Easy JavaScript/jQuery Input Mask Plugin - inputmask
File Size: | 971 KB |
---|---|
Views Total: | 214007 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
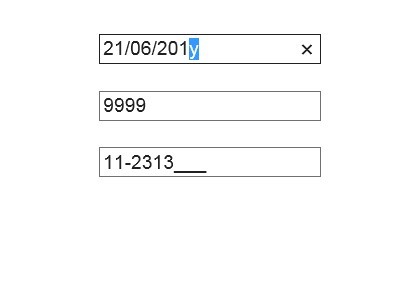
jQuery Input Mask is a lightweight and easy-to-use JavaScript/jQuery plugin that makes it easier to create an input mask. An input mask helps the user with the input by ensuring a predefined format. This can be useful for dates, numerics, phone numbers, etc.
Features:
- optional parts anywere in the mask
- possibility to define aliases which hide complexity
- date / datetime masks
- numeric masks
- lots of callbacks
- non-greedy masks
- many features can be enabled/disabled/configured by options
- supports readonly/disabled/dir="rtl" attributes
- support data-inputmask attribute
- multi-mask support
- can be used as a vanilla JavaScript plugin
See Also:
- Easy Number Formatting Plugin with jQuery - number
- Input Field Data Formatting Plugin - Mask
- Price Format jQuery Plugin - Price Format
- Easy Numbers and Currency Formatting Plugin - autoNumeric
- Automatic Input Field Data Formatting Plugin - jQuery mask
- jQuery Currency Input Filed Mask Plugin - maskmoney
- 10 Best Input Mask Plugins In JavaScript
How to use it:
1. Install and import the Input Mask plugin.
# NPM $ npm install inputmask --save
// ES6 module import Inputmask from "inputmask";
<!-- As A Vanilla JS Plugin --> <script src="/path/to/dist/inputmask.min.js"></script> <!-- As A jQuery Plugin --> <script src="/path/to/cdn/jquery.min.js"></script> <script src="/path/to/dist/jquery.inputmask.min.js"></script> <!-- Allows data-input attribute --> <script src="/path/to/dist/bindings/inputmask.binding.js"></script>
2. Define your masks using data-inputmask
attribute. The plugin supports any text fields and event content editable element.
<input data-inputmask="'alias': 'date'" /> <div contenteditable="true" data-inputmask="'mask': '9', 'repeat': 10, 'greedy' : false"></div> <textarea data-inputmask="'mask': '99-9999999'" /></textarea>
3. Default masking definitions.
- 9: numeric
- a: alphabetical
- *: alphanumeric
4. Predefined mask alias.
- datetime
- numeric
- cssunit
- url
- IP
- mac
- vin
5. Call the plugin on the target element and done.
// Vanilla JavaScript Inputmask().mask(document.querySelectorAll("Selector")); // jQuery $("Selector").inputmask();
6. You can also define the input mask in the JavaScript during init.
// Vanilla JavaScript Inputmask("9-a{1,3}9{1,3}").mask("Selector"); // jQuery $("Selector").inputmask("99-9999999");
7. All default options to customize the input mask plugin.
Inputmask({ // mask placeholder placeholder: "_", // symbols used to indicate an optional part in the mask optionalmarker: [ "[", "]" ], // symbols used to indicate a quantifier in the mask quantifiermarker: [ "{", "}" ], // symbols used to indicate a group in the mask groupmarker: [ "(", ")" ], // symbols used to indicate an alternator part in the mask alternatormarker: "|", // symbols used to escape a part in the mask escapeChar: "\\", // mask here mask: null, // define your mask using regex regex: null, // executes when the mask is complete oncomplete: $.noop, // executes when the mask is incomplete and focus is lost onincomplete: $.noop, // executes when the mask is cleared oncleared: $.noop, // repetitions of the mask: * ~ forever, // otherwise specify an integer repeat: 0, // true: allocated buffer for the mask and repetitions // false: allocate only if needed greedy: true, // automatically unmask when retrieving the value with $.fn.val or value if the browser supports __lookupGetter__ or getOwnPropertyDescriptor autoUnmask: false, // remove the mask before submitting the form. removeMaskOnSubmit: false, // remove the empty mask on blur or when not empty removes the optional trailing part clearMaskOnLostFocus: true, // insert the input or overwrite the input insertMode: true, // clear the incomplete input on blur clearIncomplete: false, // extend the masks alias: null, // callback to implement autocomplete on certain keys for example. // args => event, buffer, caretPos, opts onKeyDown: $.noop, // executes before masking the initial value to allow preprocessing of the initial value. // args => initialValue, opts => return processedValue onBeforeMask: undefined, // executes before masking the pasted value to allow preprocessing of the pasted value. // args => pastedValue, opts => return processedValue onBeforePaste: undefined, // executes before writing to the masked element. // args => event, opts onBeforeWrite: undefined, // executes after unmasking to allow postprocessing of the unmaskedvalue. // args => maskedValue, unmaskedValue, opts onUnMask: undefined, // show the mask-placeholder when the input has focus showMaskOnFocus: true, // show the mask-placeholder when hovering the empty input showMaskOnHover: true, // executes on every key-press with the result of isValid. // Params: result, opts onKeyValidation: $.noop, // a character which can be used to skip an optional part of a mask skipOptionalPartCharacter: " ", // align to the right rightAlign: false, // pressing escape reverts the value to the value before focus undoOnEscape: true, // numeric basic properties radixPoint: "", //".", // | "," // numeric basic properties groupSeparator: "", // try to keep the mask static while typing. // Decisions to alter the mask will be posponed if possible // undefined see auto selection for multi masks keepStatic: undefined, // when enabled the caret position is set after the latest valid position on TAB positionCaretOnTab: true, // allows for tabbing through the different parts of the masked field tabThrough: false, // list with the supported input types supportsInputType: ["text", "tel", "password"], // specify keyCodes which should not be considered in the keypress event, otherwise the preventDefault will stop their default behavior especially in FF ignorables: [8, 9, 13, 19, 27, 33, 34, 35, 36, 37, 38, 39, 40, 45, 46, 93, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 0, 229], // override for isComplete - args => buffer, opts - return true || false isComplete: null, //hook to alter the clear behavior in the stripValidPositions args => maskset, position, lastValidPosition, opts => return true|false/command object canClearPosition: $.noop, // hook to preValidate the input. Usefull for validating regardless the definition. args => buffer, pos, char, isSelection, opts => return true/false/command object preValidation: null, // hook to postValidate the result from isValid. Usefull for validating the entry as a whole. args => buffer, currentResult, opts => return true/false/json postValidation: null, // specify a definitionSymbol for static content, used to make matches for alternators staticDefinitionSymbol: undefined, // just in time masking ~ only mask while typing, can n (number), true or false jitMasking: false, // return nothing instead of the buffertemplate when the user hasn't entered anything. nullable: true, // dev option - testing inputfallback behavior inputEventOnly: false, // disable value property patching noValuePatching: false, // none, lvp (based on the last valid position (default), radixFocus (position caret to radixpoint on initial click) positionCaretOnClick: "lvp", // mask-level casing. Options: null, "upper", "lower" or "title" casing: null, // specify the inputmode - already in place for when browsers will support it inputmode: "verbatim", // use the data-inputmask attributes or to ignore them. importDataAttributes: true, // alter the behavior of the char shifting on entry or deletion shiftPositions: true, // use prototype definitions usePrototypeDefinitions: true, // in milliseconds validationEventTimeOut: 3000, })
8. You can also pass the options via data-inputmask-OPTION
attributes
<input data-inputmask="'mask': '9', 'repeat': 10, 'greedy' : false" /> <input data-inputmask-clearmaskonlostfocus="false" /> <input data-inputmask="'alias': 'datetime'" />
9. API methods.
// Get the unmasked value // Vanilla JavaScript var input = document.getElementById(selector); input.inputmask.unmaskedvalue(); // jQuery $(selector).inputmask('unmaskedvalue'); // Get the default mask value // Vanilla JavaScript var input = document.getElementById(selector); input.inputmask.getemptymask(); // jQuery $(selector).inputmask("getemptymask"); // Check if the returned value is masked // Vanilla JavaScript var input = document.getElementById(selector); if (input.inputmask.hasMaskedValue()) // jQuery if ($(Selector).inputmask("hasMaskedValue")); // Check whether the current value is complete or not // Vanilla JavaScript var input = document.getElementById(selector); if (input.inputmask.isComplete()) // jQuery if ($(Selector).inputmask("isComplete")); // Check if is valid // Vanilla JavaScript var input = document.getElementById(selector); if (input.inputmask.isValid()) // jQuery if ($(Selector).inputmask("isValid")); // Get the metadata of the actual mask // Vanilla JavaScript var input = document.getElementById(selector); input.inputmask.getmetadata(); // jQuery $(Selector).inputmask("getmetadatae")); // Set new values // Vanilla JavaScript var input = document.getElementById(selector); input.inputmask.setValue(Value); // jQuery $(Selector).inputmask("setValue", Value)); // Update options // Vanilla JavaScript document.querySelector(Selector).inputmask.option({ onBeforePaste: function (pastedValue, opts) { return phoneNumOnPaste(pastedValue, opts); } }); // jQuery $("Selector").inputmask("option", { onBeforePaste: function (pastedValue, opts) { return phoneNumOnPaste(pastedValue, opts); } }) // Format given values // Set new values // Vanilla JavaScript Inputmask.format("2331973", { alias: "datetime", inputFormat: "dd/mm/yyyy"}); // jQuery $(Selector).inputmask("format", "2331973"), { alias: "datetime", inputFormat: "dd/mm/yyyy"}); // Remove the inputmask var input = document.getElementById(selector); input.inputmask.remove(); // jQuery $(selector).inputmask("remove");
Changelog:
v5.0.9beta66 (2024-04-21)
- Update & Bugfix
v5.0.8beta49 (2022-09-27)
- Added validationEventTimeOut option
- User Webpack/Terserplugin for minification
- Fix caret shift with negative numbers in numeric aliases
- enhance alternation logic
- update datetime alias
- datetime prefillYear option
- Enable/disable prefilling of the year.
- Although you can just over type the proposed value without deleting, many seems to see a problem with the year prediction.
- This options is to disable this feature.
- fix japanese input ime
- Lots of bugs fixed.
v5.0.6 (2020-09-16)
- bugs fixed
v5.0.5 (2020-07-10)
- remove some fn in dependencylib
v5.0.4 (2020-03-04)
- Add: SetMaxOnOverflow option (numeric alias).
- Update: datetime alias: add autofill logic to year, complete with current year, allow filled year be overtyped when min date is provided.
- Bugs fixed.
v5.0.0 (2020-02-13)
- Add indian numbering support in numeric alias (indianns alias)
- Add roundingFN option to numeric alias. (currency, decimal, ...)
- input-inputmode support via inputmode option (if supported by browser)
- Add shortcuts option in numeric alias.
- Add insertModeVisual option
- Bugs fixed.
- Features updated.
2019-10-25
- Demo & Doc updated
v4.0.4 (2019-10-22)
- add url as supported input type
- rework jit enabled quantifiers
- restore greedy functionality
- fix focus and mouseenter behavior in IE
v3.3.7 (2017-06-09)
- Added: allow custom operation in casing option by callback
- Updated: put back Regex alias extension for legacy support #1611
- Updated: postvalidation cannot set pos of undefined
- Updated: fix undoValue initialization
- Fixed: Major issue with regex
- Fixed: React onChange event doesn't work with Inputmask
- Fixed: Currency digits and delete
- Fixed: Decimal editing problems
- Fixed: UX problem with email mask
- Fixed: Force numeric to empty (on blur) with '0' as value
- Fixed: ndxInitializer.shift is not a function
v3.3.6 (2017-04-20)
- Added: noValuePatching option
- Updates: alternator syntax update - regex like alternations is now supported (aa|99|AA) ~ aa or 99 or AA
- Fixed: integer backspace bug when set maxLength attr.
- Fixed: Regex with placeholder, not working?
- Fixed: Visualize regular expressions #1040
- Fixed: Mobile phone code update needed for Malaysia
- Fixed: suffix bug (regression)
- Fixed: 29 february of non leap-year
v3.3.2 (2016-09-03)
- Added 'casing': 'title'
- performance updates
- replaced radixFocus option by positionCaretOnClick. Allows choice for behavior of the caret on click. (none, lvp (default), radixFocus)
- removed nojumps option
- update phone alias implementation
- enhanced alternation selection
- change default of positionCaretOnTab to true
- Fixed: onUnMask is not being called
- Fixed 'setvalue' on mask with a suffix results in suffix being doubled, while $.fn.val works fine
- Fixed when typing after a fixed character
- fixup definitions for phone alias
- Fixed: Hide mask's items that have multiple options
- fix currency with " " as groupseparator
- Fixed: change addEventListener not fired in IE11
- Dynamically changing mask based on number of entered characters
- Fixed: Input event doesn't fire in IE
- Fixed: Unable to get property 'forwardPosition' of undefined or null reference
- Fixed Problems with greedy dynamic masks
- IE11 fix
- Enhance caret positioning on click
- Pasting to masked input not working on Android
- update inputfallback + checkval
v3.3.1 (2016-04-20)
- Fixed Safari Error: RangeError: Maximum call stack size exceeded
- Fixed Safari Maximum call stack size exceeded when inputmask bound twice
- Fixed Email alias @ => @._
- Fixed Safari date mask - Context switch when jquery.valhook fallback is used
- adding extra options though option method => auto apply the mask + add noremask option
- speedup insert and delete from characters
- better handle alternator logic by merging the locators
- patchValueProperty - enable native value property patch on IE8/IE9
v3.3.0 (2016-04-05)
- Fixed Email mask not accepting valid emails
- Fixed Deleting character from input with 'email' alias shifts all data
- Fixed some events like paste & cut for Vanilla dependencyLib
- Fixed AutoUnmask not working on IE11
- Fixed Email mask incorrectly including underscore => allowed as not typed => result invalid
- Fixed Can not clear value when select all and press BACKSPACE in some circumstance
- Fixed error occurs in safari 9.0.3
- Fixed Paste does not work properly when using numericInput
- Fixed "[object Object]" value after $element.inputmask('setvalue', '') call
- Fixed: Lost zero while replacing a digit in group
- Fixed: RadixFocus problem
- Fixed: "0.00" not displayed if "clearMaskOnLostFocus: true"
- Fixed: Can not change integer part when it is "0"
- Fixed NumericInput option can't handle 100.00
- Fixed: IE8 error: Object doesn't support this property or method
- Fixed: numeric alias produces "0.00" instead of null when cleared out.
- Fixed: Caret Positioned After Last Decimal Digit Disallows Sign Input When digits Option Set
- Fixed: Clicking on a highlighted masked field does not set the caret to the first valid position (Chrome)
- Fixed: 'alias': 'numeric', zero value
- Fixed: Distinguish empty value and '$ 0.00' value for currency alias
- Fixed: validate regular expression for indian vehicle registration number
- Fixed: InputMask remove a 0 in left side. (numericInput: true)
- Fixed: min value issue
- Fixed: PostValidation function fails when using placeholder and digitsOptional is false
- improve getmetadata
- Added VIN mask
- Added nullable option => switch to return the placeholder or null when nothing is entered
- change funtionality of postValidation => result may be true|false
- patchValueProperty - enable native value property patch on IE10/IE11
- update negation handling for numeric alias
v3.2.7 (2016-01-27)
- IEMobile fixes
- favor inputfallback for android
- Fixed: Issue in Android (Samsung GALAXY S5)
- Fixed: time mask, backspace behavior on android chrome
- Fixed: Android Chrome Browser
- Fixed: Mask issue in Android with Swype Keyboard
- Fixed: Pasting to masked input not working on Android
- Fixed: Decimal point/comma not working on Android 4.4
- Fixed: Doesn't work on Android #1073
- Fixed: numeric input in mobile #897
- Fixed: Support for Android default browser #368
- Fixed: Repeating a character and a number On Mobile
- Fixed: Inputs are ignored on FF 39 on Android 5.0.2
- Fixed: Phone input mask duplicates each character on Samsung Android tablet
- Fixed: Support for Android default browser
- Fixed: fixed "valids is not defined" error
v3.2.6 (2016-01-25)
- Added staticDefinitionSymbol
- Added include textarea as a valid masking element
- add jitMasking option
- add supportsInputType option
- update alternation logic
- implement missing parts in the jqlite DependencyLib
- Remove namespaces for events (simplifies implementing other dependencyLibs, besides jquery)
- Fixed No-strict mask
- Fixed Inputmask not work with textarea
- Fixed Cursor shifted to the RIGHT align any way
- Fixed Multiple masks
- Fixed Double enter for submit
- Fixed Multiple Mask Click Focus Error
- Fixed IE9 SCRIPT445: Object does not support this action
- Fixed Input mask can't be applied on other HTML5 input types
- Fixed Initial value like VAA gets truncated to V-__ with mask like "I{1,3}-ZZ"
- Fixed Show placeholder as user types
- Fixed IE11 clear not working in emulated IE9 mode
- Fixed Issue with reset of inputmask field
- Fixed '405 not allowed' error on loading phone-codes.js on certain Ajax configuration.
- enhanced caching in gettests
- Fixed Windows Phone User unable to set Date
v3.2.5 (2015-11-24)
- Fixed numeric extensions don't supported with vanilla DependencyLib
- Fixed alias options from 'data-inputmask' is not used anymore
- Fixed Email mask doesn't allow to go to the domain part by mouse
- Fixed Currency validator gives false negative if number of digits in integer part is not multiplier of groupSize
- Fixed data-inputmask => mask with optionals not parsed correctly
- improve cursor positioning and placeholder handling
- remove $("selector").inputmask("mask", { mask: "99-999 ..." }) format from plugin
v3.2.4 (2015-11-20)
- allow passing a selector to the mask function
- allow passing an element id to the mask function
- Fixed: bower package
- Fixed: autoUnmask not work in newest release
- Fixed: Definition {_} throws an exception #1106 => update readme
- Fixed: Uncaught TypeError for "percentage" alias
- Fixed: Wrong behavior for symbol delete in ip alias
- Fixed: element validation for the vanilla dependencyLib
v3.2.3 (2015-11-09)
- Add tooltip option
- Improve handling of compositionevents
- Improve extendAliases, extendDefinitions, extendDefaults
- inputmask.binding => automated inputmask binding for html attributes
- optimize init
- Fixed extra tooltip property
- Fixed Numeric aliases insert '0' in input after clearing if there was fraction part
- Fixed Clear optional tail in getvalue.
- Fixed Each character repeats on Mobile
- Fixed errors in IE8
- Fixed upper/lower case handling in data-inputmask-*
- Fixed Double Change action when pressing Enter in Firefox
- Fixed CTRL-x / Cut issue
- Fixed Cannot erase input value throw mask symbols (Android 4.4, Android 4.2)
- Fixed escaperegex call
v3.2.2 (2015-10-14)
- Fixed Missing comma in bower.json and component.json
v3.2.1 (2015-09-21)
- fixed jquery.inputmask.bundle.js
- fixed dependency paths for browserify
- fixed DependencyLib error in Internet Explorer
- fixed Dynamically switching mask in same input box not functioning as expected
- fixed Invalid JSON phone-uk.js
- fixed field input width characters cropped while writing
- fixed Currency with autogroup and no digits not working
- update files to be included for package.json, bower.json, component.json
- update namespace dependencyLib => inputmask.dependencyLib
- added inputmask.dependencyLib.jquery
- added inputmask.dependencyLib.jqlite
v3.2.0 (2015-09-04)
- Fixed: never trigger 'input' event when paste after invoke inputmask
- Fixed: Script looping start when add '.' between decimal values ('.' part)
- Fixed: Dynamic masks with {*} and zero repeats
- Fixed: Mask does not alternate back after deleting digit
- Fixed: Script looping start when add '.' between decimal values
- Fixed: Delete key not working properly
- Fixed: Chinese / Japanese characters are unable to mask
- Fixed: Infinite Loop on IE (v11) when using Japanese IME Keyboard
- Fixed: Numeric inputMask doesn't rounds value
- Fixed: Decimal looses digits
- Fixed: Firefox: cursor jumps to the right when clicking anywhere on the value
- Fixed: numeric carret position broken
- Fixed: Escape optional marker, quantifiable marker, alternator marker and backslash not working
- Fixed: Escape value is inconsistent after mask
- Fixed: Add isValueMask option to support masking value that not yet mask
- Fixed: updating a value on onincomplete event doesn't work
- Fixed: Provide convenient method to unmask value
- Fixed: phone-codes.js is missing when installing with bower
- Fixed: Typing 1000 becomes 1.00 using groupSeparator="."
- Fixed: Entering a period on a blank 'numeric' alias input not allowed
- Fixed: Decimal separator conversion
- Fixed: Auto position cursor at end of data on focus
- Fixed: Make numericInput work with complex masks
- Fixed: Regression in currency
- Fixed: On google chrome, cannot use jquery to clone the inputmask control with data and events
- Fixed: Cannot overwrite characters when highlighting the characters to the right of the decimal
- Fixed: Decimal mask accepts "123,456." (RadixPoint with no number after it)
- Fixed: Default Enter key function getting lost on an input mask text field
- Fixed: Numeric inputs with default value are setted to blank when submit the form
- Fixed: Focus loop on IE9 with numeric.extensions
- Fixed: Regression in currency alias
- Fixed: Set specific inputmask option on already initialized control
- Fixed: Issue using datamask-input attributes and event handlers
- some fixes for numeric alias - stripValidPositions
- Update placeholder handling
- separate jquery plugin code from the inputmask core (first step to remove jquery dependency from the inputmask core)
- Update placeholder handling
- remove "jquery." in the naming of the extensions to better reflect their denpendency
- remove $.inputmask in favor of inputmask class
- make auto unmask to support escape character in the middle.
- Added: inputmask class
- Added: setting defaults / definitions / aliases
- Added: numeric alias - increment/decrement by ctrl-up/ctrl-down
- Added: percentage alias
- Added: numeric alias - round values
- Added: inputmask.unmask
This awesome jQuery plugin is developed by RobinHerbots. For more Advanced Usages, please check the demo page or visit the official website.