Simple jQuery Plugin For Google Places Autocomplete - autoPlace.js
File Size: | 6.05 KB |
---|---|
Views Total: | 6734 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
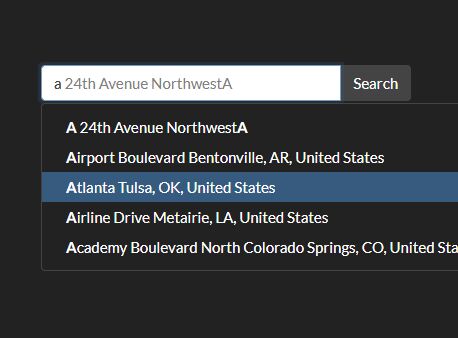
autoPlace.js is a jQuery plugin which adds the Google Places autocomplete and location picker functionalities to a normal text input. Heavily based on the Google Places API.
How to use it:
1. To use this plugin, make sure the latest version of jQuery library and Google Places API are loaded in the document.
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity="sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin="anonymous"></script> <script src="https://maps.googleapis.com/maps/api/js?v=3.exp&libraries=places"></script>
2. Download and load the autoPlace.js
script after jQuery library.
<script src="jquery.autoplace.js"></script>
3. Create a text field for the location picker.
<div class="wrapper"> <input type="text" placeholder="Enter an Address" autocomplete="off" autocorrect="off" autocapitalize="off" spellcheck="false" id="autoplace"> <div class="suggestion-wrapper"> <input type="text" class="suggestion" tabindex="-1"> </div> </div>
4. Enable the plugin.
$('#autoplace').autoplace({ types: ['address'], componentRestrictions: {country: 'us'}, });
5. Get and place the user selected places in the suggestion
field.
$('#autoplace').on('autoplace-suggestion', function(e) { var suggestion = $(e.target).attr('data-autoplace-corrected-suggestion'); $('.suggestion').val(suggestion); });
6. All possible options. For more information, visit Google Maps JavaScript API V3 Reference.
$('#autoplace').autoplace({ // The area in which to search for places. bounds: LatLngBounds|LatLngBoundsLiteral // The types of predictions to be returned. // If nothing is specified, all types are returned. // In general only a single type is allowed. // The exception is that you can safely mix the 'geocode' and 'establishment' types, but note that this will have the same effect as specifying no types. types: ['address'], // The component restrictions. // Component restrictions are used to restrict predictions to only those within the parent component. // E.g., the country. componentRestrictions: {country: 'us'}, // Whether to retrieve only Place IDs. // The PlaceResult made available when the place_changed event is fired will only have the place_id, types and name fields, with the place_id, types and description returned by the Autocomplete service. placeIdOnly: false, // A boolean value, indicating that the Autocomplete widget should only return those places that are inside the bounds of the Autocomplete widget at the time the query is sent. // Setting strictBounds to false (which is the default) will make the results biased towards, but not restricted to, places contained within the bounds. strictBounds: true });
This awesome jQuery plugin is developed by tristanmills. For more Advanced Usages, please check the demo page or visit the official website.