Feature-rich Accordion Slider In jQuery
File Size: | 121 KB |
---|---|
Views Total: | 5883 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
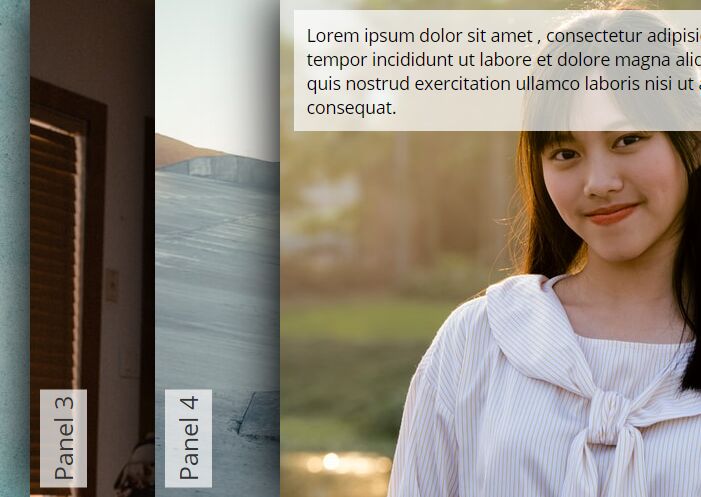
A jQuery plugin that helps you create responsive, touch-friendly, retina-ready accordion sliders for presenting anything on the page.
More Features:
- Image lazy loading.
- Dynamic content loading: JSON or XML.
- Allows multiple layers at the same panel.
- Smooth transitions.
- Pagination bullets.
- Supports Youtube/Vimeo/Sublime/HTML5 videos.
- Keyboard & Mouse interactions.
- Can be integrated with any lightbox plugin like the known FancyBox.
- Supports deep linking.
Table Of Contents:
- Lazy Loading & Retina Support
- Layer Types and Options
- Load Content From JSON
- Load Content From XML
- Smart Videos
- Plugin Options
- API Methods
- Events
How to use it:
1. Install the Accordion Slider jQuery plugin.
<link rel="stylesheet" href="../dist/css/accordion-slider.min.css" /> <script src="/path/to/cdn/jquery.min.js"></script> <script src="./dist/js/jquery.accordionSlider.min.js"></script>
2. Add panels to the accordion slider.
<div id="example" class="accordion-slider"> <div class="as-panels"> <div class="as-panel"> <img class="as-background" src="1.jpg" /> </div> <div class="as-panel"> <img class="as-background" src="2.jpg" /> </div> <div class="as-panel"> <img class="as-background" src="3.jpg" /> </div> <div class="as-panel"> <img class="as-background" src="4.jpg" /> </div> <div class="as-panel"> <img class="as-background" src="5.jpg" /> </div> </div> </div>
3. Initialize the plugin on the parent container to generate a basic accordion slider.
$('#example').accordionSlider({ // options here });
4. Enable lazy loading & high resolution/retina images.
<img class="as-background" src="./dist/css/images/blank.gif" data-src="/path/to/original/image.jpg" data-retina="/path/to/original/[email protected]" />
5. Add multiple layers to accordion panels using the following CSS classes and HTML data
attributes.
- as-opened: The layer will be visible only when the panel is opened.
- as-closed: The layer will be visible only when the panel is closed.
- as-black: Black background.
- as-white: Light background.
- as-padding: Add a 10px padding to the layer.
- as-rounded: Rounded corner.
- as-vertical: Set the layer's orientation to vertical.
- as-background-opened: Swap background images when the panel is opended
- data-width: Width of the layer.
- data-height: Height of the layer.
- data-depth: Z-index CSS property.
- data-position: 'topLeft' (default), 'topRight', 'bottomLeft' or 'bottomRight'
- data-horizontal: Fixed value, percentage value or 'center'.
- data-vertical: The same as 'data-horizontal'.
- data-show-transition: 'left', 'right', 'up' or 'down'.
- data-hide-transition: The same as 'data-show-transition'.
- data-show-offset: Add an offset to the layer.
- data-hide-offset: Add an offset to the layer.
- data-show-duration: The duration of the transition.
- data-hide-duration: The duration of the transition.
- data-show-delay: Transition delay.
- data-hide-delay: Transition delay.
<div class="as-panel"> <a href="http://bqworks.com"> <img class="as-background" src="../dist/css/images/blank.gif" data-src="https://source.unsplash.com/700x500/?summer" data-retina="https://source.unsplash.com/700x500/?summer"/> </a> <div class="as-layer as-closed as-white as-vertical panel-counter" data-position="bottomLeft" data-horizontal="8" data-vertical="8"> Panel 2 </div> <div class="as-layer" data-position="bottomLeft" data-horizontal="20" data-vertical="20" data-width="100%"> <p class="as-layer as-opened as-white as-padding" data-position="bottomLeft" data-show-transition="left" data-hide-transition="up" data-show-delay="400" data-hide-delay="200"> Lorem ipsum </p> <p class="as-layer as-opened as-black as-padding hide-small-screen" data-position="bottomLeft" data-horizontal="120" data-show-transition="left" data-hide-transition="up" data-show-delay="600" data-hide-delay="100"> dolor sit amet </p> <p class="as-layer as-opened as-white as-padding hide-small-screen" data-position="bottomLeft" data-horizontal="245" data-show-transition="left" data-hide-transition="up" data-show-delay="800"> consectetur adipisicing elit. </p> </div> </div>
6. Load content from JSON.
$('#example').accordionSlider({ JSONSource: '/path/to/accordion.json', });
// accordion.json { "accordion": { "panels": [ { "background": {"source": "/path/to/1.jpg"}, "backgroundRetina": {"source": "path/to/[email protected]"}, "backgroundOpened": {"source": "path/to/alt1.jpg"}, "backgroundOpenedRetina": {"source": "path/to/[email protected]"}, "backgroundLink": {"address": "https://jqueryscript.net"}, "layers": [ {"content": "Panel 1", "style": "closed white padding panel-counter", "position": "bottomLeft", "horizontal": "8", "vertical": "8"}, {"content": "Lorem ipsum dolor sit amet", "style": "opened black padding", "showTransition": "down", "hideTransition": "up", "horizontal": "20", "vertical": "20"} ] }, //... ] } }
7. Load content from XML.
$('#example').accordionSlider({ JSONSource: '/path/to/accordion.xml', });
<!-- accordion.xml --> <accordion> <panel> <background>path/to/1.jpg</background> <backgroundRetina>path/to/[email protected]</backgroundRetina> <backgroundOpened>path/to/1.jpg</backgroundOpened> <backgroundOpenedRetina>path/to/[email protected]</backgroundOpenedRetina> <backgroundLink>https://jqueryscript.net/</backgroundLink> <layer vertical="8" horizontal="8" position="bottomLeft" style="closed white vertical panel-counter">Panel 1 </layer> <layer vertical="10%" horizontal="40" style="opened black padding" hideTransition="left" showTransition="left">Lorem ipsum dolor sit amet </layer> <layer vertical="22%" horizontal="40" style="opened white padding" hideTransition="left" showTransition="left" hideDelay="200" showDelay="200">consectetur adipisicing elit </layer> <layer vertical="34%" horizontal="40" style="opened black padding" hideTransition="left" showTransition="left" hideDelay="500" showDelay="400" width="350">sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. </layer> </panel> ... </accordion>
8. It also supports Youtube/Vimeo/Sublime/HTML5 videos.
<!-- Youtube --> <iframe class="as-video" src="https://www.youtube.com/embed/VIDEO-ID?enablejsapi=1&wmode=opaque" width="500" height="350" frameborder="0" allowfullscreen></iframe> <!-- Vimeo --> <iframe class="as-video" src="https://player.vimeo.com/video/VIDEO-ID?api=1" width="500" height="350" frameborder="0" webkitallowfullscreen mozallowfullscreen allowfullscreen></iframe> <!-- HTML5 Video --> <video class="as-video" poster="path/to/poster.jpg" width="500" height="350" controls="controls" preload="none"> <source src="path/to/video.mp4" type="video/mp4"/> <source src="path/to/video.ogv" type="video/ogg"/> </video> <!-- Video.js --> <div class="as-video" data-videojs-id="video1"> <video id="video1" class="video-js vjs-default-skin" poster="path/to/poster.jpg" width="500" height="350" controls="controls" preload="none" data-setup="{}"> <source src="path/to/video.mp4" type="video/mp4"/> <source src="path/to/video.ogv" type="video/ogg"/> </video> </div> <!-- Sublime Video --> <video id="video2" class="as-video sublime" poster="path/to/poster.jpg" width="500" height="350" controls="controls" preload="none"> <source src="path/to/video.mp4" type="video/mp4"/> <source src="path/to/video.ogv" type="video/ogg"/> </video>
9. Full plugin options.
$('#example').accordionSlider({ // width/height width: 800, height: 400, // enable responsive layout responsive: true, // 'auto' or 'custom' responsiveMode: 'auto', // aspect ratio aspectRatio: -1, // 'horizontal' or 'vertical'. orientation: 'horizontal', // 0 for the first panel startPanel: -1, // the size of the opened panel // fixed or percentage value openedPanelSize: 'max', // max size of opened panel maxOpenedPanelSize: '80%', // 'hover', 'click', or 'never' openPanelOn: 'hover', // close the opened panels on mouse out closePanelsOnMouseOut: true, // the delay in milliseconds between the movement of the mouse pointer and the opening of the panel mouseDelay: 200, // the distance between consecutive panels panelDistance: 0, // duration on ms openPanelDuration: 700, closePanelDuration: 700, pageScrollDuration: 500, // easing function pageScrollEasing: 'swing', /* e.g. { 960: {visiblePanels: 5}, 800: {visiblePanels: 3, orientation: 'vertical', width: 600, height: 500}, 650: {visiblePanels: 4}, 500: {visiblePanels: 3, orientation: 'vertical', aspectRatio: 1.2} }/* breakpoints: null, // visible panels visiblePanels: -1, // 0 for the first page startPage: 0, // adds shadow to the accordion slider shadow: true, // determines if the panels will be shuffled/randomized shuffle: false, // determines if the panels will be overlapped panelOverlap: true, // autoplay options autoplay: true, autoplayDelay: 5000, autoplayDirection: 'normal', // 'normal' or 'backwards autoplayOnHover: 'pause', // 'pause', 'stop' or 'none' // keyboard options keyboard: true, keyboardOnlyOnFocus: false, keyboardTarget: 'panel', // 'panel' or 'page', // mousewheel options mouseWheel: true, mouseWheelSensitivity: 10, mouseWheelTarget: 'panel', // 'panel' or 'page' // swap background options swapBackgroundDuration: 700, fadeOutBackground: false, // touch swipe opitons touchSwipe: true, touchSwipeThreshold: 50, // 'playVideo' or 'none' openPanelVideoAction: 'playVideo', // 'pauseVideo' or 'stopVideo' closePanelVideoAction: 'pauseVideo', // 'stopAutoplay' or 'none' playVideoAction: 'stopAutoplay', // 'startAutoplay' or 'none' pauseVideoAction: 'none', // 'startAutoplay', 'nextPanel', 'replayVideo' or 'none' endVideoAction: 'none', // callback functions // or $('#example').on('callbackName', function(event) {}) init: function() {}, update: function() {}, accordionMouseOver: function() {}, accordionMouseOut: function() {}, panelClick: function(index) {}, panelMouseOver: function(index) {}, panelMouseOut: function(index) {}, panelOpen: function(index, previousIndex) {}, panelsClose: function(previousIndex) {}, pageScroll: function(index) {}, panelOpenComplete: function(index) {}, panelsCloseComplete: function(previousIndex) {}, pageScrollComplete: function(index) {}, breakpointReach: function(size, settings) {}, videoPlay: function() {}, videoPause: function() {}, videoEnd: function() {}, });
10. API methods.
// $('#example').accordionSlider('methodName', value); // or // var accordion = $('#example').data('accordionSlider'); // accordion.methodName(value); // Returns all the data of the panel at the specified index. accordion.getPanelAt(index); // Returns the index of the current panel. accordion.getCurrentIndex(); // Returns the total number of panels. accordion.getTotalPanels(); // Opens the next panel. accordion.nextPanel(); // Opens the previous panel. accordion.previousPanel(); // Opens the panel at the specified index. accordion.openPanel(index); // Closes all panels. accordion.closePanels(); // Gets the number of visible panels. accordion.getVisiblePanels(); // Gets the number of pages. accordion.getTotalPages(); // Gets the index of the page currently displayed. accordion.getCurrentPage(); // Scrolls to the specified page. accordion.gotoPage(index); // Goes to the next page. accordion.nextPage(); // Goes to the previous page. accordion.previousPage(); // Destroy accordion.destroy(); // Updates the accordion accordion.update(); // Removes all panels accordion.removePanels(); // resize the accordion slider accordion.resize();
10. Events.
// $('#example').on(eventType, callback); // $('#example').off(eventType); $('#example').on('init', function(event) { // do something }); $('#example').on('update', function(event) { // do something }); $('#example').on('accordionMouseOver', function(event) { // do something }); $('#example').on('accordionMouseOut', function(event) { // do something }); $('#example').on('panelClick', function(event, index) { // do something }); $('#example').on('panelMouseOver', function(event, index) { // do something }); $('#example').on('panelMouseOut', function(index) { // do something }); $('#example').on('panelOpen', function(event, index, previousIndex) { // do something }); $('#example').on('panelsClose', function(event, previousIndex) { // do something }); $('#example').on('pageScroll', function(event, index) { // do something }); $('#example').on('panelOpenComplete', function(event, index) { // do something }); $('#example').on('panelsCloseComplete', function(event, previousIndex) { // do something }); $('#example').on('pageScrollComplete', function(event, index) { // do something }); $('#example').on('breakpointReach', function(event, size, settings) { // do something }); $('#example').on('videoPlay', function(event) { // do something }); $('#example').on('videoPause', function(event) { // do something }); $('#example').on('videoEnd', function(event) { // do something });
Changelog:
2022-12-16
- revert code changes in touch swipe module
This awesome jQuery plugin is developed by bqworks. For more Advanced Usages, please check the demo page or visit the official website.