Easy Configurable BBCode Editor In jQuery
File Size: | 24.8 KB |
---|---|
Views Total: | 2407 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
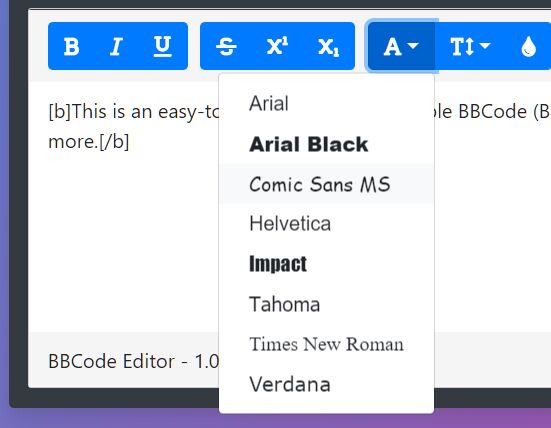
This is an easy-to-use yet fully configurable BBCode (Bulletin Board Code) editor for forums, message boards, contact & comment forms and much more.
More Features:
- Live preview.
- Allows to share the BBcode (base64 encoded) with others.
- Customize the buttons displayed in the toolbar.
- Convenient color picker.
- Image upload is supported as well.
- Multiple languages.
Dependendies:
- Bootstrap framework
- Input Mask plugin.
- An iconic font (Defaults to Font Awesome 5).
- pickr Color picker library.
How to use it:
1. Load the required JavaScript and CSS files in the document.
<!-- Bootstrap 4 Stylesheet --> <link rel="stylesheet" href="/path/to/cdn/bootstrap.min.css" /> <!-- Font Awesome 5 Iconic Font --> <link rel="stylesheet" href="/path/to/cdn/fontawesome/all.min.css" /> <!-- Pickr Stylesheet --> <link rel="stylesheet" href="/path/to/cdn/pickr/dist/themes/monolith.min.css" /> <!-- jQuery --> <script src="/path/to/cdn/jquery.min.js"></script> <!-- Bootstrap 4 JavaScript --> <script src="/path/to/cdn/popper.min.js"></script> <script src="/path/to/cdn/bootstrap.min.js"></script> <!-- Pickr JavaScript --> <script src="/path/to/cdn/pickr.es5.min.js"></script> <!-- Input Mask JavaScript --> <script src="/path/to/cdn/inputmask.min.js"></script>
2. Load the BBCodeditor's files in the document.
<!-- Stylesheet --> <link rel="stylesheet" href="./dist/jquery.editor.css"> <!-- You can find all languages under the lang folder --> <script src="./dist/lang/en_EN.js"></script> <!-- Or font-awesome-4.js --> <script src="./dist/icons/font-awesome-5.js"></script> <!-- Main JavaScript --> <script src="./dist/jquery.editor.js"></script>
3. Create a container element to hold the BBCode editor.
<div id="simple-bbcode-editor"></div> <!-- OPTIONAL --> <div id="simple-bbcode-editor-content"></div>
4. The JavaScript to generate a basic BBCode editor.
$(function() { $('#simple-bbcode-editor').bbcodeditor({ // This option must be included whenever the editor is called (it can be left empty) defaultValue: "", // This "option" is optional but you must call it if you want the get the value of the content area (editor) content_class: "simple-bbcode-editor-content" }); });
5. Add a Preview button to the BBCode editor.
$(function() { $('#simple-bbcode-editor').bbcodeditor({ defaultValue: "", content_class: "simple-bbcode-editor-content", includedButtons: [['bold', 'italic', 'underline'], ['strikethrough', 'supperscript', 'subscript'], ['font-name', 'font-size', 'color'], ['unordered-list', 'ordered-list', 'align'], ['link', 'image', 'media'], ['misc', 'advcode', 'table'],['preview']], customRequestToken: true, previewRequestTokenName: "_token", previewRequestToken: "vf5Un8KUDa", previewRequestUrl: "https://example.com/request_preview.php" }); });
6. Enable the image upload functionality on the BBCode editor.
$(function() { $('#simple-bbcode-editor').bbcodeditor({ defaultValue: "", content_class: "simple-bbcode-editor-content", enableImageUpload: true, uploadFileName: "filename", uploadFile: "bbcodeditor-image-upload", uploadFileTokenName: "_token", uploadFileToken: "vf5Un8KUDa" }); });
7. All default configuration options.
$(function() { $('#simple-bbcode-editor').bbcodeditor({ lang: 'en-EN', icons: 'font-awesome-5', height: 200, minHeight: 100, maxHeight: 400, button_class: 'btn btn-primary', content_class: '', includedButtons: [ ['bold', 'italic', 'underline'], ['strikethrough', 'supperscript', 'subscript'], ['font-name', 'font-size', 'color'], ['unordered-list', 'ordered-list', 'align'], ['link', 'image', 'media'], ['misc', 'advcode', 'table'] ], advcodeLanguages: ['General Code', 'HTML', 'CSS', 'Javascript', 'PHP', 'XML', 'JSON', 'SQL', 'Ruby', 'Python', 'Java', 'C', 'C#', 'C++', 'Lua', 'Markdown', 'Yaml'], enableTextareaResize: true, colorPickerDefaultColor: '#3498DB', colorPickerSwatches: [ 'rgba(52, 152, 219, 1)', 'rgba(46, 204, 113, 1)', 'rgba(26, 188, 156, 1)', 'rgba(234, 76, 136, 1)', 'rgba(155, 89, 182, 1)', 'rgba(241, 196, 15, 1)', 'rgba(243, 156, 18, 1)', 'rgba(231, 76, 60, 1)', 'rgba(236, 240, 241, 1)', 'rgba(189, 195, 199, 1)', 'rgba(149, 165, 166, 1)', 'rgba(127, 140, 141, 1)', 'rgba(52, 73, 94, 1)', 'rgba(44, 62, 80, 1)' ], enableImageUpload: false, imageUploadUrl: "", imageUploadType: "POST", uploadFileName: "filename", uploadFile: "bbcodeditor-image-upload", uploadFileTokenName: "_token", uploadFileToken: "", includedMiscItems: ['h1', 'h2', 'h3', 'h4', 'h5', 'h6', 'blockquote', 'code', 'linebreak'], previewRequestUrl: "", previewRequestType: "GET", customRequestToken: false, previewRequestTokenName: "_token", previewRequestToken: "" }); });
7. Get the content of the BBCode editor.
$('#simple-bbcode-editor').val();
Changelog:
2020-03-19
- Update fr_FR.js
2020-01-16
- Update jquery.editor.js
This awesome jQuery plugin is developed by MrAnonymusz. For more Advanced Usages, please check the demo page or visit the official website.