jQuery Plugin For iOS-like Swipe To Delete - swipeTo
File Size: | 10 KB |
---|---|
Views Total: | 15142 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
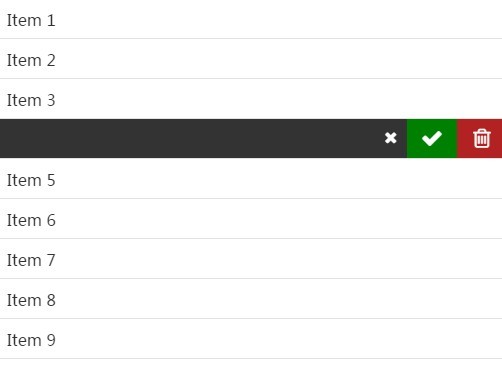
swipeTo is a small jQuery plugin to emulate iOS swipe to delete functionality which displays a group of action buttons as the visitor swipe on a list view. Works only on touch devices which support swipe events.
How to use it:
1. Include Font Awesome 4 for the action button icons.
<link rel="stylesheet" href="//netdna.bootstrapcdn.com/font-awesome/4.3.0/css/font-awesome.min.css">
2. Create a list of items with action buttons.
<div class="list"> <div class="item"> <a href="#" class="item-swipe">Item 1</a> <div class="item-back"> <button class="action first btn-delete" type="button"> <i class="fa fa-trash-o"></i> </button> <button class="action second btn-check" type="button"> <i class="fa fa-check"></i> </button> </div> </div> <div class="item"> <a href="#" class="item-swipe">Item 2</a> <div class="item-back"> <button class="action first btn-delete" type="button"> <i class="fa fa-trash-o"></i> </button> <button class="action second btn-check" type="button"> <i class="fa fa-check"></i> </button> </div> </div> <div class="item"> <a href="#" class="item-swipe">Item 3</a> <div class="item-back"> <button class="action first btn-delete" type="button"> <i class="fa fa-trash-o"></i> </button> <button class="action second btn-check" type="button"> <i class="fa fa-check"></i> </button> </div> </div> ... </div>
3. The sample CSS styles for the list view.
.list { width: 100%; overflow: hidden; } .list .item { border-bottom: 1px solid #E1E1E1; position: relative; height: 40px; } .list .item .item-swipe { background: #ffffff; position: absolute; top: 0; right: 0; bottom: 0; left: 0; padding: 10px; z-index: 1; } .list .item a.item-swipe { display: block; height: 100%; position: relative; text-decoration: inherit; color: #333; } .list .item a.item-swipe:active { color: inherit; } .list .item .item-swipe.open { background: #333; } .list .item .item-swipe.open:before { content: "\f00d"; font-family: FontAwesome; font-style: normal; font-weight: normal; text-decoration: inherit; color: #fff; position: absolute; right: 10px; } .list .item .item-back { background: #E1E1E1; position: absolute; top: 0; right: 0; bottom: 0; left: 0; padding: 10px; text-align: right; } .list .item .item-back .action { position: absolute; top: 0; bottom: 0; right: 0; width: 50px; border: inherit; outline: inherit; color: #fff; padding: 0; font-size: 22px; } .list .item .item-back .action.first { right: 0; } .list .item .item-back .action.second { right: 50px; } .list .item .item-swipe.swiped { -webkit-transition: all .1s linear; -ms-transition: all .1s linear; -moz-transition: all .1s linear; -o-transition: all .1s linear; transition: all .1s linear; } .btn-delete { background: firebrick; } .btn-check { background: green; }
4. Include jQuery library and the jQuery swipeTo plugin at the bottom of the web page.
<script src="//code.jquery.com/jquery-1.11.2.min.js"></script> <script src="swipeTo.js"></script>
5. Enable the swipe to delete functionality on the list view.
$(function() { $('.item-swipe').swipeTo({ //default options minSwipe: 100, angle: 10, binder: true, wrapScroll: 'body', //callback functions swipeStart: function() { console.log('start'); }, swipeMove: function() { console.log('move'); }, swipeEnd: function() { console.log('end'); }, }); deleteItem(); }) // function to delete items var deleteItem = function() { var deleteItemFnc = $('body').on('click tap', '.btn-delete', function(e) { e.preventDefault(); var that = $(this); that.parent().parent().fadeOut('500'); }) }
This awesome jQuery plugin is developed by Ipno84. For more Advanced Usages, please check the demo page or visit the official website.