jQuery Based Youtube/Vimeo/HTML5 Video Controller - Video.js
File Size: | 16.3 KB |
---|---|
Views Total: | 6316 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
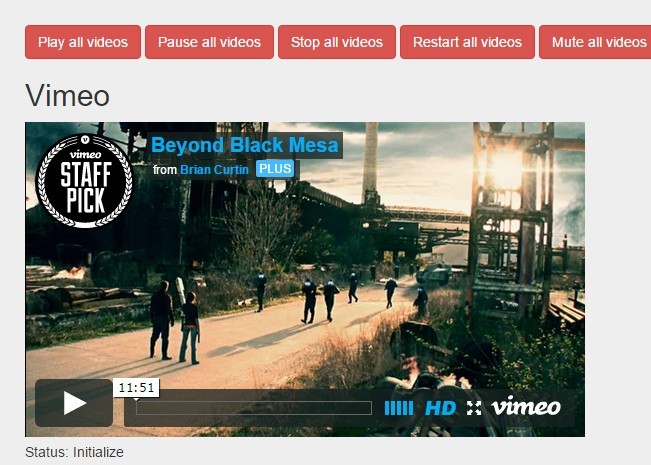
A jQuery video plugin that provides useful methods and events to control Youtube, Vimeo, and HTML5 videos from JavaScript.
Basic usage:
1. Include jQuery library and the jQuery Video Controller plugin at the bottom of the html page.
<script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/jquery.video.min.js"></script>
2. Add your videos into the web page.
<iframe class="video" src="YOUTUBE/VIMEO VIDEO"></iframe> <video class="video" controls=""> <source src="video.mp4" type="video/mp4"> <source src="video.webm" type="video/webm"> </video>
3. Initialize the plugin and we're ready to go.
$('.video').video({ // options attr_ready: 'data-video-ready', attr_playing: 'data-video-playing', attr_paused: 'data-video-paused', });
4. Play all videos.
$('.video').playVideo();
5. Pause all videos.
$('.video').pauseVideo();
6. Stop all videos.
$('.video').stopVideo();
7. Restart all videos.
$('.video').restartVideo();
8. Mute all videos.
$('.video').muteVideo();
9. Unmute all videos.
$('.video').unmuteVideo();
10. Seek to 20 sec.
$('.video').seekToVideo(30);
11. Add events to videos.
$('.video').addVideoEvent('ready', function(e, $video, video_type) { // Triggers if a video is ready. }); $('.video').addVideoEvent('play', function(e, $video, video_type) { // Triggers on play or resume }); $('.video').addVideoEvent('pause', function(e, $video, video_type) { // Triggers on pause }); $('.video').addVideoEvent('finish', function(e, $video, video_type) { // Triggers if a video is finished }); $('.video').addVideoEvent('destroy', function(e, $video, video_type) { // Triggers if the plugin is destroyed });
12. Global settings.
// The suffix for all the video events (for unique purposes) $.video.event_suffix = '_video'; // An array with callbacks for the onYouTubeIframeAPIReady() call $.video.youtube_api_ready_callbacks = [], // The youtube iframe api url $.video.youtube_iframe_api = 'https://www.youtube.com/iframe_api'
Changelog:
v0.1.4 (2023-03-08)
- fix for missing video configuration
This awesome jQuery plugin is developed by faktorvier. For more Advanced Usages, please check the demo page or visit the official website.