Accordion-style Content Slider With jQuery And CSS
File Size: | 6.7 KB |
---|---|
Views Total: | 2879 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
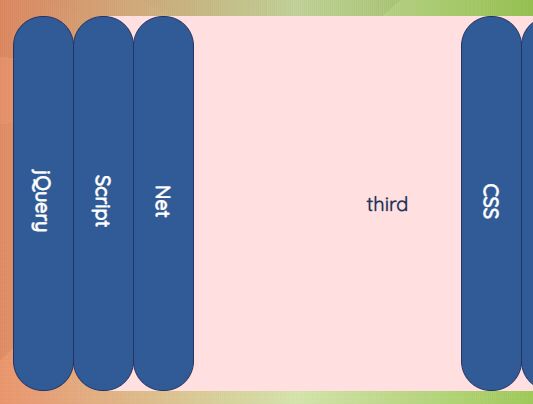
Yet another jQuery accordion slider plugin which allows the user to expand & collapse slides just like an accordion.
Fully responsive and mobile compatible. The responsive layout is based on CSS3 flexbox.
How to use it:
1. Build the HTML structure for the accordion slider.
<div class="slider-wrap"> <div class="slider current"> <button class="index"><span>Accordion 1</span></button> <figure class="img"> Accordion 1 Content </figure> </div> <div class="slider current"> <button class="index"><span>Accordion 2</span></button> <figure class="img"> Accordion 2 Content </figure> </div> <div class="slider current"> <button class="index"><span>Accordion 3</span></button> <figure class="img"> Accordion 3 Content </figure> </div> <div class="slider current"> <button class="index"><span>Accordion 4</span></button> <figure class="img"> Accordion 4 Content </figure> </div> <div class="slider current"> <button class="index"><span>Accordion 5</span></button> <figure class="img"> Accordion 5 Content </figure> </div> ... </div>
2. The basic styling of the accordion slider.
.slider-wrap { width: 100%; height: 300px; background: transparent; position: relative; overflow: hidden; border-radius: 20px; } .slider-wrap .slider { position: absolute; top: 0; display: flex; width: 100%; height: 100%; } .slider-wrap .slider .index { width: 50px; height: 100%; color: #fff; font-weight: bold; border: 1px solid #233567; background: #315b96; border-radius: 25px; } .slider-wrap .slider .index span { -webkit-writing-mode: vertical-rl; -ms-writing-mode: tb-rl; writing-mode: vertical-rl; } .slider-wrap .slider .img { display: flex; justify-content: center; align-items: center; color: #233567; font-weight: bold; width: calc(100% - 40px); border-radius: 25px; background: #ffdfdf; transition: width .7s; } .slider-wrap .slider.current { width: 100%; } .slider-wrap .slider.current .img { width: calc(100% - 40px); }
3. The necessary JavaScript (jQuery script) to enable the accordion slider. Copy the following snippets and paste them into your document, after loading jQuery library.
<script src="/path/to/cdn/jquery.min.js"></script>
const $sliderWrap = $('.slider-wrap'); const $slider = $sliderWrap.find('.slider'); const $index = $slider.find('.index'); const $img = $slider.find('.img'); const sliderNum = $slider.length; let indexWidth = $index.width() + 1; let sliderWrapWidth = $sliderWrap.width(); const duration = 300; $(window).on('resize', function() { sliderWrapWidth = $sliderWrap.width(); console.log(sliderWrapWidth) }); for(let i = 0; i < sliderNum; i++) { $slider.eq(i).css({ left: i * indexWidth, width: sliderWrapWidth - (i * indexWidth) }); } $index.on('click', function() { let num = $index.index(this); let start = sliderWrapWidth - (indexWidth * (sliderNum - num - 1)); for(let i = num; i > 0; i--){ $slider.eq(i).stop().animate({left: indexWidth * i}, duration, 'swing'); } for(let i = num; i < sliderNum; i++) { $slider.eq(i + 1).stop().animate({left: start + (indexWidth * (i - num))}, duration, 'swing'); } })
This awesome jQuery plugin is developed by Yusuke Saio. For more Advanced Usages, please check the demo page or visit the official website.