Easy URL Query String Manipulation Plugin - jQuery querystring.js
File Size: | 13.1 KB |
---|---|
Views Total: | 456 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
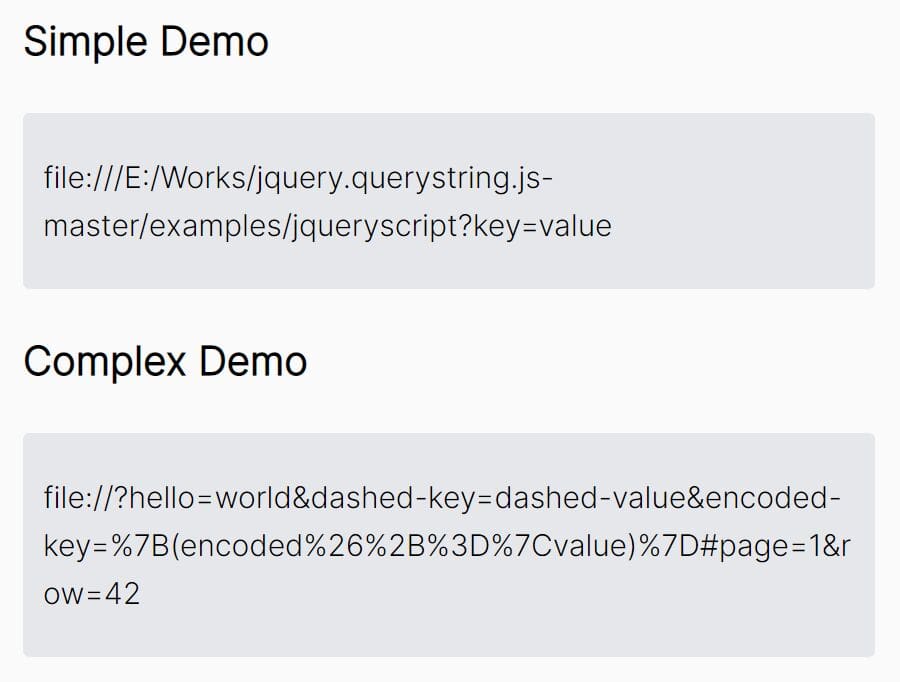
Query strings (also known as URL query parameters) are used in multiple places throughout websites and web applications. They allow adding extra information to the end of a URL(request), and can be used for many different things, such as passing state between requests within your application or even for user identification.
In this article I'll introduce you to a tiny jQuery plugin that you can use to easily dynamically add/modify/delete/get query strings using plain JavaScript. It supports standard query strings, non-standard slash query strings, and hash strings.
See Also:
- Manipulate Data In URL Parameters - jQuery gp-link
- Add Custom Parameter To URL - jQuery PushDataToUrl
- Manipulate Query Parameters Of URL In JavaScript – vanilla-js-url
- Set & Get Query Parameters In URL – hashparser
- Get URL Parameter Values With jQuery - Getjs
How to use it:
1. To get started, include the jquery.querystring.min.js
script after jQuery library.
<script src="/path/to/cdn/jquery.slim.min.js"></script> <script src="/path/to/cdn/jquery.querystring.min.js"></script>
2. Create a new instance of the queryString
// basic var instance = new $.queryString(); // OR var instance = new $.queryString('jqueryscript'); => /path/to/jqueryscript?key=value // OR var instance = $.queryString("https://www.jqueryscript.net"); => https://www.jqueryscript.net/?key=value
3. Set query/hash string parameters.
// set standard parameters instance.params.hello = "world"; instance.params["dashed-key"] = "dashed-value"; instance.params["encoded-key"] = "{(encoded&+=|value)}"; // set hash values instance.hash.page = 1; instance.hash.row = 42;
4. Get all query/hash string parameters.
// get standard parameters var value = instance.params[key]; console.info(key, value); // get hash values var value = instance.hash[key]; console.info(key, value);
5. Delete all query/hash string parameters.
// delete standard parameters delete instance.params.key; // delete hash values delete instance.hash.key;
6. Available options.
var instance = new $.queryString(url, { // encode query string encode: true, // standard, slash format: "standard", // callback encodeCallback: encode, });
This awesome jQuery plugin is developed by godlikemouse. For more Advanced Usages, please check the demo page or visit the official website.