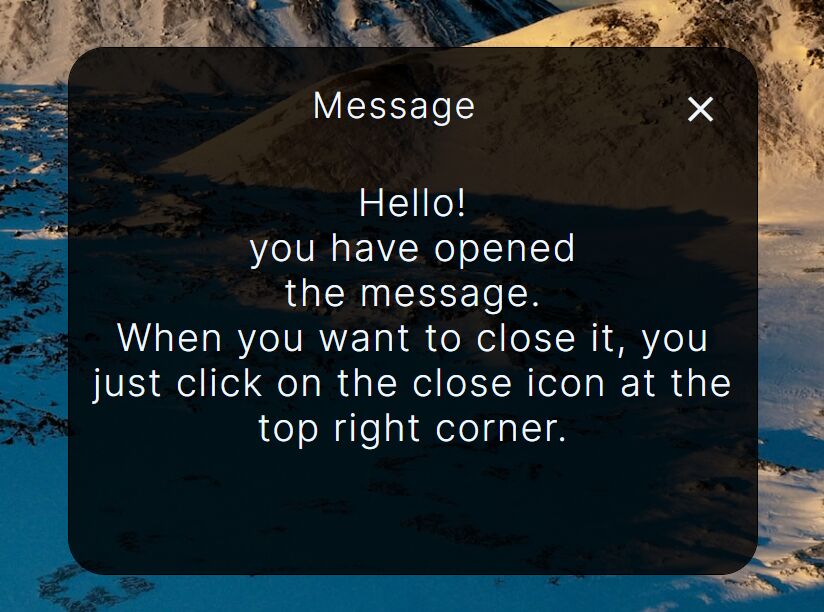
An animated and expandable message box written in jQuery that allows users to quickly preview a message before diving into the details.
With a simple click, users can quickly get the full context without having to navigate to another page.
How to use it:
1. Add a message box to the webpage.
<div id="message-box"> <!-- Message Box --> <div id="header"> <p id="message">Brief Message Here</p> <!-- Close Button --> <span id="close">×</span> </div> <!-- Detailed Message Here --> <div id="content"> <p>More Content Here</p> </div> </div>
2. Apply CSS styles to the message box.
#message-box{ background: navy; color: aliceblue; text-align: center; width: 30%; border-radius: 20px; background: rgb(0, 0, 0, 0.5); border: solid 1px #000; } #header{ height: 20px; padding: 20px; display: flex; justify-content: center; align-items: center; } #message{ flex: 1; font-size: larger; letter-spacing: 1px; } #content{ height: 200px; padding: 10px; display: none; font-size: 20px; letter-spacing: 1px; } #close{ cursor: pointer; font-size: 30px; } #close:hover{ color: darkgrey; }
3. Load the necessary jQuery library at the end of the document.
<script src="/path/to/cdn/jquery.min.js"></script>
4. Expand the message box into a detailed view with a single click.
$(function(){ $("#header").click(function(){ $("#content").toggle("fast"); $("#warn").hide("fast"); }); $("#close").click(function(){ $("#message-box").hide("fast"); }); });
This awesome jQuery plugin is developed by slhinanc. For more Advanced Usages, please check the demo page or visit the official website.